Handling Service Degradation in Microservices Architectures
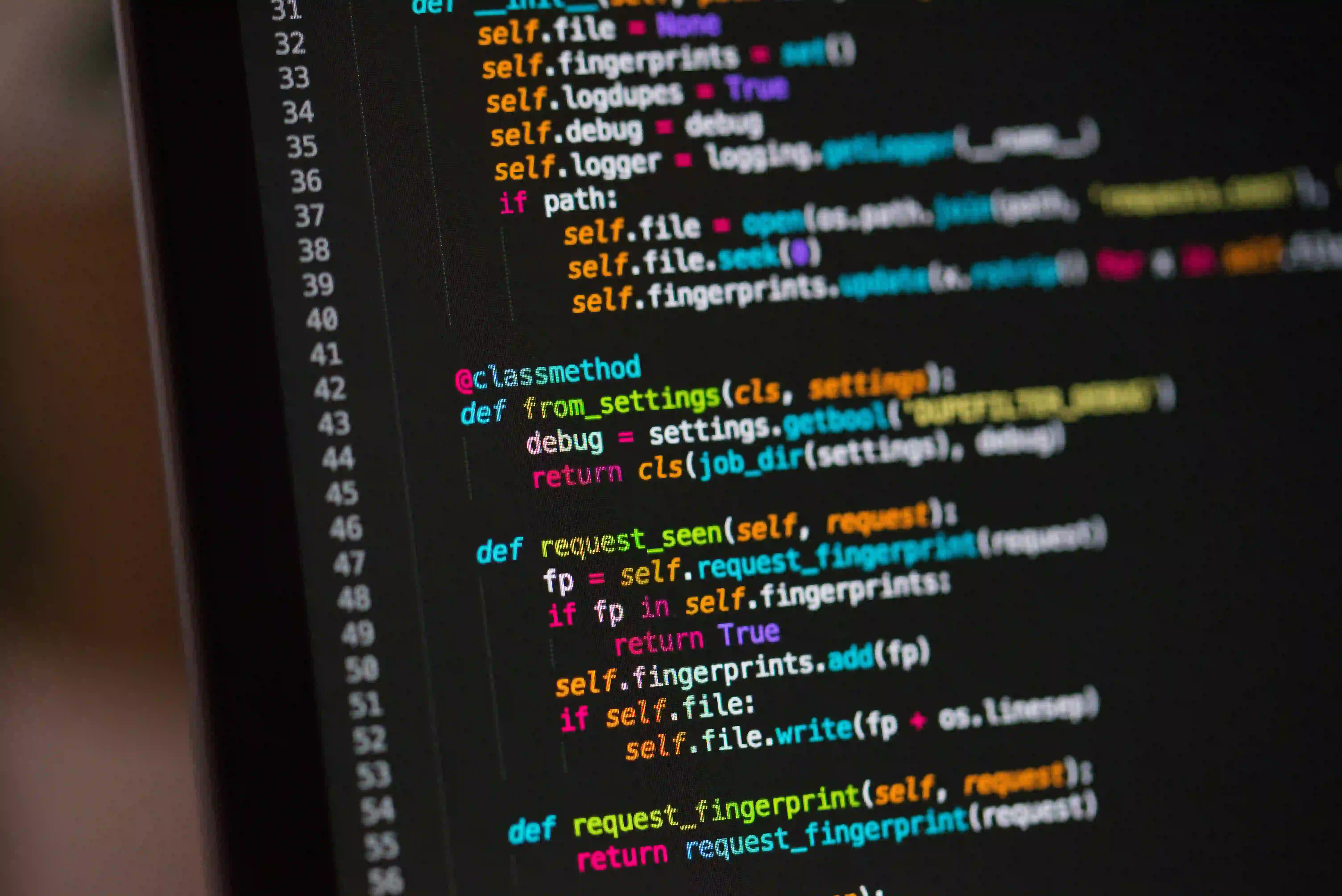
Handling Service Degradation in Microservices Architectures
In a microservices architecture, services are designed to be independent, decentralized, and resilient. However, service degradation can occur due to various reasons such as network latency, database overload, or third-party service failures. Therefore, it's crucial to implement strategies to handle service degradation effectively. In this post, we'll discuss some techniques and best practices to handle service degradation in Java-based microservices architectures.
Circuit Breaker Pattern
The circuit breaker pattern is a crucial component for handling service degradation in microservices architectures. It acts as a safeguard to prevent cascading failures and allows services to gracefully degrade when a dependent service is experiencing issues.
Example of Circuit Breaker Implementation in Java
public class MyService {
private CircuitBreaker circuitBreaker;
public MyService() {
CircuitBreakerConfig config = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.ringBufferSizeInClosedState(5)
.build();
circuitBreaker = CircuitBreaker.of("myServiceCircuitBreaker", config);
}
public String invokeRemoteService() {
return circuitBreaker.executeSupplier(() -> {
// Call remote service
return remoteServiceCall();
});
}
}
In the above example, we use the resilience4j
library to implement the circuit breaker pattern in Java. We configure the failure rate threshold and the ring buffer size in the closed state to control the behavior of the circuit breaker.
The invokeRemoteService
method demonstrates how to execute a remote service call within the circuit breaker. If the failure rate exceeds the specified threshold, the circuit breaker will open, preventing further calls to the remote service.
Bulkhead Pattern
Another essential pattern for handling service degradation is the bulkhead pattern. It isolates different parts of the system to prevent failures in one part from affecting other parts. This is particularly important in microservices architectures where services may share resources such as thread pools.
Example of Bulkhead Pattern Implementation in Java
public class MyService {
private ThreadPoolBulkhead bulkhead;
public MyService() {
ThreadPoolBulkheadConfig config = ThreadPoolBulkheadConfig.custom()
.maxThreadPoolSize(10)
.build();
bulkhead = ThreadPoolBulkhead.of("myServiceBulkhead", config);
}
public String processRequest() {
return bulkhead.executeSupplier(() -> {
// Process the request
return requestProcessing();
});
}
}
In this example, we use the resilience4j
library's ThreadPoolBulkhead
to implement the bulkhead pattern. We configure the maximum thread pool size to limit the concurrency of the processRequest
method.
Timeout Handling
Timeout handling is crucial for preventing services from getting stuck while waiting for a response from a slow or unresponsive dependency. In a microservices architecture, it's essential to define appropriate timeouts for invoking external services.
Example of Timeout Handling in Java
public class MyService {
public String invokeRemoteService() {
OkHttpClient client = new OkHttpClient.Builder()
.callTimeout(Duration.ofSeconds(5))
.build();
Request request = new Request.Builder()
.url("https://example.com")
.build();
try (Response response = client.newCall(request).execute()) {
return response.body().string();
} catch (IOException e) {
// Handle timeout or network issues
return "Error: " + e.getMessage();
}
}
}
In this example, we use the OkHttp
library to invoke a remote service with a defined call timeout of 5 seconds. If the request takes longer than the specified timeout, an exception will be thrown, and we can handle it accordingly.
Graceful Degradation
When handling service degradation, it's important to implement graceful degradation to provide a good user experience even when some parts of the system are not functioning optimally.
Example of Graceful Degradation in Java
public class MyService {
public String processRequest() {
try {
// Process the request
return requestProcessing();
} catch (Exception e) {
// Log the error and return a fallback response
log.error("Error processing request: {}", e.getMessage());
return "Fallback response";
}
}
}
In this example, the processRequest
method gracefully handles any exceptions that may occur during request processing. It logs the error and returns a fallback response to ensure that the user's request is still handled, even in the event of service degradation.
Lessons Learned
In a microservices architecture, handling service degradation is a critical aspect of ensuring the reliability and resilience of the system. By implementing patterns such as the circuit breaker, bulkhead, timeout handling, and graceful degradation, Java-based microservices can effectively handle service degradation and provide a consistent user experience.
By following these best practices and leveraging libraries such as resilience4j
and OkHttp
, Java developers can build robust and resilient microservices architectures that can gracefully handle service degradation scenarios.
To delve deeper into these concepts, I encourage you to explore the official documentation for resilience4j
and the OkHttp documentation. These resources provide comprehensive insights into implementing these patterns and best practices in Java-based microservices architectures.