JDK 9 Update: Navigating NotNullOrElse in Java
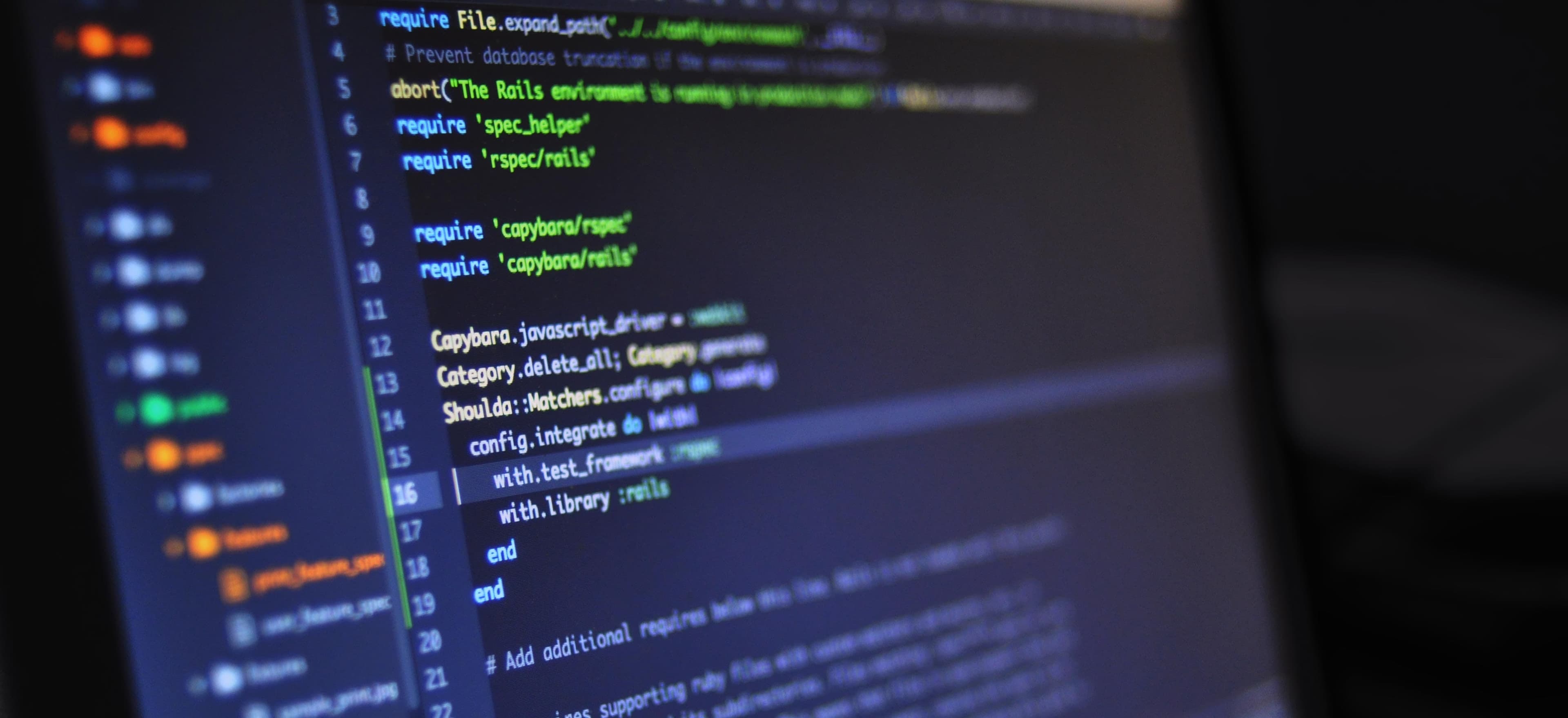
- Published on
Understanding NotNullOrElse in Java
In this post, we will delve into the concept of NotNullOrElse
introduced in JDK 9. We'll explore its significance and how it can enhance the overall development experience for Java programmers.
What is NotNullOrElse?
NotNullOrElse
is a new method added to the Objects
class in JDK 9. It provides a concise way to handle scenarios where you need to perform an action if an object is not null, and another action if it is null. This new addition streamlines and simplifies the code by eliminating the need for verbose null checks and conditional statements.
Examining the Syntax
The syntax of NotNullOrElse
is straightforward:
public static <T> T notNullOrElse(T obj, T defaultObj)
Here, obj
is the object to be checked for null, and defaultObj
is the value to be returned if obj
is null.
The return value of NotNullOrElse
will be obj
if it is not null, or defaultObj
if obj
is null.
Understanding the Advantage
Prior to JDK 9, achieving the behavior facilitated by NotNullOrElse
required writing explicit if-else statements, which increased the verbosity of the code. With the introduction of NotNullOrElse
, developers can now achieve the same outcome using a single method call. This not only makes the code more readable, but also reduces the possibility of null pointer exceptions, thereby contributing to a more robust and reliable codebase.
Let's delve into an example to illustrate the utility of NotNullOrElse
.
Example Usage
Consider the scenario where we want to assign a default value to a variable if it is null. Traditionally, this would involve writing an explicit null check and fallback logic. With NotNullOrElse
, this task becomes significantly more concise. Let's take a look at the following example:
String input = null;
String validInput = Objects.notNullOrElse(input, "Default");
System.out.println(validInput); // Output: Default
In the example above, if input
is null, it is replaced with the default value "Default" without having to resort to verbose null checks and conditional statements. This simplifies the code and improves its readability.
Use Case
The NotNullOrElse
method is particularly useful when dealing with method parameters or class fields that need default values in case of null. By leveraging NotNullOrElse
, you can streamline the handling of null values without cluttering the codebase with unnecessary conditional logic.
Handling Collections
NotNullOrElse
is not limited to handling single objects; it is equally applicable to collections. When dealing with collections, it provides a convenient way to handle null collections by providing a default value.
Let's consider a scenario where we want to work with a list and provide a default empty list if the original list is null:
List<String> myList = null;
List<String> validList = Objects.notNullOrElse(myList, Collections.emptyList());
System.out.println(validList); // Output: []
In this case, if myList
is null, it is replaced with an empty list. This simplifies the code and ensures that subsequent operations on the list won't result in null pointer exceptions.
Wrapping Up
In conclusion, the addition of NotNullOrElse
to the Objects
class in JDK 9 is a valuable enhancement that simplifies null handling and contributes to writing clearer, more concise, and less error-prone code. By leveraging this method, developers can streamline the handling of null values in their codebase, leading to more robust and maintainable applications.
In your Java development journey, remember that understanding the latest additions to the language and its libraries can significantly impact your ability to write efficient and maintainable code. Stay updated and leverage the power of new features to enhance your development experience.
So why not give NotNullOrElse
a try and see how it can streamline your code?