Simplifying Access: Mastering Chained Delegation
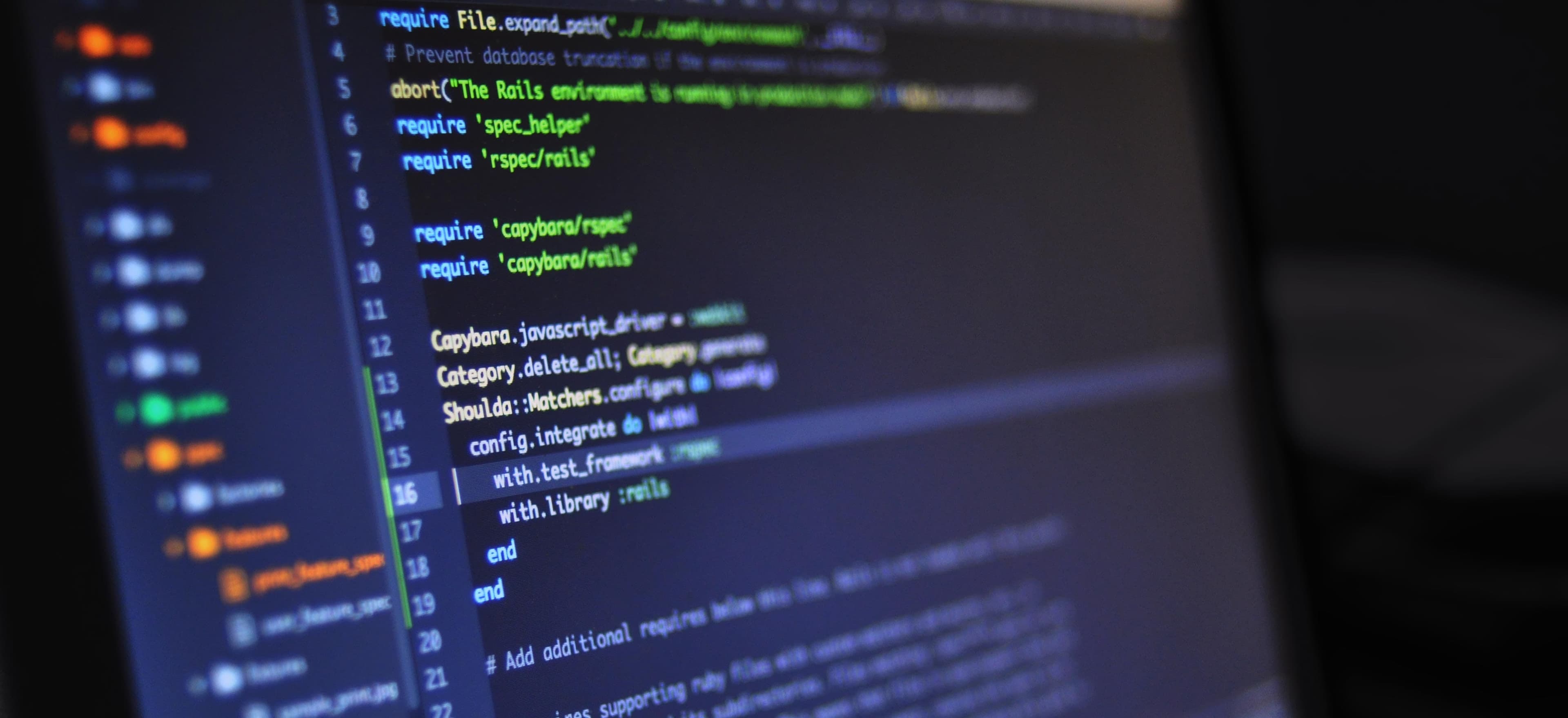
- Published on
Simplifying Access: Mastering Chained Delegation
In Java development, effectively managing access control and simplifying the delegation of responsibilities are crucial aspects of creating robust, maintainable, and secure applications. Chained delegation, a powerful design pattern, enables the streamlining of method calls and can significantly enhance the readability and maintainability of code.
Understanding Chained Delegation
Chained delegation, also known as method chaining, involves invoking multiple methods in a sequential, chained manner. This pattern allows for concise and fluent code construction, where the output of one method call seamlessly serves as the input for the next method invocation.
Consider the following example:
public class ChainedDelegationExample {
private int result;
public ChainedDelegationExample add(int number) {
result += number;
return this;
}
public ChainedDelegationExample subtract(int number) {
result -= number;
return this;
}
public int getResult() {
return result;
}
public static void main(String[] args) {
ChainedDelegationExample example = new ChainedDelegationExample();
int finalResult = example.add(5).subtract(3).getResult();
System.out.println("Final result: " + finalResult);
}
}
In this example, the add
and subtract
methods both return the current instance of the ChainedDelegationExample
class (this
), allowing for subsequent method calls in a chained fashion. This results in concise and expressive code, as the method calls can be fluently chained together, leading to improved readability and reduced verbosity.
Benefits of Chained Delegation
Improved Readability
Chained delegation allows for a more natural and understandable representation of sequential operations. By eliminating the need for temporary variables to store intermediate results, the code becomes more linear and easier to comprehend.
Fluent API Design
The method chaining pattern facilitates the creation of fluent APIs, where each method call closely resembles a natural language construct. This leads to more intuitive and user-friendly APIs, promoting a better developer experience.
Reduced Verbosity
Chained delegation streamlines the code by eliminating the repetitive references to the object on which the methods are invoked. This reduction in verbosity enhances the overall conciseness of the codebase.
Enhanced Method Call Sequencing
When employing chained delegation, the sequence of method calls becomes seamless, as the output of one method directly feeds into the input of the subsequent method. This leads to a more organized and interconnected method call structure, simplifying the overall flow of operations.
Implementing Chained Delegation with Fluent Interfaces
In addition to the basic chaining capability demonstrated earlier, chained delegation can be further enhanced through the use of fluent interfaces. Fluent interfaces are a specialized form of method chaining that focus on providing a highly readable, domain-specific API.
Let's illustrate the concept with a practical scenario:
public class FluentInterfaceExample {
private String query;
public FluentInterfaceExample select(String fields) {
query = "SELECT " + fields;
return this;
}
public FluentInterfaceExample from(String table) {
query += " FROM " + table;
return this;
}
public FluentInterfaceExample where(String condition) {
query += " WHERE " + condition;
return this;
}
public String build() {
return query + ";";
}
public static void main(String[] args) {
FluentInterfaceExample queryBuilder = new FluentInterfaceExample();
String sqlQuery = queryBuilder.select("id, name").from("users").where("age > 18").build();
System.out.println("Generated SQL query: " + sqlQuery);
}
}
In this example, the FluentInterfaceExample
class provides a fluent interface for constructing SQL queries. By applying method chaining, the sequence of select
, from
, and where
method calls succinctly form a complete SQL query.
Why Use Fluent Interfaces with Chained Delegation?
Fluent interfaces, when combined with chained delegation, result in code that closely resembles a DSL (Domain-Specific Language), making the code more expressive and easier to understand. This approach is particularly effective in scenarios where method calls often follow a specific sequence or syntax, such as query construction, configuration, or other domain-specific operations.
Best Practices for Chained Delegation
To make the most of chained delegation in Java, it is essential to adhere to certain best practices:
-
Consistent Return Types: Ensure that methods within the chain consistently return the appropriate object type to support further chaining. This promotes a seamless and predictable chaining experience.
-
Immutability: Consider leveraging immutability within the chain to prevent unintended side effects. Immutable objects ensure that each method call returns a new instance, maintaining the integrity of the original object.
-
Clear Documentation: As method chaining may alter the traditional flow of method calls, it is crucial to provide clear documentation and examples to guide developers in effectively utilizing the chained delegation pattern.
-
Avoid Chaining Overuse: While method chaining can greatly enhance code readability, excessive chaining should be avoided. Overuse of chaining may lead to overly complex and less maintainable code.
By following these best practices, developers can harness the full potential of chained delegation, fostering the creation of more intuitive and maintainable codebases.
In Conclusion, Here is What Matters
Chained delegation, when utilized appropriately, serves as a powerful mechanism for improving code clarity, streamlining method calls, and simplifying the management of responsibilities within Java applications. By understanding the principles of chained delegation and incorporating best practices, developers can unlock the full potential of this design pattern, contributing to the creation of more expressive, concise, and maintainable code.
Mastering chained delegation and fluent interfaces can greatly enhance the development experience, enabling the creation of elegant and intuitive APIs and codebases that resonate with both developers and end-users.
Embrace the elegance of chained delegation, and witness the transformation of your Java code into a fluent and expressive masterpiece.
Checkout our other articles