Boosting Reliability: Overcoming Common Software Hurdles
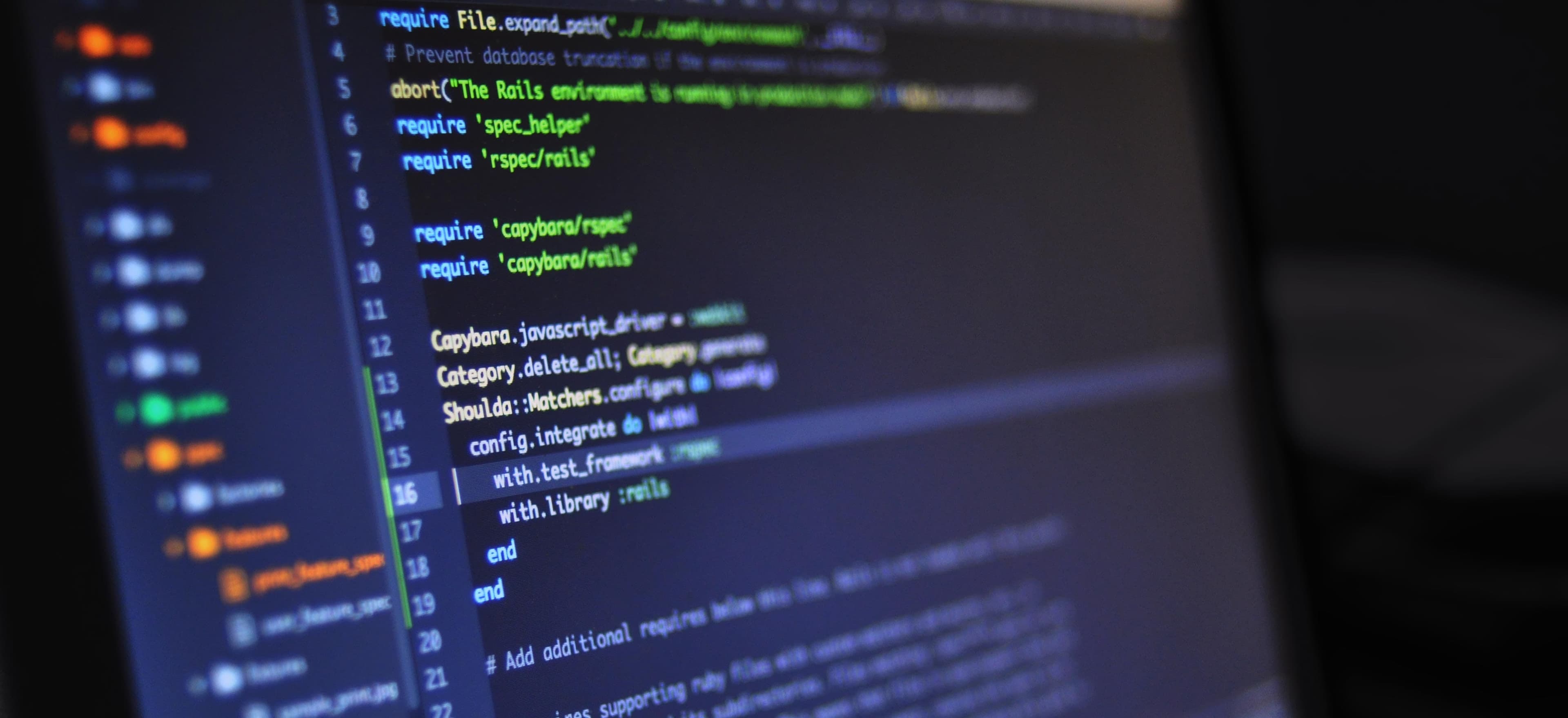
- Published on
Boosting Reliability: Overcoming Common Software Hurdles
In the fast-paced world of software development, reliability is key. Whether it's a small utility or a large enterprise application, ensuring that your software performs consistently and predictably is of utmost importance. This is where Java, with its robust features and tools, plays a critical role. Let's delve into some common hurdles in software reliability and explore how Java can help us overcome these challenges.
Exception Handling in Java
Exception handling is a fundamental aspect of building reliable software. Java provides a comprehensive mechanism for handling exceptions through try
, catch
, and finally
blocks. Here's a simple example:
try {
// Code that may throw an exception
int result = 10 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
// Handle the exception
System.out.println("An arithmetic exception occurred: " + e.getMessage());
} finally {
// This block is always executed, regardless of whether an exception is thrown
System.out.println("Cleanup code");
}
The above code demonstrates how Java's exception handling can prevent unexpected program termination and gracefully handle errors, thus improving reliability.
Effective Logging
Logging is indispensable for diagnosing issues and monitoring the behavior of a software system. Java offers a robust logging framework through its built-in java.util.logging
package. Here's a brief example:
import java.util.logging.*;
public class Example {
private static final Logger logger = Logger.getLogger(Example.class.getName());
public static void main(String[] args) {
logger.setLevel(Level.INFO);
logger.info("This is an informational message");
logger.warning("This is a warning message");
}
}
Using Java's logging capabilities enables developers to record valuable information for troubleshooting and performance analysis, thereby enhancing reliability.
Concurrency Management
Concurrency issues can significantly impact the reliability of a software system. Java provides powerful tools to manage concurrency, such as the synchronized
keyword and the java.util.concurrent
package. Consider the following synchronization example:
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
}
By utilizing Java's concurrency management features, developers can mitigate race conditions and ensure the consistent behavior of their applications, thereby improving reliability.
Comprehensive Testing with JUnit
Testing is paramount for ensuring the reliability of software. JUnit, a widely-used testing framework for Java, facilitates the creation and execution of comprehensive test suites. Here's a basic example:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class MyMathTest {
@Test
public void testAddition() {
assertEquals(5, MyMath.add(2, 3));
}
}
By incorporating JUnit into the development workflow, developers can systematically validate the correctness of their code, leading to more reliable software.
Memory Management
Effective memory management is critical for the reliability and performance of software applications. In Java, the automatic memory management provided by the garbage collector alleviates the burden of manual memory allocation and deallocation. This helps prevent memory leaks and segmentation faults, thereby enhancing the reliability of Java applications.
Exceptional Community Support
Java boasts a vast and active community of developers, which translates to a wealth of resources, forums, and expertise. Leveraging this community support can be invaluable when troubleshooting issues, improving code quality, and staying updated with best practices, thus contributing to overall software reliability.
By harnessing the power of Java's features and leveraging its extensive ecosystem, developers can effectively overcome common software hurdles, ultimately boosting the reliability of their applications.
In conclusion, prioritizing reliability in software development is non-negotiable. With Java's exceptional toolset and community support, developers are well-equipped to address and surmount common challenges, ensuring that their software systems perform consistently and predictably.
Checkout our other articles