Maximizing Arquillian for Jakarta EE TCKs
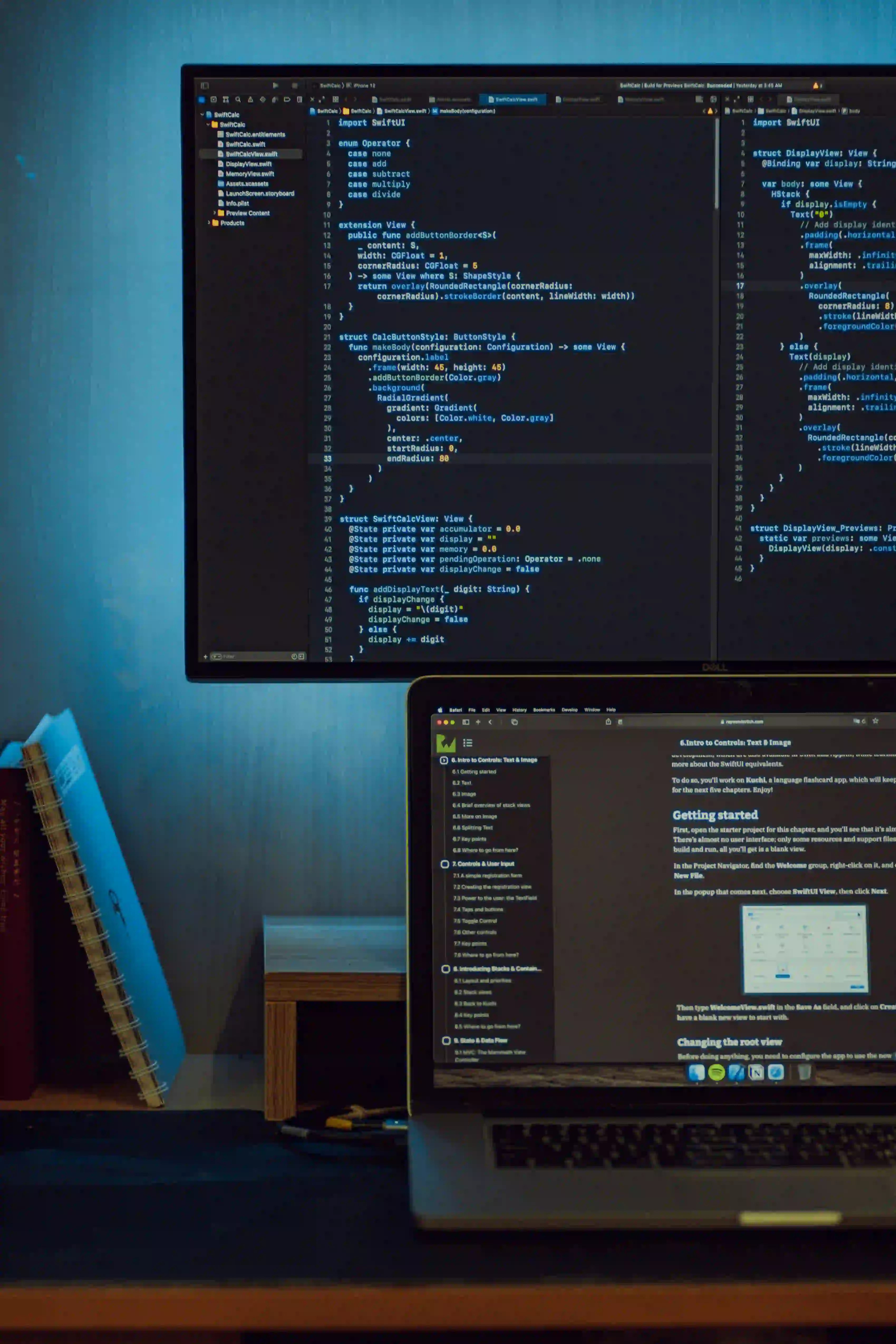
Maximizing Arquillian for Jakarta EE TCKs
As a Java developer, you understand the significance of testing your Jakarta EE applications thoroughly to ensure they adhere to established standards and specifications. This is where the Jakarta EE Technology Compatibility Kits (TCKs) play a pivotal role. TCKs validate that your codebase aligns with the Jakarta EE specifications, ensuring compatibility across different implementations.
In this blog post, we’ll delve into the realm of maximizing Arquillian for Jakarta EE TCKs. Arquillian serves as a powerful testing platform, allowing you to seamlessly execute TCKs against your Jakarta EE applications. Let’s explore how to harness the full potential of Arquillian to conduct comprehensive TCK testing.
Understanding Arquillian
Arquillian is a sophisticated testing platform that simplifies the process of integration testing for Java applications. It seamlessly integrates with Jakarta EE containers, enabling you to test your applications in a real environment without the need for complex setup or management.
Arquillian acts as a bridge between your test code and the Jakarta EE container, facilitating the deployment of your application and executing test cases within the container itself. This empowers you to perform a wide array of tests, including TCK testing, in a more realistic and comprehensive manner.
Integrating Arquillian and Jakarta EE TCKs
To harness the full potential of Arquillian for TCK testing, you need to integrate the TCKs into your testing environment. Here’s how you can achieve this seamlessly:
Adding Jakarta EE TCK Dependencies
Firstly, you need to ensure that the Jakarta EE TCK dependencies are included in your project. This entails adding the relevant TCK artifacts to your project’s build configuration. For example, using Maven, you can include the TCK dependencies within the <dependencies>
section of your pom.xml
:
<dependencies>
<dependency>
<groupId>org.eclipse</groupId>
<artifactId>jakartaee-platform</artifactId>
<version>8.0</version>
<type>pom</type>
</dependency>
<!-- Include other TCK dependencies here -->
</dependencies>
By including the TCK dependencies, you ensure that your project has access to the required Jakarta EE TCK artifacts for conducting comprehensive testing.
Setting Up Arquillian Configuration
Next, you need to configure Arquillian to orchestrate the TCK testing process. This involves defining the necessary deployment and test execution configurations within your project.
An example arquillian.xml
configuration file could look like this:
<?xml version="1.0" encoding="UTF-8"?>
<arquillian xmlns="http://jboss.org/schema/arquillian"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://jboss.org/schema/arquillian
http://jboss.org/schema/arquillian/arquillian_1_0.xsd">
<container qualifier="jboss" default="true">
<configuration>
<property name="jbossHome">path/to/your/jakarta-ee-container</property>
</configuration>
</container>
</arquillian>
In this configuration, you specify the container qualifier and its configuration, such as the location of your Jakarta EE container. This allows Arquillian to seamlessly deploy your application and execute tests within the specified container environment.
Writing TCK Test Cases
With the TCK dependencies and Arquillian configuration in place, you can proceed to write your TCK test cases. These test cases leverage the Arquillian framework to execute the TCK tests within the Jakarta EE container.
Consider the following example of a simple TCK test case using Arquillian:
@RunWith(Arquillian.class)
public class JakartaEETCKTest {
@Deployment
public static JavaArchive createDeployment() {
return ShrinkWrap.create(JavaArchive.class)
.addClass(MyJakartaEEApplication.class)
.addAsManifestResource(EmptyAsset.INSTANCE, "beans.xml");
}
@Test
public void testSomething() {
// Perform TCK test assertions here
}
}
In this test case, the @RunWith(Arquillian.class)
annotation indicates that the test will be executed within the Arquillian framework. The @Deployment
method defines the deployment configuration for the test, specifying the necessary application artifacts to be deployed within the Jakarta EE container. Finally, the @Test
method encapsulates the TCK test assertions, allowing you to verify the compliance of your Jakarta EE application with the specifications.
Maximizing TCK Testing with Arquillian Extensions
Beyond the fundamental integration of Arquillian with Jakarta EE TCKs, you can further maximize your TCK testing by leveraging Arquillian extensions. These extensions provide additional capabilities and features to enhance your testing process.
Arquillian Chameleon
Arquillian Chameleon is an extension that simplifies the management of container configurations, allowing you to effortlessly switch between different container versions and configurations. This is particularly useful when conducting TCK tests across multiple Jakarta EE container implementations.
Integrating Arquillian Chameleon into your testing environment enables you to dynamically adjust the container configurations, ensuring comprehensive TCK testing across various Jakarta EE environments.
Arquillian Cube
Arquillian Cube facilitates the integration of container orchestration tools, such as Docker and Kubernetes, into your testing workflow. By incorporating Arquillian Cube, you can extend your TCK testing to encompass containerized environments, enabling more extensive validation of your Jakarta EE application’s compatibility.
To Wrap Things Up
Maximizing Arquillian for Jakarta EE TCKs is pivotal to ensuring the robustness and compliance of your Jakarta EE applications. By seamlessly integrating Arquillian with Jakarta EE TCKs, configuring the necessary dependencies, and leveraging Arquillian extensions, you can elevate the quality of your TCK testing process.
Arquillian serves as a powerful ally in your quest for Jakarta EE compatibility, empowering you to conduct comprehensive TCK testing with ease and efficiency. Embrace the potential of Arquillian to fortify the reliability and adherence of your Jakarta EE applications to industry standards and specifications.
In conclusion, by honing your skills in Arquillian and TCK testing, you can pave the way for Jakarta EE applications that not only meet but exceed the established standards, solidifying their position in the realm of enterprise-grade Java development.
So, why wait? Start maximizing Arquillian for Jakarta EE TCKs and elevate your testing game to new heights of excellence!