Top 9 Java Security Pitfalls Developers Need to Dodge
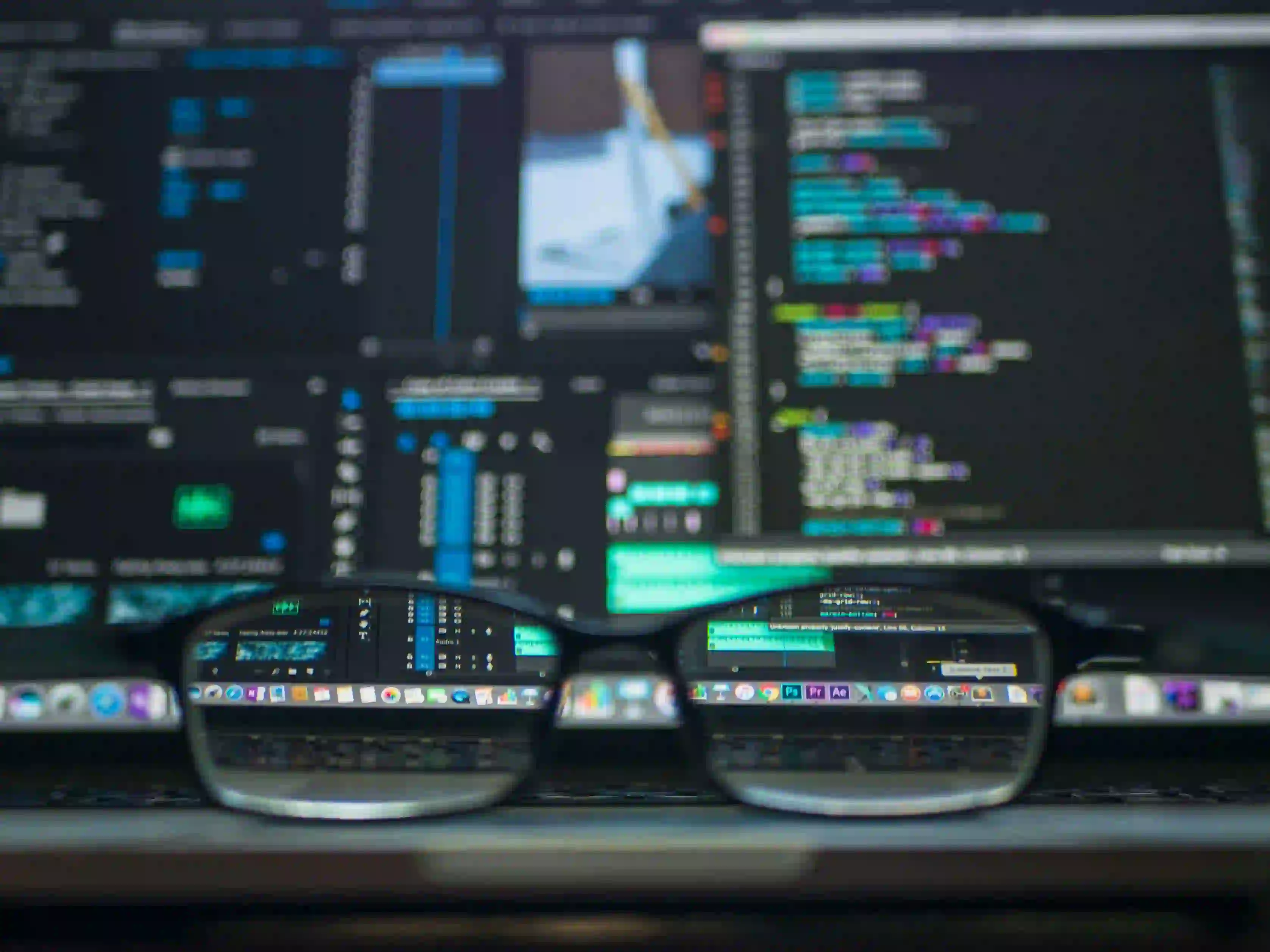
Top 9 Java Security Pitfalls Developers Need to Dodge
Java is a powerful and versatile language, widely used for building enterprise-level applications, web services, and Android apps. However, its flexibility can lead to security vulnerabilities if not handled properly. In this article, we'll delve into the top 9 Java security pitfalls that developers need to be aware of and how to avoid them.
1. Injection Attacks
What are Injection Attacks?
Injection attacks occur when untrusted data is sent to an interpreter as part of a command or query. In Java, the most common types of injection attacks are SQL injection, where malicious SQL commands are inserted into input fields, and code injection, where untrusted code is executed by the Java runtime.
How to Dodge Injection Attacks
Prevent injection attacks by using parameterized queries with PreparedStatement or using Object Relational Mapping (ORM) frameworks like Hibernate. Always validate and sanitize user inputs and use input validation libraries such as OWASP ESAPI.
String query = "SELECT * FROM users WHERE username = ?";
PreparedStatement statement = connection.prepareStatement(query);
statement.setString(1, userInput);
ResultSet result = statement.executeQuery();
2. Cross-Site Scripting (XSS)
What is Cross-Site Scripting?
Cross-Site Scripting is a vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. In Java, this can occur if user inputs are not properly validated and sanitized before being displayed on web pages, potentially leading to the execution of malicious scripts on the client's browser.
How to Avoid Cross-Site Scripting
To avoid XSS vulnerabilities, encode user inputs before displaying them in web pages. Use libraries like Apache Commons Text or OWASP Java Encoder to HTML-encode user inputs.
String userInput = "<script>alert('XSS Attack')</script>";
String encodedInput = StringEscapeUtils.escapeHtml4(userInput);
3. Deserialization Vulnerabilities
What are Deserialization Vulnerabilities?
Deserialization vulnerabilities arise from insecure deserialization of untrusted data, which can lead to remote code execution and other attacks. In Java, this is a critical security concern, especially when dealing with serialized objects from untrusted sources.
How to Address Deserialization Vulnerabilities
Avoid deserialization vulnerabilities by using safe deserialization practices, such as using whitelisting to restrict the classes that can be deserialized, and ensuring that serialized objects are not received from untrusted sources.
ObjectInputStream ois = new ObjectInputStream(socket.getInputStream());
ois.enableResolveObject(false);
MyObject obj = (MyObject) ois.readObject();
4. Insecure Cryptographic Practices
What are Insecure Cryptographic Practices?
Insecure cryptographic practices involve the improper use of cryptographic algorithms, weak key management, or insecure random number generation. These practices can lead to data breaches, integrity violations, and compromised security.
How to Secure Cryptographic Practices
Use strong cryptographic algorithms from the Java Cryptography Architecture (JCA) providers. Always use secure random number generators, strong key lengths, and up-to-date encryption algorithms. Avoid using custom cryptographic algorithms and ensure secure key management practices.
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256);
SecretKey secretKey = keyGen.generateKey();
5. Insecure File Handling
What is Insecure File Handling?
Insecure file handling occurs when developers do not adequately control file operations, leading to directory traversal attacks, file disclosure, and unauthorized file access. In Java, this can occur when handling file uploads, file permissions, and file I/O operations.
How to Secure File Handling
Always validate file paths, perform proper file permission checks, and sanitize file inputs. Use high-level libraries like Apache Commons IO for file operations to avoid low-level security pitfalls.
File file = new File("/path/to/secure/directory");
if(file.canRead() && file.canWrite()) {
// Perform file operations
}
6. Lack of Input Validation
What is Lack of Input Validation?
Lack of input validation allows attackers to insert malicious input that can compromise the application's security. In Java, this can occur in web applications, APIs, and user inputs where data is not properly validated for type, length, format, and range.
How to Validate Input
Always validate and sanitize user inputs using regex, validation libraries, and input validation frameworks like Hibernate Validator. Additionally, perform server-side input validation to complement client-side validation.
@NotNull
@Size(min=8, max=20)
@Pattern(regexp="^[a-zA-Z0-9]+$")
private String username;
7. Insufficient Authentication and Session Management
What is Insufficient Authentication and Session Management?
Insufficient authentication and session management can lead to unauthorized access, session hijacking, and account takeover. In Java, this can occur when authentication mechanisms are weak, session tokens are not securely managed, or persistent sessions are not properly invalidated.
How to Ensure Strong Authentication and Session Management
Use strong authentication mechanisms like multi-factor authentication, enforce secure password policies, and implement secure session management using frameworks like Spring Security. Always use secure, random session tokens and ensure session tokens are invalidated after logout or a period of inactivity.
// Using Spring Security for authentication and session management
http
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/")
.failureUrl("/login?error")
.permitAll()
.and()
.logout()
.logoutUrl("/logout")
.logoutSuccessUrl("/login?logout")
.invalidateHttpSession(true);
8. Overlooking Security Headers
What are Security Headers?
Security headers are HTTP response headers that provide security controls against common web exploits. In Java web applications, overlooking security headers such as Content Security Policy (CSP), HTTP Strict Transport Security (HSTS), and Cross-Origin Resource Sharing (CORS) can leave applications vulnerable to various attacks.
How to Implement Security Headers
Configure security headers in Java web applications using frameworks like Spring Security or by directly setting headers in web server configurations. Always include security headers like CSP, HSTS, X-Frame-Options, and X-Content-Type-Options to enhance web application security.
// Setting security headers in Spring Security configuration
http
.headers()
.contentSecurityPolicy("script-src 'self'");
9. Using Outdated Libraries and Components
Risks of Using Outdated Libraries and Components
Using outdated libraries and components in Java applications can expose the application to known vulnerabilities, as these libraries may not receive security updates or patches. This can lead to exploitability and compromise of the entire application.
How to Mitigate Risks of Outdated Libraries
Regularly update and patch libraries using build tools like Maven or Gradle. Use software composition analysis tools like OWASP Dependency-Check to identify and update outdated dependencies. Consider using libraries backed by organizations with active support and maintenance.
<!-- Using Maven to handle dependencies -->
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
The Last Word
Java, with its rich ecosystem and vast capabilities, requires careful attention to security best practices to avoid falling into common pitfalls. By understanding and proactively addressing these security pitfalls -- including injection attacks, XSS vulnerabilities, insecure deserialization, cryptographic practices, file handling, input validation, authentication, session management, security headers, and library management -- developers can build robust, secure Java applications that safeguard against potential threats.
Ensuring secure coding practices, staying updated with the latest security trends, and leveraging tools and frameworks can contribute significantly to building resilient Java applications that protect sensitive data and maintain user trust.
Incorporating security into the development life cycle from the outset, fostering a security-aware culture, and conducting periodic security assessments are paramount in fortifying Java applications against evolving cyber threats. By conscientiously addressing these security pitfalls, developers can bolster the security posture of their Java applications and bolster their resilience against cyber threats.