Solving Callback Hell: Promises in jQuery and AngularJS
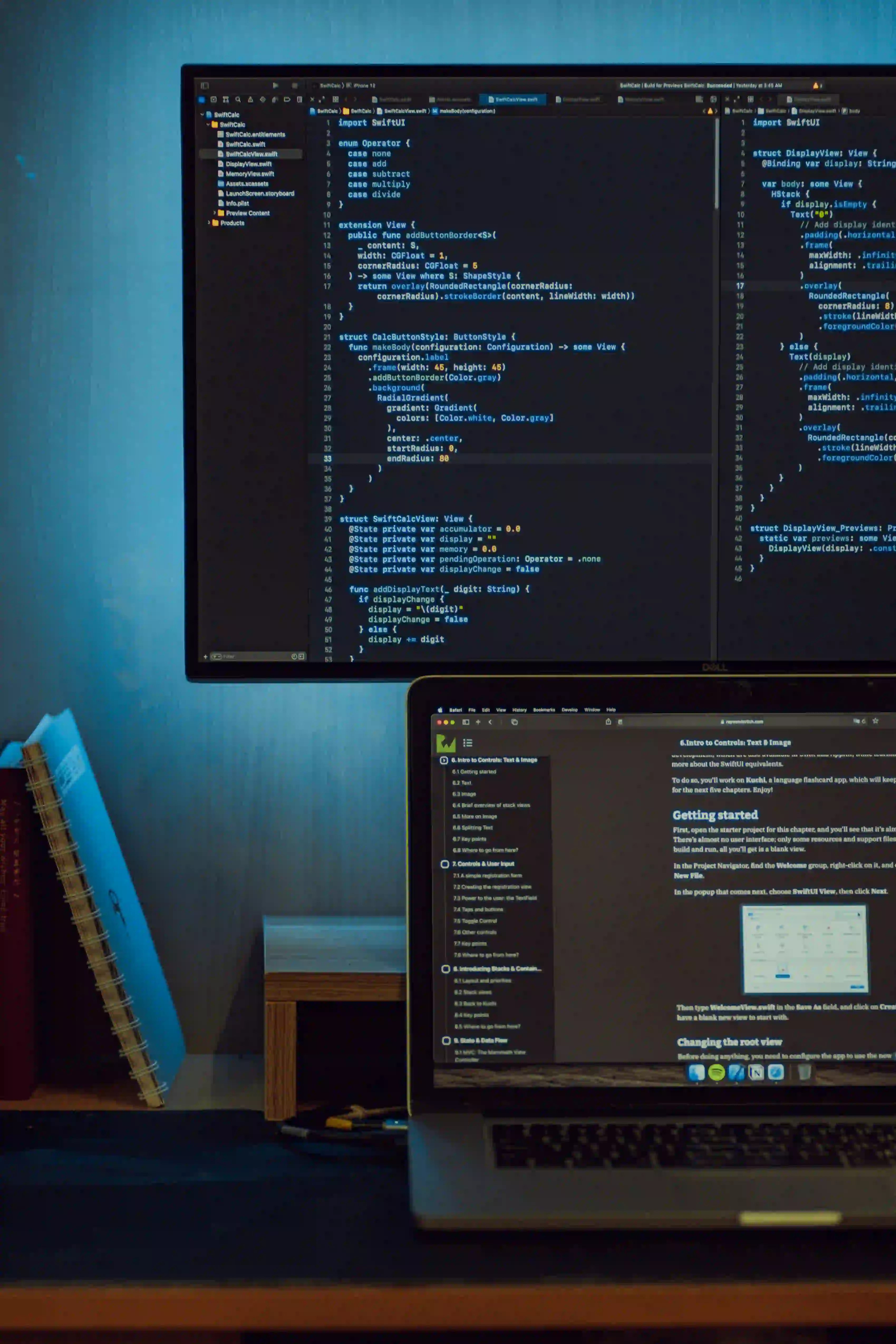
Solving Callback Hell: Promises in jQuery and AngularJS
Callback hell, also known as the Pyramid of Doom, refers to the situation when a sequence of asynchronous operations leads to deeply nested callback functions, resulting in code that is hard to read, understand, and maintain. It's a common issue when working with JavaScript, especially when dealing with AJAX requests, timeouts, and event handling. However, there's a powerful solution: Promises.
Understanding Callback Hell
Before we delve into promises, let's take a moment to understand callback hell. Consider the following example of nested callbacks in jQuery:
$.ajax({
url: "/data",
success: function(data) {
$.ajax({
url: "/data/processed",
success: function(processedData) {
// Process processedData
},
error: function() {
// Handle error
}
});
},
error: function() {
// Handle error
}
});
As you can see, the code quickly becomes unwieldy and difficult to follow as more logic is added inside the callbacks. This leads to maintenance nightmares and reduces code readability.
Enter Promises
Promises provide a cleaner alternative to handling asynchronous operations. They simplify the chaining of asynchronous tasks and allow for more readable and maintainable code. Both jQuery and AngularJS have adopted the promise pattern to handle asynchronous operations.
Promises in jQuery
In jQuery, the $.ajax
method returns a promise, which allows us to chain .then
and .catch
methods for handling success and error conditions, respectively.
$.ajax({
url: "/data"
}).then(function(data) {
return $.ajax({
url: "/data/processed"
});
}).then(function(processedData) {
// Process processedData
}).catch(function(error) {
// Handle error
});
In this example, the code flows sequentially, making it easier to understand the logic and handle errors uniformly.
Promises in AngularJS
AngularJS also provides its own implementation of promises through the $q
service, which allows for similar chaining of asynchronous operations.
$http.get("/data")
.then(function(response) {
return $http.get("/data/processed");
})
.then(function(response) {
// Process response.data
})
.catch(function(error) {
// Handle error
});
The use of promises in AngularJS follows a similar pattern to jQuery, allowing for a consistent approach to dealing with asynchronous tasks.
Wrapping Callback-Based APIs with Promises
Many libraries and APIs still use the traditional callback style. Fortunately, it's possible to wrap these APIs with promises to take advantage of the benefits they offer.
Wrapping a Callback-Based API with Promises in JavaScript
Let's consider an example of wrapping the Node.js fs.readFile
function, which uses callbacks, with promises using the util.promisify
function from Node.js's built-in util
module.
const util = require('util');
const fs = require('fs');
const readFileAsync = util.promisify(fs.readFile);
readFileAsync('example.txt', 'utf8')
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
By wrapping the callback-based fs.readFile
function with a promise, we can now use it in a more streamlined and readable manner.
Wrapping a Callback-Based API with Promises in AngularJS
In AngularJS, we can create a service that wraps an existing callback-based API with promises. Let's create a simple example using the $q
service to wrap the setTimeout
function.
app.service('customService', function($q) {
this.customTimeout = function(ms) {
var deferred = $q.defer();
setTimeout(function() {
deferred.resolve('Finished after ' + ms + 'ms');
}, ms);
return deferred.promise;
};
});
// Later, in a controller
customService.customTimeout(1000)
.then(function(result) {
console.log(result);
});
This approach allows us to leverage promises in scenarios where the existing API uses callbacks, improving code readability and maintainability.
A Final Look
Promises provide a powerful tool for managing asynchronous tasks in both jQuery and AngularJS. By avoiding callback hell, code becomes more readable, maintainable, and less prone to errors. Additionally, when working with callback-based APIs, the ability to wrap them with promises enables a consistent approach to asynchronous programming. Embracing promises is a valuable step towards writing clean and reliable JavaScript code.
By mastering promises, developers can elevate the quality of their code, streamline asynchronous operations, and ultimately, provide a better user experience.
Now that you've grasped the concept of promises, take a deeper dive into their intricacies and nuances, and explore how they can further enhance your JavaScript development efforts.