"Memory Management: Understanding OutOfMemoryError"
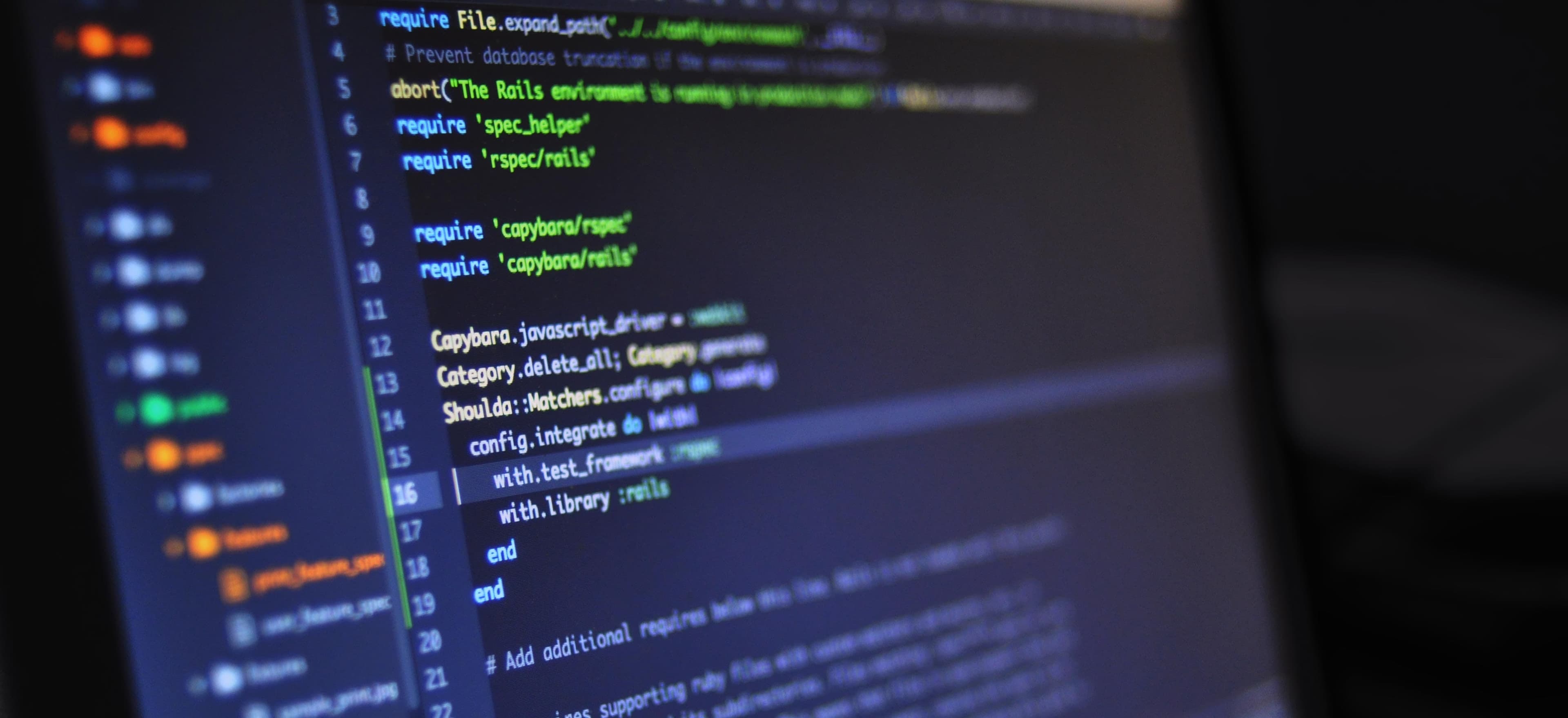
- Published on
Understanding OutOfMemoryError in Java
Memory management is a critical aspect of Java programming, and understanding the common issue of OutOfMemoryError is essential for writing efficient and robust applications. In this article, we will delve into the causes of OutOfMemoryError, how to diagnose it, and techniques to prevent and handle it effectively.
What is OutOfMemoryError?
OutOfMemoryError is a runtime exception thrown when the Java Virtual Machine (JVM) cannot allocate an object in the heap space due to insufficient memory. This error typically occurs when an application attempts to use more memory than the JVM can provide, leading to the JVM unable to fulfill allocation requests.
Common Causes of OutOfMemoryError
Memory Leaks
Memory leaks occur when an application holds references to objects that are no longer needed, preventing them from being garbage collected. This leads to a gradual consumption of memory, eventually causing an OutOfMemoryError.
Insufficient Heap Space
If the heap size allocated to the JVM is too small to accommodate the application's memory requirements, it can lead to OutOfMemoryError. The default heap size may not be sufficient for memory-intensive applications, requiring manual adjustment.
Large Object Creation
Creating large objects or large number of objects without proper memory management can quickly deplete the heap space, resulting in an OutOfMemoryError.
Diagnosing OutOfMemoryError
When encountering an OutOfMemoryError, it's crucial to diagnose the root cause to effectively address the issue. Here are some techniques for diagnosing OutOfMemoryError:
Analyzing Heap Dumps
Heap dump analysis provides valuable insights into memory usage, object retention, and potential memory leaks. Tools like VisualVM and YourKit can be used to capture and analyze heap dumps for identifying memory consumption patterns.
Monitoring and Profiling Tools
Utilizing monitoring and profiling tools like JConsole, JVisualVM, or Java Mission Control can help in identifying memory hotspots, memory leaks, and understanding memory allocation behavior of the application.
Techniques to Prevent OutOfMemoryError
Proper Object Lifecycle Management
Ensure that objects are released and dereferenced when they are no longer needed. This includes explicitly setting objects to null when they are no longer in use, enabling the garbage collector to reclaim memory efficiently.
Efficient Data Structures and Algorithms
Optimizing data structures and algorithms to minimize memory usage can significantly reduce the risk of encountering OutOfMemoryError.
Heap Size Configuration
Adjusting the heap size according to the application's memory requirements can prevent OutOfMemoryError. This can be achieved by setting appropriate values for -Xms
(initial heap size) and -Xmx
(maximum heap size) JVM options.
Memory Profiling and Tuning
Regular memory profiling and tuning can help in identifying memory bottlenecks and optimizing memory usage to prevent OutOfMemoryError.
Handling OutOfMemoryError
When encountering an OutOfMemoryError, it's essential to handle it gracefully to prevent application crashes and provide better user experience. Here are some techniques for handling OutOfMemoryError:
Logging and Alerting
Implementing proper logging and alerting mechanisms can help in identifying OutOfMemoryError occurrences in production environments and take necessary actions to mitigate the impact.
Graceful Degradation
Designing the application to gracefully degrade its functionality when encountering memory constraints can prevent catastrophic failures and improve the overall stability of the system.
Restarting Application
In scenarios where memory leaks are difficult to identify and resolve, periodic application restarts can serve as a temporary workaround to clear up memory and prevent prolonged downtime.
Closing the Chapter
OutOfMemoryError is a common yet critical issue in Java applications, and understanding its causes, diagnosis techniques, prevention methods, and handling strategies is essential for writing reliable and efficient software. By following best practices in memory management, diagnosing issues promptly, and implementing preventive measures, developers can mitigate the risk of OutOfMemoryError and ensure optimal application performance.
Remember, proactive memory management is key to keeping your Java applications running smoothly and avoiding the pitfalls of OutOfMemoryError.
So, keep coding, keep optimizing, and keep the memory in check!
For more in-depth understanding of memory management and Java performance optimization, consider exploring the book "Java Performance: The Definitive Guide", which provides comprehensive insights into Java performance tuning and memory management.
Happy coding!