Handling ConcurrentHashMap Thread Interference
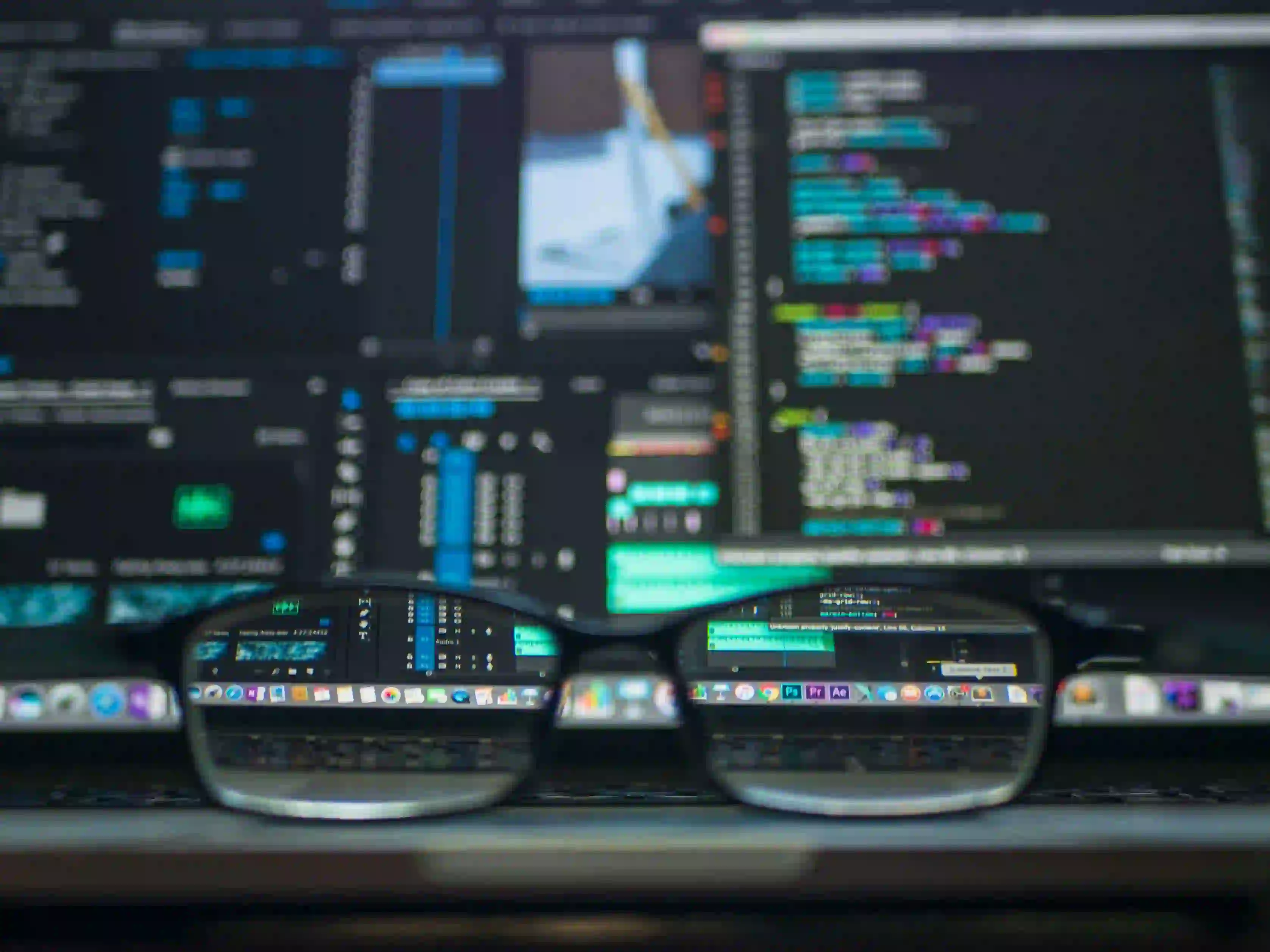
Handling ConcurrentHashMap Thread Interference
When working with multi-threaded applications in Java, handling thread interference is crucial to ensure data consistency and prevent race conditions. One of the common ways to address this issue is by using ConcurrentHashMap, which is a concurrent collection class provided by the Java Collections framework. In this article, we will delve into the nuances of handling thread interference with ConcurrentHashMap and explore best practices to mitigate such issues.
Understanding ConcurrentHashMap
ConcurrentHashMap is a thread-safe implementation of the Map interface, designed specifically to be used in a multi-threaded environment. It allows concurrent read and write operations without the need for external synchronization. This is achieved through the use of internal synchronization mechanisms, such as the use of a segmented structure and lock striping, to allow multiple threads to access and modify the map concurrently.
Dealing with Thread Interference
Thread interference in a ConcurrentHashMap can occur when multiple threads attempt to modify the map concurrently, leading to potential data corruption or inconsistent state. To mitigate thread interference, it's essential to utilize the concurrent operations provided by ConcurrentHashMap and adhere to best practices for concurrent programming.
Using putIfAbsent()
One of the common scenarios where thread interference can occur is when adding a new key-value pair to the map only if the key is absent. The putIfAbsent(key, value)
method in ConcurrentHashMap provides a solution to this problem by ensuring that the insertion is performed atomically, i.e., only if the specified key is not already associated with a value.
ConcurrentMap<String, String> map = new ConcurrentHashMap<>();
String key = "exampleKey";
String value = "exampleValue";
map.putIfAbsent(key, value);
In the above code snippet, the putIfAbsent()
method ensures that the key-value pair is added atomically, preventing thread interference during the insertion process.
Leveraging Atomic Operations
In addition to the built-in methods provided by ConcurrentHashMap, leveraging atomic operations can further help in mitigating thread interference. The compute()
method, introduced in Java 8, allows for atomic updates based on the existing value associated with a key.
ConcurrentMap<String, Integer> map = new ConcurrentHashMap<>();
String key = "exampleKey";
map.compute(key, (k, v) -> (v == null) ? 1 : v + 1);
In the above example, the compute()
method atomically updates the value associated with the key, ensuring that the modification is performed consistently across multiple threads.
Best Practices for Concurrent Modification
When working with ConcurrentHashMap, it's vital to follow best practices to minimize the risk of thread interference and ensure data consistency.
-
Minimize Lock Contention: ConcurrentHashMap uses internal concurrency control mechanisms to minimize lock contention. However, it's essential to design your application in a way that reduces the need for excessive locking.
-
Utilize Concurrent Operations: Leverage the concurrent methods provided by ConcurrentHashMap, such as
putIfAbsent()
andcompute()
, to perform atomic operations without the need for explicit synchronization. -
Atomicity: Ensure that operations performed on the ConcurrentHashMap are atomic, meaning they are indivisible and appear to the rest of the system as if they occurred instantaneously.
-
Iterating Safely: When iterating over a ConcurrentHashMap, consider using the
keySet()
orentrySet()
with enhanced for loops to ensure safe iteration without the risk of concurrent modifications.
The Closing Argument
In a multi-threaded environment, handling thread interference is paramount to maintaining data consistency and integrity. By leveraging ConcurrentHashMap and adhering to best practices for concurrent modification, you can effectively mitigate thread interference and ensure the reliability of your application.
To delve deeper into the intricacies of ConcurrentHashMap and concurrent programming in Java, Oracle's official documentation provides comprehensive insights and guidelines. Additionally, exploring advanced topics such as lock striping and internal mechanisms of ConcurrentHashMap can further enhance your understanding of concurrent data structures in Java.
In summary, mastering the art of handling thread interference with ConcurrentHashMap empowers you to build robust, scalable, and thread-safe applications in Java.