Jumpstart Your Java Microservices with Lagom: A Guide
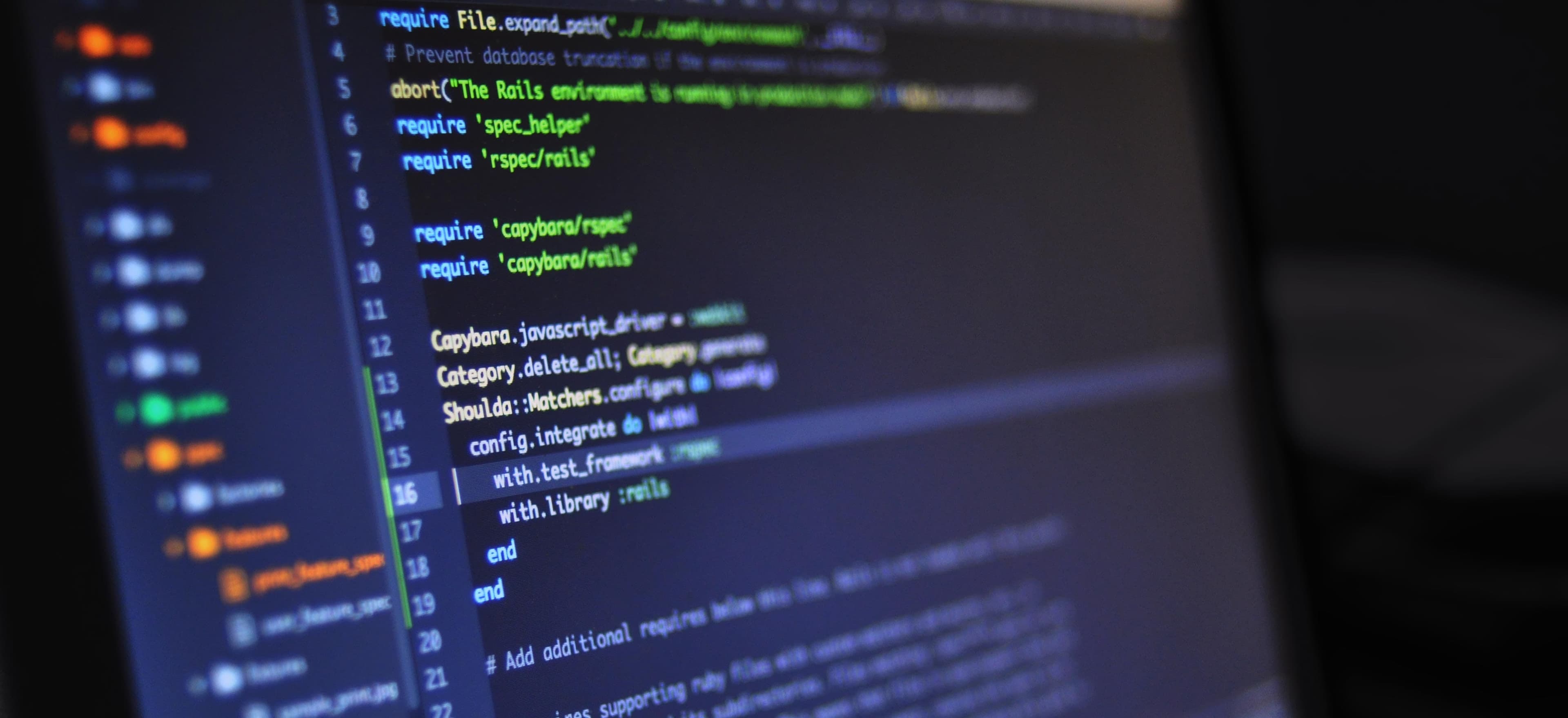
- Published on
Jumpstart Your Java Microservices with Lagom: A Guide
Are you ready to dive into the world of microservices in Java? Look no further than Lagom, a powerful framework that can help you build resilient and scalable systems. In this guide, we'll explore the fundamentals of Lagom and provide you with the insights you need to jumpstart your journey into Java microservices.
What is Lagom?
Lagom, a Swedish word meaning "just the right amount," is a modern microservices framework designed to help developers build reactive systems in Java and Scala. Developed by Lightbend, Lagom embodies the principles of Domain-Driven Design (DDD) and embraces the Actor Model and CQRS (Command Query Responsibility Segregation) patterns.
Why Lagom?
Lagom offers a holistic approach to microservices development by providing built-in support for event sourcing, CQRS, and asynchronous communication. It also comes with a development environment, built-in testing capabilities, and native integration with Akka and Play Framework.
Getting Started with Lagom
To start your journey with Lagom, you'll need to set up your development environment. Make sure you have Java Development Kit (JDK) 11 or newer installed on your machine.
Next, you can use sbt to create a new Lagom project. Here's a simple example of how to create a Lagom project using sbt:
sbt new lagom/lagom-scala.g8
Once your project is set up, you can start defining your Lagom services and entities. Lagom embraces the concept of service API contracts, which are defined using Akka gRPC. This provides a language-agnostic way to define service interfaces and data models.
Let's take a look at a basic Lagom service definition:
import com.lightbend.lagom.javadsl.api.*;
public interface HelloService extends Service {
ServiceCall<NotUsed, String> sayHello();
@Override
default Descriptor descriptor() {
return named("hello").withCalls(
pathCall("/api/hello", this::sayHello)
).withAutoAcl(true);
}
}
In this example, we define a simple service called HelloService
with a single method sayHello()
. The Descriptor
defines the service path and its corresponding method.
Building Reactive Systems with Lagom
Reactive programming lies at the heart of Lagom. Lagom embraces the principles of reactive systems, which are responsive, resilient, elastic, and message-driven. This means that Lagom applications are designed to handle a large number of concurrent users and provide consistent high performance.
Lagom leverages Akka under the hood to implement the Actor Model, providing a powerful and scalable foundation for building reactive systems. Additionally, Lagom's built-in support for event sourcing and CQRS enables developers to easily implement event-driven architectures.
Here's a snippet demonstrating event sourcing in Lagom:
@EventSourcedEntity(entityType = "hello")
public class HelloEntity extends PersistentEntity<HelloCommand, HelloEvent, HelloState> {
@Override
public Behavior initialBehavior(Optional<HelloState> snapshotState) {
BehaviorBuilder builder = newBehaviorBuilder(
snapshotState.orElse(HelloState.of("Hello"))
);
builder.setCommandHandler(UseGreetingMessage.class, (cmd, ctx) ->
ctx.thenPersist(new GreetingMessageChanged(cmd.message), evt ->
ctx.reply(Done.getInstance())
)
);
builder.setEventHandler(GreetingMessageChanged.class, evt ->
HelloState.of(evt.message)
);
return builder.build();
}
}
In this example, HelloEntity
represents an event-sourced entity that maintains its state through a series of events. The initialBehavior
method defines the entity's behavior, including command and event handlers.
Deploying Lagom Microservices
Once you have developed your Lagom microservices, you can deploy them to your preferred infrastructure. Lagom provides seamless integration with technologies like Lightbend ConductR, Kubernetes, and Docker, allowing you to easily package and deploy your microservices.
For example, you can use ConductR to manage and orchestrate your Lagom microservices as a distributed system. ConductR simplifies the deployment and scaling of Lagom services by providing features such as automatic service discovery, dynamic configuration, and resilience patterns.
Wrapping Up
Lagom offers a comprehensive toolkit for developing reactive microservices in Java, empowering developers to build scalable and resilient systems. By embracing the principles of reactive programming and event sourcing, Lagom simplifies the creation of distributed systems and enables seamless deployment to modern infrastructure platforms.
With the fundamentals and examples provided in this guide, you're now equipped to jumpstart your Java microservices journey with Lagom. Start building reactive, scalable, and resilient systems today!
Ready to explore Lagom further? Visit the official Lagom documentation for in-depth insights.
Checkout our other articles