Dealing with Inconsistent Transparency in JavaFX
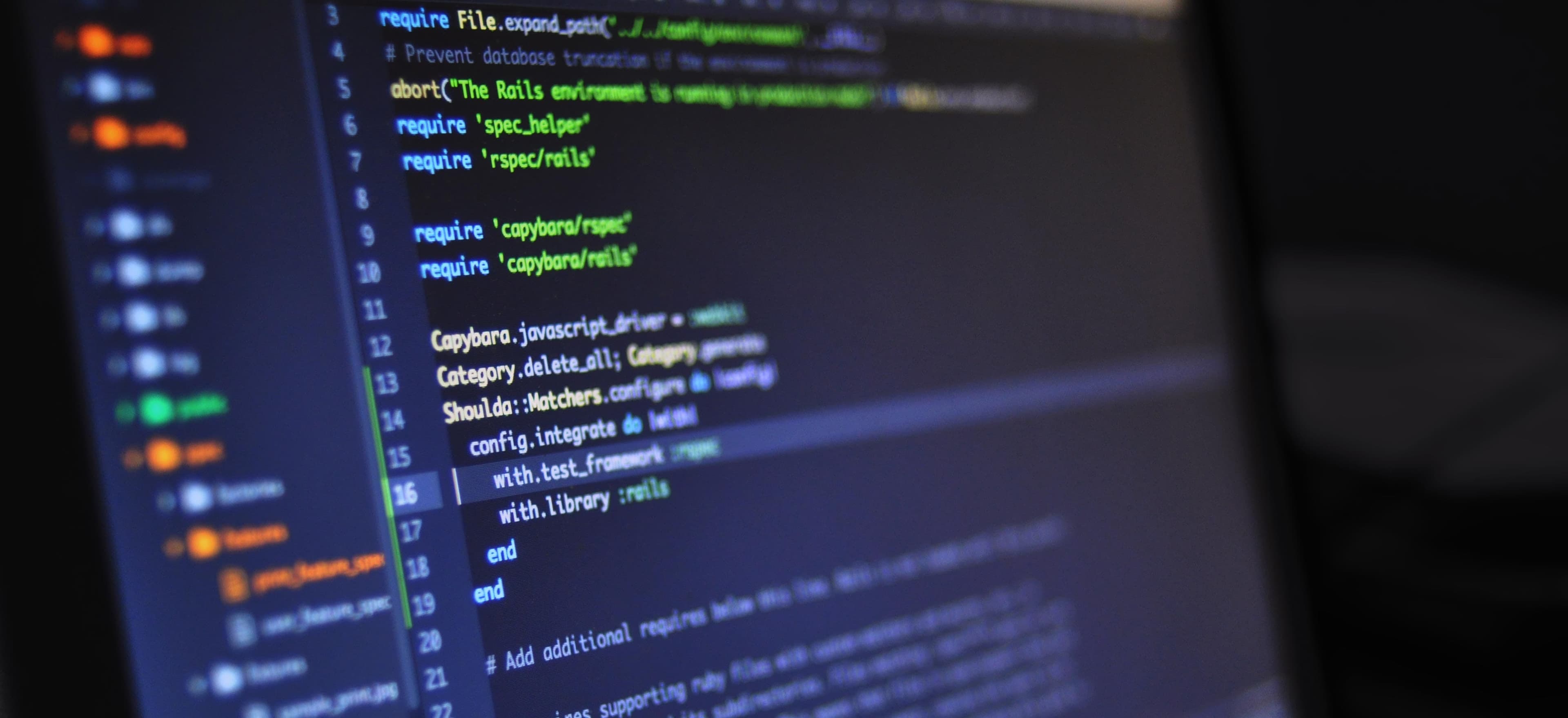
- Published on
Dealing with Inconsistent Transparency in JavaFX
When developing user interfaces in Java, ensuring consistent and predictable behavior of graphical elements is crucial. However, dealing with transparency in JavaFX can sometimes lead to unexpected results. This blog post will explore the potential pitfalls of handling transparency in JavaFX and present best practices and solutions to achieve consistent and expected behavior.
Understanding the Issue
Transparency in JavaFX is typically achieved through the use of alpha channels in colors and opacity settings in nodes. While the JavaFX framework provides powerful tools for creating visually appealing interfaces, developers often encounter issues with inconsistent transparency rendering. This inconsistency can manifest when overlaying transparent nodes, applying effects, or working with different blending modes.
Alpha Compositing
Alpha compositing is the process of combining a semi-transparent foreground color with a background color to produce a composite color. The alpha value represents the opacity of the color, with 0 being fully transparent and 1 being fully opaque. When two transparent elements overlap, the alpha compositing algorithm determines the resulting visual appearance. Understanding how alpha compositing works is essential for resolving transparency issues in JavaFX.
Pitfalls and Solutions
Overlapping Transparent Nodes
When transparent nodes overlap in JavaFX, the resulting visual appearance may not align with expectations. This is due to the alpha compositing process, which considers the alpha values of the overlapping nodes to calculate the final color. To address this issue, it's crucial to understand the blend modes available in JavaFX.
Example: Using Blend Modes
// Creating two overlapping rectangles with transparency
Rectangle rect1 = new Rectangle(100, 100, Color.rgb(255, 0, 0, 0.5));
Rectangle rect2 = new Rectangle(50, 50, Color.rgb(0, 0, 255, 0.5));
// Applying different blend modes to the second rectangle
rect2.setBlendMode(BlendMode.DARKEN);
// Adding the rectangles to a common parent node
Group group = new Group(rect1, rect2);
In this example, the BlendMode.DARKEN
is applied to the second rectangle, altering how its transparency interacts with the underlying rectangle. By experimenting with different blend modes, developers can achieve the desired visual composition when dealing with overlapping transparent nodes.
Effects and Transparency
Applying effects, such as drop shadows or blurs, to transparent nodes can lead to unexpected visual artifacts. This is particularly evident when the effect is applied to a semi-transparent node, as the interaction between the effect and transparency may not produce the intended result.
Example: Applying Drop Shadow to Transparent Node
// Creating a transparent rectangle
Rectangle transparentRect = new Rectangle(200, 200, Color.rgb(0, 255, 0, 0.5));
// Applying a drop shadow effect to the transparent rectangle
DropShadow dropShadow = new DropShadow(10, Color.BLACK);
transparentRect.setEffect(dropShadow);
In this example, applying a drop shadow to a semi-transparent rectangle can result in unexpected visual artifacts, such as the shadow becoming more pronounced in areas with higher transparency. To mitigate this issue, developers can adjust the blending and rendering order of nodes to achieve the desired visual outcome.
Rendering Order and Transparency
The order in which transparent nodes are rendered can significantly impact the final visual appearance. When dealing with overlapping transparent elements, the rendering order determines how the alpha compositing algorithm blends the colors, affecting the overall transparency outcome.
Example: Setting Rendering Order
// Creating two overlapping circles with transparency
Circle circle1 = new Circle(100, 100, 50, Color.rgb(255, 0, 0, 0.5));
Circle circle2 = new Circle(150, 150, 50, Color.rgb(0, 0, 255, 0.5));
// Adjusting rendering order to control transparency blending
group.getChildren().addAll(circle2, circle1); // Rendering circle2 on top of circle1
In this example, adjusting the rendering order of the circles within the parent node can influence how their transparency is composited. By carefully managing the rendering order, developers can ensure the expected visual hierarchy and transparency blending.
Best Practices
To achieve consistent and expected transparency rendering in JavaFX, developers should consider the following best practices:
- Use Blend Modes: Experiment with different blend modes to control how transparency interacts between overlapping nodes.
- Be Mindful of Effects: When applying effects to transparent nodes, consider how the effect interacts with the node's transparency and adjust as needed.
- Manage Rendering Order: Control the rendering order of transparent nodes to influence the alpha compositing process and achieve the desired visual outcome.
By adhering to these best practices, developers can overcome the challenges of inconsistent transparency rendering in JavaFX and create visually compelling user interfaces with predictable transparency behavior.
My Closing Thoughts on the Matter
Transparency management in JavaFX requires a nuanced understanding of alpha compositing, blend modes, rendering order, and the interaction of effects with transparency. By recognizing the potential pitfalls and applying best practices, developers can achieve the desired visual consistency and predictability in their JavaFX user interfaces. With a firm grasp of transparency handling, developers can elevate the aesthetic appeal and user experience of their Java applications.
In conclusion, transparency in JavaFX offers immense creative potential, but mastery of its intricacies is essential for achieving desired visual outcomes. By addressing the pitfalls and embracing best practices, developers can harness the power of transparency to create stunning user interfaces in JavaFX.
For more in-depth insights into JavaFX development and best practices, be sure to check out the official JavaFX documentation and the insightful community discussions on Stack Overflow.
Remember, mastering transparency in JavaFX is not only about making elements see-through, but also about making your code transparent and understandable for future developers. Happy coding!