Getting Started with AWS Lambda: A Complete Guide
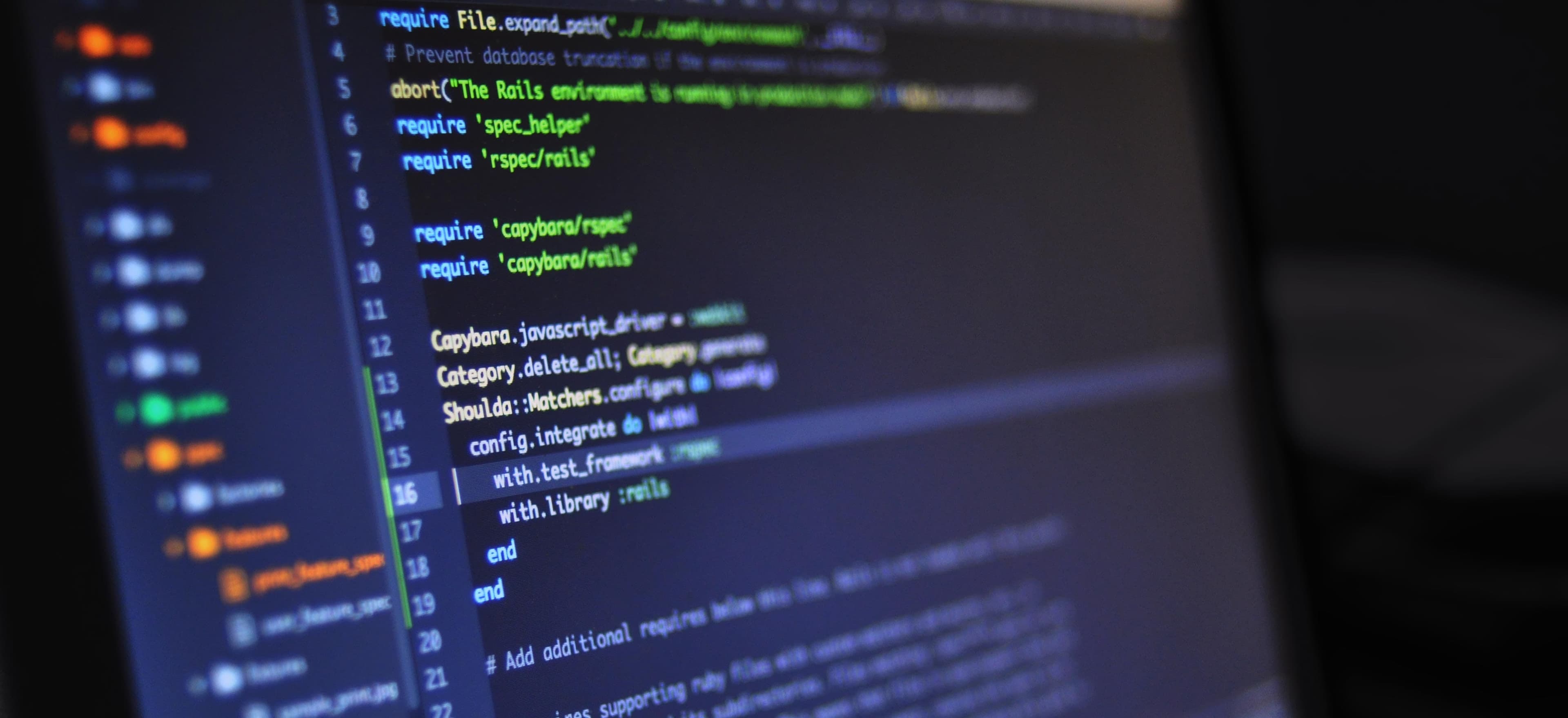
- Published on
Getting Started with AWS Lambda: A Complete Guide
AWS Lambda is a powerful serverless computing service that allows you to run code without provisioning or managing servers. In this guide, we'll explore the basics of using AWS Lambda with Java. We'll cover everything from setting up your environment to deploying your first Lambda function.
Prerequisites
Before we dive into using AWS Lambda with Java, make sure you have the following:
- An AWS account: You'll need an AWS account to use Lambda.
- Java Development Kit (JDK): Make sure you have Java installed on your machine.
Setting Up Your Environment
1. Setting Up AWS CLI
The AWS Command Line Interface (CLI) is a powerful tool that allows you to interact with AWS services from the command line. Install the AWS CLI by following the instructions here.
Once installed, run aws configure
and enter your AWS access key, secret key, and default region.
2. Setting Up AWS Toolkit for Eclipse
If you prefer using an Integrated Development Environment (IDE) like Eclipse, you can install the AWS Toolkit for Eclipse. This toolkit provides seamless integration with AWS services, including Lambda.
Follow the instructions to install the AWS Toolkit for Eclipse from the AWS Toolkit for Eclipse documentation.
Creating Your First Lambda Function
Now that you have your environment set up, let's create a simple Lambda function in Java.
1. Create a New Java Project
In your preferred IDE, create a new Java project. Make sure to include the AWS SDK for Java as a dependency in your project. You can do this by adding the following Maven dependency to your pom.xml
:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-lambda-java-core</artifactId>
<version>1.2.0</version>
</dependency>
2. Write Your Lambda Function
Create a new Java class for your Lambda function. Here's an example of a simple Lambda function that takes a string input and returns it in uppercase:
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class MyLambda implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
return input.toUpperCase();
}
}
In this example, the MyLambda
class implements the RequestHandler
interface provided by the AWS Lambda Java SDK. This interface has a handleRequest
method that you need to override. It takes the input type (String
in this case) and the output type (String
), and it also has a Context
parameter for additional information about the function's runtime environment.
3. Package Your Function
Once you've written your Lambda function, package it into a JAR file. Make sure to include any dependencies in the JAR file as well.
4. Deploy Your Function
You can deploy your Lambda function using the AWS CLI or the AWS Management Console. If you're using the CLI, you can use the following command:
aws lambda create-function --function-name MyLambda --zip-file fileb://path/to/your/jarfile.jar --handler com.example.MyLambda::handleRequest --runtime java11 --role arn:aws:iam::123456789012:role/lambda-role
In this command:
--function-name
is the name of your Lambda function.--zip-file
points to the location of your JAR file.--handler
specifies the fully qualified class name and the method name that AWS Lambda uses as the entry point for your function.--runtime
specifies the runtime for your function (in this case, Java 11).--role
is the Amazon Resource Name (ARN) of the IAM role that AWS Lambda assumes when it executes your function.
Testing Your Lambda Function
After deploying your Lambda function, you can test it using the AWS Management Console or the AWS CLI. You can invoke your function and pass in test input to see the result.
Invoking Your Function Using the AWS Management Console
- Navigate to the AWS Lambda console.
- Select your Lambda function.
- Click the "Test" button.
- Enter a test event and click "Test" to invoke your function.
Invoking Your Function Using the AWS CLI
You can also test your function using the AWS CLI. Use the following command to invoke your function with test input:
aws lambda invoke --function-name MyLambda --payload '{"input": "hello"}' output.txt
In this example, we're invoking the MyLambda
function with a JSON payload that contains the input "hello"
. The result will be written to the output.txt
file.
The Closing Argument
In this guide, we've covered the basics of getting started with AWS Lambda using Java. We've set up our environment, created a simple Lambda function, and deployed and tested it. AWS Lambda provides a powerful platform for running code without the hassle of managing servers, and integrating it with Java allows you to leverage the robustness of the Java ecosystem.
Now that you've built and deployed your first Lambda function, you can explore more advanced features and integrations to build scalable and efficient serverless applications using AWS Lambda and Java. Happy coding!