Migrating from Java 7 to Java 8 File Channels
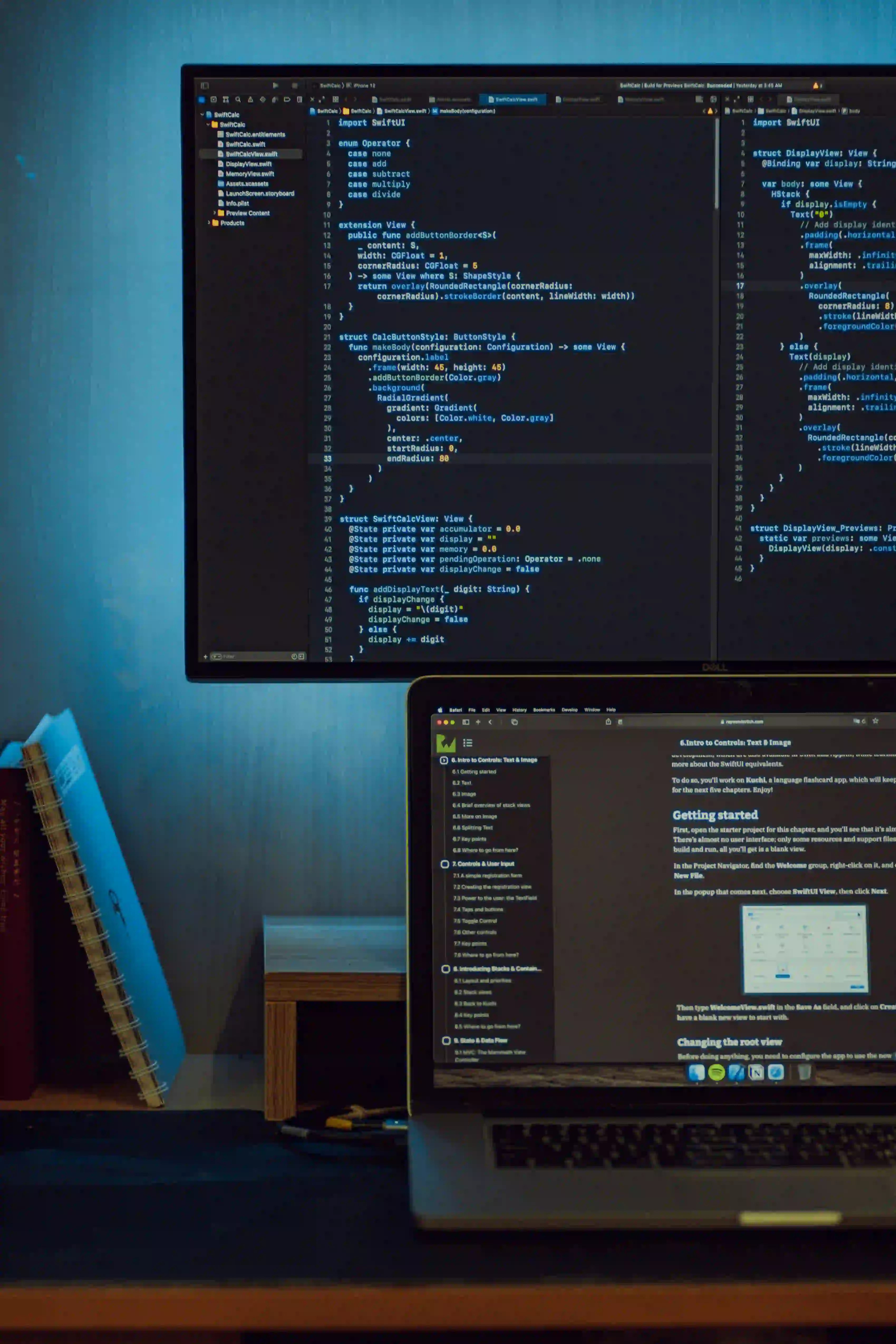
Migrating to Java 8 File Channels for Enhanced IO Operations
In this blog post, we will discuss the migration from using traditional IO operations in Java 7 to leveraging the powerful File Channel API introduced in Java 8 for enhanced IO operations. File Channels provide an efficient, low-level way of performing IO operations, and migrating to them can significantly improve the performance of file-related operations in your Java applications.
Traditional IO Operations in Java 7
In Java 7, the standard way of performing file IO operations involves using classes like FileInputStream
, FileOutputStream
, BufferedInputStream
, and BufferedOutputStream
. While these classes serve their purpose well, they have certain limitations when it comes to performance and flexibility.
Introducing Java 8 File Channels
Java 8 introduced the java.nio.channels.FileChannel
class, which provides a more flexible and efficient way of performing file IO operations. File Channels offer features such as memory-mapped IO, scatter/gather IO, and support for file locking, making them a powerful tool for handling IO operations in Java.
Migrating to File Channels
File Channel Basics
Let's start by examining how to obtain a FileChannel
from a FileInputStream
or a FileOutputStream
:
FileInputStream fileInputStream = new FileInputStream("input.txt");
FileChannel inputChannel = fileInputStream.getChannel();
FileOutputStream fileOutputStream = new FileOutputStream("output.txt");
FileChannel outputChannel = fileOutputStream.getChannel();
The getChannel()
method in both FileInputStream
and FileOutputStream
returns a FileChannel
associated with the file.
Reading from a File Using FileChannel
Here's an example of reading from a file using a FileChannel
:
try (FileChannel channel = FileChannel.open(Paths.get("input.txt"), StandardOpenOption.READ)) {
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (channel.read(buffer) > 0) {
buffer.flip();
// Process the data in the buffer
buffer.clear();
}
} catch (IOException e) {
// Handle exception
}
In this code snippet, we open a FileChannel
for reading using FileChannel.open()
and then read from the channel into a ByteBuffer
. Using a ByteBuffer
allows for more efficient IO operations.
Writing to a File Using FileChannel
Similarly, writing to a file using a FileChannel
can be done as follows:
try (FileChannel channel = FileChannel.open(Paths.get("output.txt"), StandardOpenOption.WRITE, StandardOpenOption.CREATE)) {
String data = "Hello, File Channel!";
ByteBuffer buffer = ByteBuffer.wrap(data.getBytes());
channel.write(buffer);
} catch (IOException e) {
// Handle exception
}
In this code snippet, we open a FileChannel
for writing using FileChannel.open()
and then write data to the channel using a ByteBuffer
.
Memory-Mapped IO with FileChannel
One of the most powerful features of FileChannel
is its support for memory-mapped IO. Memory-mapped IO allows a file to be mapped into memory, enabling direct manipulation of the file data using ByteBuffer
s. Here's an example of memory-mapped IO using FileChannel
:
try (FileChannel channel = FileChannel.open(Paths.get("input.txt"), StandardOpenOption.READ)) {
MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_ONLY, 0, channel.size());
// Process the data in the buffer
} catch (IOException e) {
// Handle exception
}
In this code snippet, we use the map()
method of a FileChannel
to map the entire file into memory as a MappedByteBuffer
.
Benefits of Migrating to File Channels
Performance
File Channels can offer better performance compared to traditional IO operations, especially when dealing with large files. Their support for memory-mapped IO allows for efficient manipulation of file data directly in memory.
Flexibility
File Channels provide more flexibility in handling IO operations. The support for scatter/gather IO, file locking, and memory-mapped IO opens up new possibilities for implementing advanced IO operations in Java.
Asynchronous IO
Java 7 IO operations can be blocking, which could lead to performance bottlenecks in high-throughput applications. However, File Channels in Java 8 offer asynchronous IO operations through CompletableFuture
s and CompletionHandler
s, enabling non-blocking IO.
Closing Remarks
Migrating from traditional IO operations in Java 7 to the File Channel API in Java 8 can bring significant performance and flexibility improvements to your file-related IO operations. By leveraging the advanced features of File Channels, such as memory-mapped IO, scatter/gather IO, and asynchronous IO, you can enhance the efficiency of your Java applications.
So, consider embracing File Channels in Java 8 for your file IO tasks, and unlock the full potential of efficient, low-level IO operations in your Java applications.
We hope this guide has provided you with valuable insights into the benefits and usage of File Channels in Java 8. Happy coding!
For further reading, you can refer to the official documentation on FileChannel and explore more advanced usage scenarios.