Mastering Java: Avoid Serialization Pitfalls with Proxy Pattern!
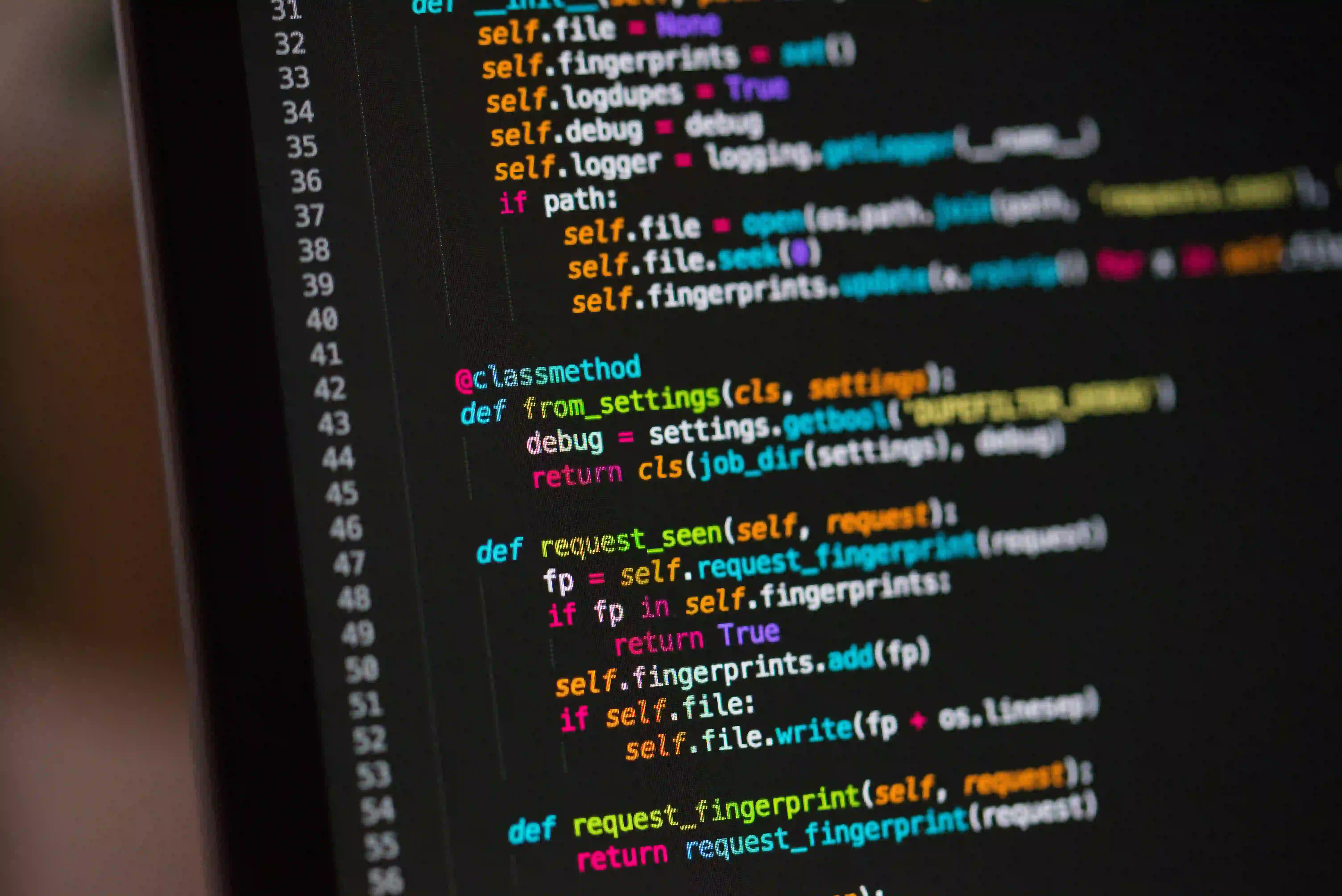
Mastering Java: Avoid Serialization Pitfalls with Proxy Pattern
Serialization is a powerful mechanism in Java that allows objects to be converted into a stream of bytes for storage or transmission and subsequently reconstructed. However, it comes with its own set of challenges, particularly when it comes to versioning, security, and performance. In this blog post, we'll explore how the Proxy Pattern can be leveraged to address these challenges and ensure a robust and efficient serialization process in Java.
Understanding Serialization Pitfalls
When dealing with serialization in Java, several pitfalls need to be carefully navigated to ensure the integrity and security of the serialized objects. Some of the common challenges include:
Versioning
As the application evolves, the structure of the serialized objects may change. This can lead to compatibility issues when deserializing objects that were serialized using an older version of the class.
Security
Serialization and deserialization of objects can potentially introduce security vulnerabilities, such as the execution of arbitrary code from deserialized objects (a.k.a. "serialization gadgets").
Performance
The default serialization mechanism in Java can be less performant, leading to large serialized payloads and slower serialization/deserialization processes.
Introducing the Proxy Pattern
The Proxy Pattern is a structural design pattern that provides a surrogate or placeholder for another object to control access to it. In the context of serialization, the Proxy Pattern can be used to address the aforementioned pitfalls by providing a controlled and version-independent mechanism for object serialization and deserialization.
Implementing the Proxy Pattern for Serialization
Let's take a look at how the Proxy Pattern can be implemented to overcome the challenges associated with serialization in Java.
Versioning with Proxy Pattern
import java.io.*;
class ProxyObject implements Serializable {
private static final long serialVersionUID = 1L;
private ActualObject actualObject;
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject();
out.writeObject(actualObject);
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
actualObject = (ActualObject) in.readObject();
}
}
In the above example, the ProxyObject
acts as a version-independent surrogate for the ActualObject
. By explicitly defining the writeObject
and readObject
methods, versioning issues can be mitigated, ensuring compatibility during deserialization.
Security with Proxy Pattern
class SecureProxyObject implements Serializable {
private static final long serialVersionUID = 1L;
private transient ActualObject actualObject;
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject();
// Perform security checks
out.writeObject(actualObject);
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
// Perform security checks
actualObject = (ActualObject) in.readObject();
}
}
In this example, the SecureProxyObject
introduces security checks during serialization and deserialization by explicitly controlling the write and read operations. The use of the transient
keyword prevents the actualObject
from being serialized directly, further enhancing security.
Performance with Proxy Pattern
class EfficientProxyObject implements Externalizable {
private ActualObject actualObject;
@Override
public void writeExternal(ObjectOutput out) throws IOException {
// Implement efficient serialization logic
out.writeObject(actualObject);
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
// Implement efficient deserialization logic
actualObject = (ActualObject) in.readObject();
}
}
By implementing the Externalizable
interface, the EfficientProxyObject
gains full control over the serialization and deserialization process. This allows for the implementation of custom, more efficient logic tailored to the specific requirements of the ActualObject
.
The Bottom Line
Serialization in Java is a powerful feature that comes with its own set of challenges, including versioning, security, and performance. By leveraging the Proxy Pattern, these challenges can be effectively addressed, ensuring robust, version-independent, secure, and efficient serialization and deserialization processes.
In this blog post, we explored how the Proxy Pattern can be used to overcome serialization pitfalls in Java, providing a glimpse into its practical implementation for versioning, security, and performance optimization.
By mastering the Proxy Pattern and its application in the realm of serialization, Java developers can ensure the seamless and secure interchange of objects while maintaining optimal performance. This paves the way for resilient and version-agnostic serialization solutions, laying a strong foundation for complex and evolving Java applications.
To delve deeper into the Proxy Pattern and its diverse applications in Java, check out the official Java documentation and the insightful guidance provided by the renowned Gang of Four book.
Master the art of serialization with the Proxy Pattern - pave the way to robust and efficient Java applications!