Unlocking Immutability: Mastering Value Types in Java
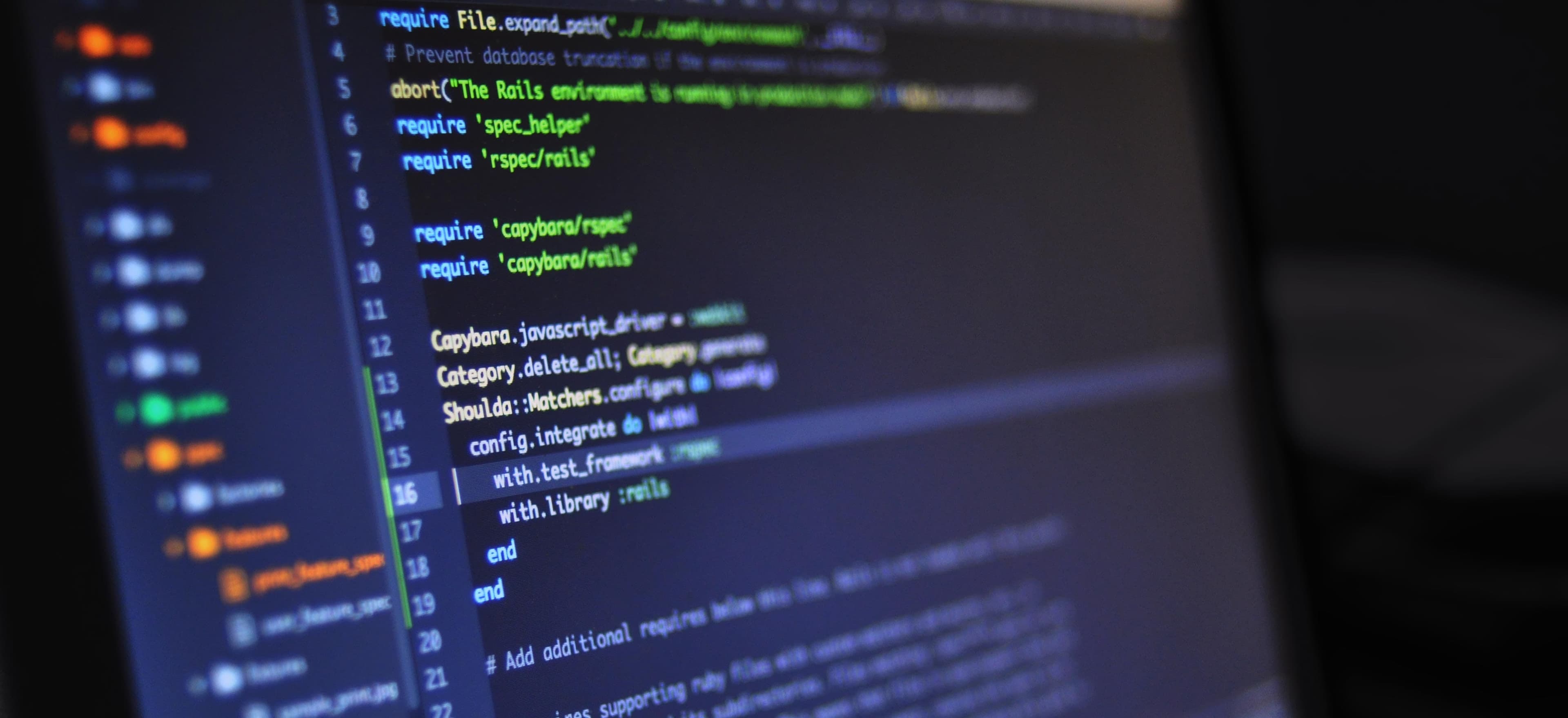
- Published on
Unlocking Immutability: Mastering Value Types in Java
Immutability is a crucial concept in Java programming, and it plays a vital role in creating robust, thread-safe, and efficient code. With the introduction of Project Valhalla in Java, developers now have the opportunity to explore value types, which aim to provide immutable, data-oriented types at a low level. In this blog post, we'll delve into the world of value types and understand how they can help us unlock immutability in Java.
Understanding Immutability
In Java, an immutable object is an object whose state cannot be modified after it is created. This means that once an immutable object is instantiated, its state remains constant throughout its lifetime. This concept brings several advantages, such as simplifying concurrent programming, allowing for safe sharing of objects across threads, and enhancing overall code predictability and reliability.
Creating an Immutable Class
Let's create an immutable class Person
to grasp the concept better:
public final class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In the above code, the Person
class is declared as final
, making it non-extendable. The member variables name
and age
are also marked as final
, and they are assigned values only through the constructor. The accessor methods getName()
and getAge()
provide read-only access to the internal state of the Person
objects.
By following these guidelines, we ensure that once a Person
object is created, its state cannot be altered, thus achieving immutability.
Diving Into the Subject to Value Types
Value types, as proposed in Project Valhalla, aim to provide immutable, data-oriented types at a low level. These types are intended to represent small, fixed-size, and immutable aggregates of data, while being free of identity. This means that instances of value types can be treated purely as values, allowing for efficient memory layout and optimized performance.
Declaring a Value Type
Let's declare a simple value type Point
to understand the basics:
public final class Point {
private final int x;
private final int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
In this example, the Point
class is declared as final
, and its fields x
and y
are marked as final
as well, ensuring immutability. This basic structure captures the essence of a value type, encapsulating a small, fixed-size, immutable data aggregate.
Benefits of Value Types
The introduction of value types brings compelling benefits to Java developers:
Efficient Memory Layout
By treating instances of value types as pure values, the JVM can employ more aggressive optimizations, including stack allocation, packed structures, and inlining.
Improved Performance
Value types allow for more efficient use of memory and CPU, resulting in improved performance for certain types of data.
Enhanced Predictability
The immutability of value types promotes code predictability and reliability, which are essential for building robust and maintainable systems.
Reduced Garbage Collection Overhead
With value types, the need for heap allocation is minimized, leading to reduced pressure on the garbage collector and potentially fewer pauses in the application.
Working with Value Types
To truly grasp the power of value types, it's essential to understand how they differ from reference types and how they can be leveraged in practice.
Value Types vs. Reference Types
In Java, most types are reference types, meaning that variables hold references to objects in memory. When it comes to value types, instances are embodied as actual values, allowing them to be stack-allocated, passed by value, and easily identified by their content.
Leveraging Value Types in Practice
Consider a scenario where a large number of Point
instances need to be created and manipulated. By utilizing value types, the memory overhead of objects and indirection through references can be minimized, leading to more efficient memory usage and potentially improved performance.
Embracing Immutability with Value Types
To showcase the synergy of immutability and value types, let's enhance our Point
class by leveraging the sealed
and non-sealed
modifiers introduced in Java 17.
Sealed and Non-Sealed Classes
public sealed non-sealed class Point permits ImmutablePoint {
private final int x;
private final int y;
public record ImmutablePoint(int x, int y) {}
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
In the above code, Point
is declared as a sealed non-sealed
class, allowing it to be extended only within the same compilation unit. The nested ImmutablePoint
record serves as an immutable variant of the Point
class, providing a convenient way to represent values.
By embracing the sealed
and non-sealed
modifiers and incorporating records, we not only enforce the immutability of ImmutablePoint
but also restrict the extensibility of Point
, thereby reinforcing the principles of immutability and value-oriented design.
Bringing It All Together
Value types, as a part of Project Valhalla, aim to revolutionize the way we model and manipulate data in Java. By embracing immutability and leveraging value types, developers can unlock new levels of performance, predictability, and efficiency in their code.
With a clear understanding of value types and their benefits, you are now equipped to explore their potential in your Java projects and harness the power of immutability for building robust and efficient systems.
Immutability and value types form the bedrock of a modern, data-centric Java ecosystem, enabling developers to write code that not only runs efficiently but is also easier to reason about and maintain.
So, embrace immutability, master value types, and embark on a journey to unlock the full potential of Java programming.
To dive deeper into the world of Java value types and immutability, be sure to explore the official Project Valhalla documentation and stay informed about the latest advancements in this exciting space.
Remember, immutability is not just a concept; it's a paradigm that can elevate your Java programming to new heights.
Checkout our other articles