Optimizing Pull-to-Refresh Functionality in AndroidListView
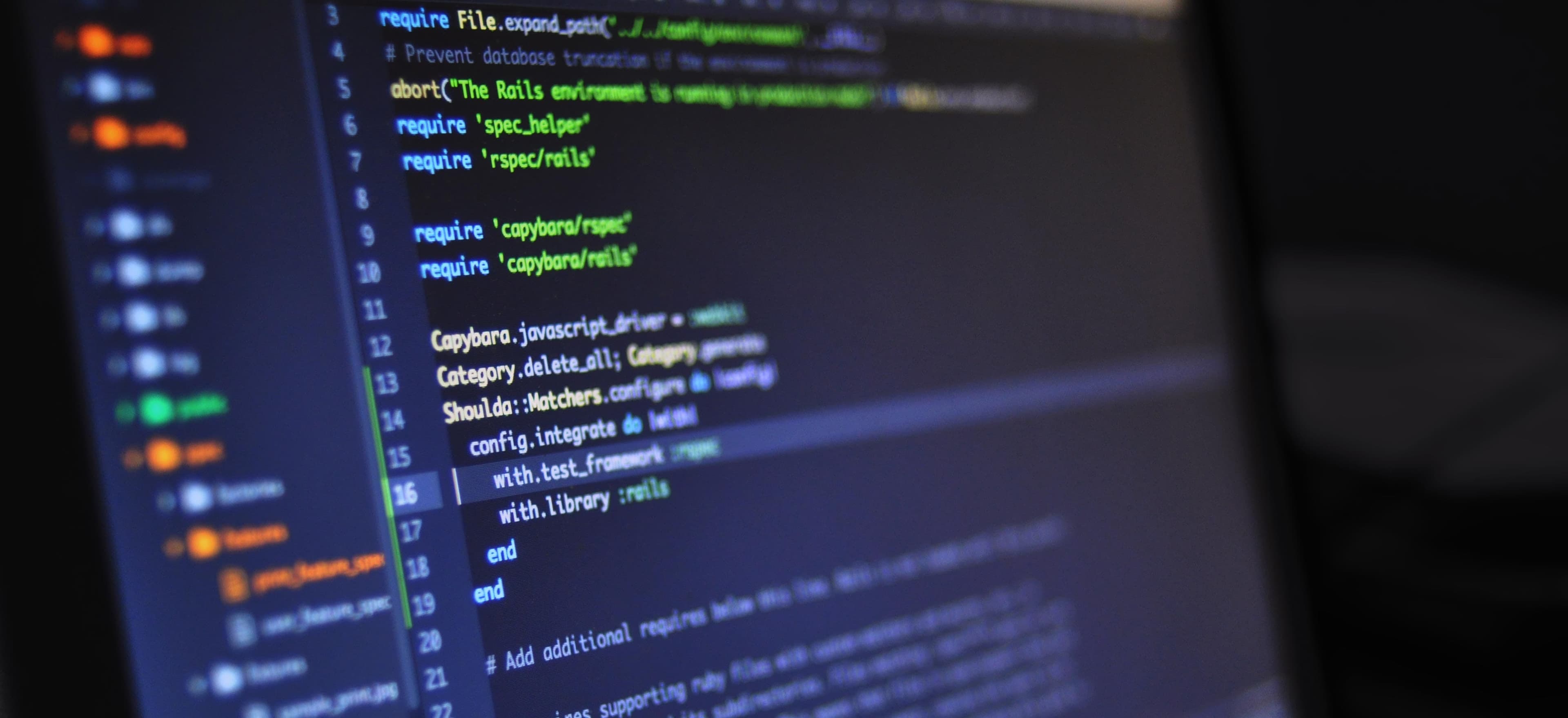
- Published on
Improving User Experience with Efficient Pull-to-Refresh in Android ListView
In today's competitive app market, user experience plays a pivotal role in determining an app's success. When it comes to displaying dynamic content in Android apps, the need for efficient and intuitive pull-to-refresh functionality cannot be overstated. In this article, we will explore how to optimize the pull-to-refresh feature for an Android ListView, leveraging Java for seamless integration and improved user experience.
Understanding Pull-to-Refresh
Before diving into the coding aspect, it's crucial to grasp the significance of pull-to-refresh. This feature allows users to update the content of a scrollable list by pulling the list down from the top. Pull-to-refresh is often used to fetch fresh data from a remote server, refreshing the list with the latest information. As a result, it enhances user engagement and ensures that users are presented with the most up-to-date content.
Implementing Pull-to-Refresh with SwipeRefreshLayout
To implement pull-to-refresh in an Android ListView efficiently, we can make use of the SwipeRefreshLayout
widget provided by the Android Support library. This widget simplifies the process of adding pull-to-refresh functionality to any scrollable view, including ListView.
Let's walk through a step-by-step guide on how to integrate SwipeRefreshLayout
into an Android app to enable pull-to-refresh for a ListView:
Step 1: Adding the SwipeRefreshLayout Widget to the Layout
<android.support.v4.widget.SwipeRefreshLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/swipeRefreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</android.support.v4.widget.SwipeRefreshLayout>
In the layout file, we have added the SwipeRefreshLayout
as the parent layout, containing the ListView as its child. This establishes the foundation for integrating pull-to-refresh functionality.
Step 2: Initializing SwipeRefreshLayout in the Java Code
// Initializing SwipeRefreshLayout
SwipeRefreshLayout swipeRefreshLayout = findViewById(R.id.swipeRefreshLayout);
// Setting refresh listener
swipeRefreshLayout.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() {
@Override
public void onRefresh() {
// Perform the actual data refresh operation here
refreshData();
}
});
In the Java code, we initialize the SwipeRefreshLayout
and set an OnRefreshListener
to handle the refresh operation when the user triggers a pull-to-refresh action.
Step 3: Implementing the refreshData() Method
private void refreshData() {
// Perform data fetching and update the ListView
fetchDataFromServer();
// Stop the refreshing indicator
swipeRefreshLayout.setRefreshing(false);
}
Within the refreshData()
method, we carry out the data-fetching operation, such as fetching updated content from a remote server. Upon completion of the data update, we stop the refreshing indicator by calling setRefreshing(false)
on the SwipeRefreshLayout
instance.
Optimizing the Pull-to-Refresh Process
While the integration of pull-to-refresh with SwipeRefreshLayout
provides the fundamental functionality, optimizing the refresh process is equally crucial for a seamless user experience. Let's explore some strategies to enhance the pull-to-refresh mechanism further.
Throttling Network Requests
Throttling network requests can prevent excessive data fetching when users perform multiple pull-to-refresh actions in quick succession. By incorporating a debouncing mechanism, we can control the frequency of network requests triggered by pull-to-refresh, thereby enhancing the app's performance and avoiding unnecessary server load.
// Implementing a debounce mechanism with a delay of 500 milliseconds
private long lastRefreshTime = 0;
public void onRefresh() {
if (System.currentTimeMillis() - lastRefreshTime > 500) {
lastRefreshTime = System.currentTimeMillis();
// Perform the data refresh operation
refreshData();
}
}
By introducing a time-based check, we ensure that the refreshData()
method is only invoked if a specific time interval has elapsed since the last refresh, thus preventing rapid and redundant network requests.
Visual Enhancements for Pull-to-Refresh
Incorporating visual indicators and animations during the pull-to-refresh process can elevate the user experience. By providing feedback through visual cues, such as progress spinners or animated pull-to-refresh arrows, users are informed about the ongoing refresh operation, creating a more engaging interaction.
// Customizing the color scheme of the swipe refresh indicator
swipeRefreshLayout.setColorSchemeColors(
getResources().getColor(R.color.colorPrimary),
getResources().getColor(R.color.colorAccent),
getResources().getColor(R.color.colorPrimaryDark)
);
With the setColorSchemeColors()
method, we can customize the color scheme of the swipe refresh indicator to align with the app's visual identity, thereby enhancing the overall aesthetic appeal during the pull-to-refresh action.
Bringing It All Together
In conclusion, integrating and optimizing pull-to-refresh functionality for an Android ListView using Java empowers developers to deliver a compelling user experience. By leveraging the SwipeRefreshLayout
widget and implementing performance enhancements, such as network request throttling and visual feedback, developers can ensure efficient data updates and an intuitive interaction model for users. Striking the right balance between functionality and visual appeal is pivotal in creating a cohesive pull-to-refresh experience that elevates the app's usability and engagement.
Incorporating these best practices for pull-to-refresh in Android ListView will undoubtedly contribute to a seamless and engaging user experience, setting your app apart in today's competitive landscape. Keep refining and evolving the pull-to-refresh mechanism to align with user expectations and preferences, reinforcing the app's position as a user-friendly and dynamic platform for accessing information and staying connected.
Integrating pull-to-refresh functionality with additional features, such as caching strategies, error handling, and adaptive UI updates, further enriches the user experience and strengthens the app's reliability. Embracing a user-centric approach and continuously refining the app's interaction patterns will undoubtedly solidify its position as a preferred choice for users seeking a compelling and responsive platform.
By continuously optimizing and refining the pull-to-refresh mechanism, app developers can deliver a seamless and rewarding user experience, fostering long-term user engagement and satisfaction.
Remember, the pull-to-refresh feature is not merely a functional necessity—it is an opportunity to delight users and elevate the app's appeal in a competitive digital landscape. Keep innovating and fine-tuning the pull-to-refresh experience to ensure that users are greeted with fresh content effortlessly and enjoy a consistently engaging experience.
Continuously refining and adapting the pull-to-refresh mechanism reinforces the app's commitment to user satisfaction and dynamic content delivery, positioning it as a responsive and user-friendly platform in the ever-evolving landscape of mobile applications.