Optimizing JUnit Test Structure for Efficient Testing
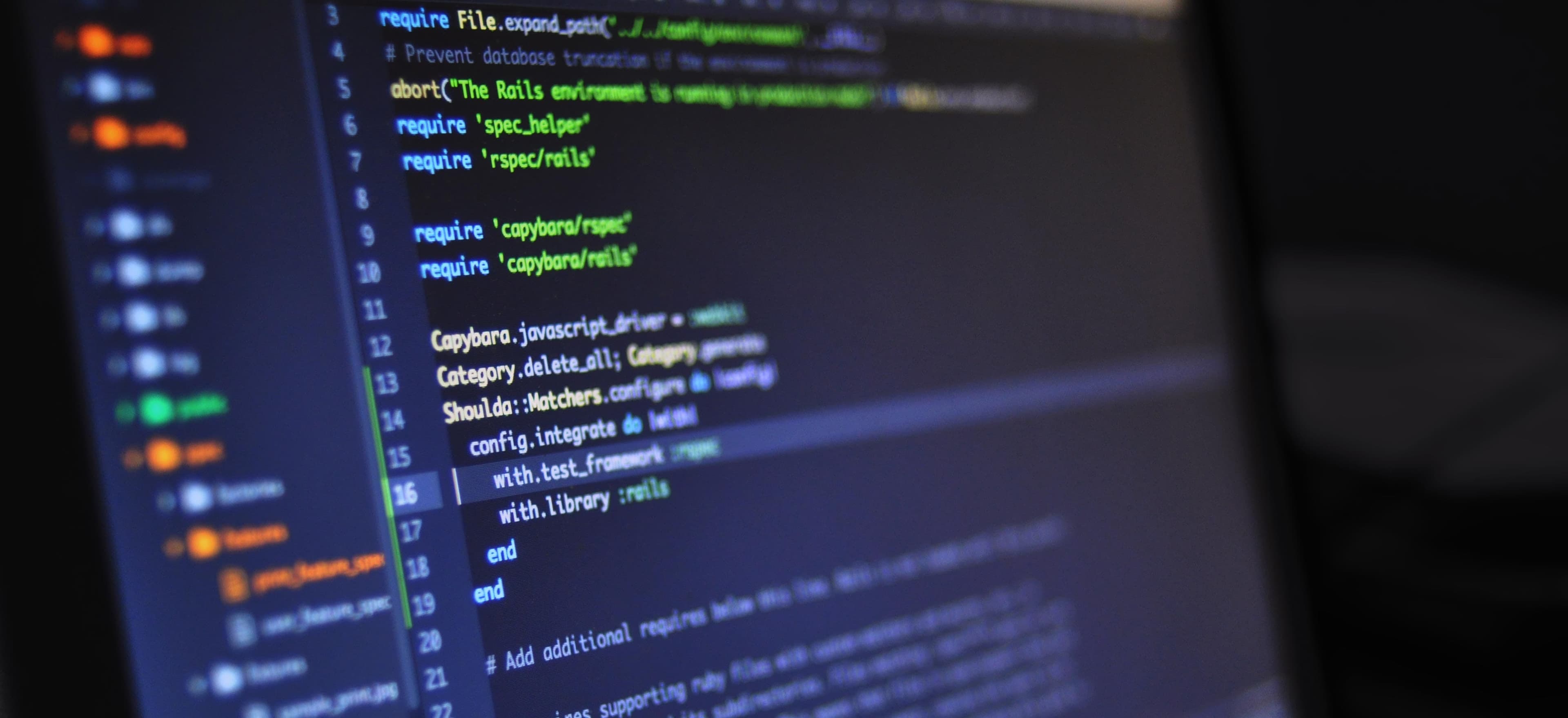
- Published on
Optimizing JUnit Test Structure for Efficient Testing
In the world of Java development, writing robust and reliable code is not enough. It's crucial to ensure that the code behaves as expected and continues to do so even after future changes. This is where JUnit, the most popular testing framework for Java, comes into play. JUnit allows developers to write and run repeatable automated tests, ensuring that their code functions as intended. However, writing JUnit tests effectively requires more than just basic knowledge of the framework. In this post, we'll discuss how to optimize the structure of your JUnit tests for efficient and effective testing.
Understanding JUnit Test Structure
Before diving into optimization techniques, let's review the basic structure of a JUnit test. A JUnit test typically consists of three main sections:
-
Setup: This section involves preparing the necessary preconditions for the test. It often includes initializing objects, setting up mocks, and configuring the test environment.
-
Execution: This section is where the actual test logic is executed. It involves calling the methods being tested and making assertions about the expected behavior or state.
-
Teardown: Also known as cleanup, this section is responsible for releasing resources, resetting the environment, and cleaning up any state created during the setup or execution phases.
Understanding these three sections is crucial for writing effective JUnit tests. Now, let's explore some optimization techniques for each of these sections.
Optimizing the Setup Phase
The setup phase is where you prepare the environment for the test. To optimize this phase, consider the following techniques:
Use @Before and @BeforeClass Annotations
Why: The @Before
and @BeforeClass
annotations are provided by JUnit to designate methods that need to run before each test and before all tests in the class, respectively.
Example:
public class MyTest {
private SomeObject obj;
@Before
public void setUp() {
obj = new SomeObject();
}
}
By using these annotations, you can avoid code duplication and ensure that the setup code is executed consistently before each test.
Utilize Parameterized Tests
Why: Parameterized tests allow you to run the same test logic with different inputs. This is useful for testing the same behavior with multiple sets of data.
Example:
@RunWith(Parameterized.class)
public class ParameterizedTest {
@Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 1, 2, 3 },
{ 5, 5, 10 }
});
}
private int a;
private int b;
private int expectedResult;
public ParameterizedTest(int a, int b, int expectedResult) {
this.a = a;
this.b = b;
this.expectedResult = expectedResult;
}
@Test
public void testAddition() {
assertEquals(expectedResult, MathUtils.add(a, b));
}
}
Parameterized tests help to keep your test code concise and DRY (Don't Repeat Yourself) by testing multiple cases with minimal duplication.
Optimizing the Execution Phase
The execution phase is where the actual test logic is run. To optimize this phase, consider the following techniques:
Use Descriptive and Readable Assertions
Why: Descriptive and readable assertions enhance the understandability of the test cases, making it easier to pinpoint issues when a test fails.
Example:
@Test
public void testAddition() {
int result = MathUtils.add(2, 3);
assertEquals("Addition of 2 and 3 should be 5", 5, result);
}
By providing descriptive messages with assertions, you improve the clarity of the test's intent and make it easier for developers to diagnose failures.
Leverage Custom Matchers
Why: Custom matchers allow you to define your own assertion logic, making the tests more expressive and aligned with the specific domain of your code.
Example:
public class CustomMatchers {
public static Matcher<List<String>> containsStringIgnoreCase(String expected) {
return new TypeSafeMatcher<>() {
@Override
protected boolean matchesSafely(List<String> actual) {
return actual.stream().anyMatch(s -> s.equalsIgnoreCase(expected));
}
@Override
public void describeTo(Description description) {
description.appendText("should contain string (case-insensitive): ").appendValue(expected);
}
};
}
}
By creating custom matchers, you can write assertions that are directly aligned with the requirements of your code, leading to more meaningful test cases.
Optimizing the Teardown Phase
The teardown phase is responsible for cleaning up resources and resetting the environment after the test. To optimize this phase, consider the following techniques:
Use @After and @AfterClass Annotations
Why: The @After
and @AfterClass
annotations are counterparts to @Before
and @BeforeClass
that handle cleanup operations.
Example:
public class MyTest {
private TemporaryFile file;
@Before
public void setUp() {
file = createTemporaryFile();
}
@After
public void tearDown() {
file.delete();
}
}
These annotations ensure that cleanup logic is consistently executed after each test or after all tests in the class.
Utilize try-with-resources for External Resources
Why: When dealing with external resources such as files or network connections, using Java's try-with-resources statement ensures proper resource management and automatic cleanup.
Example:
@Test
public void testFileRead() {
try (FileReader reader = new FileReader("test.txt")) {
// Test file reading logic
} catch (IOException e) {
// Handle exception
}
}
By using try-with-resources, you can prevent resource leaks and ensure that resources are properly released after the test execution.
To Wrap Things Up
In conclusion, optimizing the structure of your JUnit tests is essential for efficient and effective testing. By applying the discussed techniques to the setup, execution, and teardown phases of your tests, you can improve the readability, maintainability, and reliability of your test suite. To delve deeper into JUnit best practices, I recommend exploring the official JUnit documentation and the book "Effective JUnit" by Scott Stirling.
Remember, writing good tests is just as important as writing good code. Embrace these optimization techniques and strive for a test suite that provides confidence in the correctness of your Java code. Happy testing!