JDBC Hack: Emulating Sequences in Unsupported Databases!
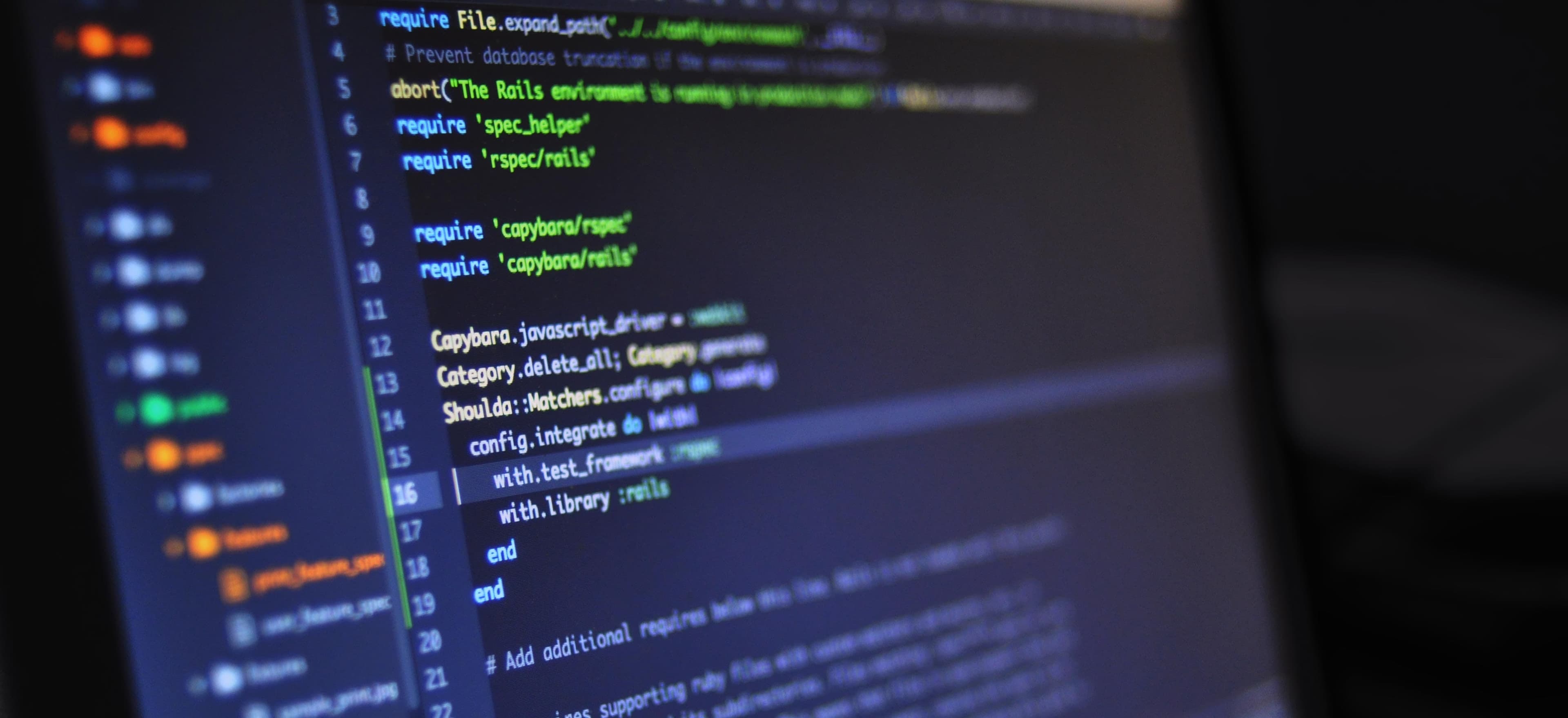
- Published on
Emulating Sequences in Unsupported Databases with JDBC
In the world of Java development, Java Database Connectivity (JDBC) plays a crucial role in connecting Java applications to databases. Although JDBC serves as a standard interface to interact with various databases, there are still differences in the features supported by different database systems.
One such feature that is not universally supported across all databases is sequences (or the equivalent of auto-increment columns). Sequences are commonly used to generate unique identifiers for database records, and they are well-supported in databases like PostgreSQL and Oracle.
However, some databases, such as MySQL and SQL Server, do not have built-in support for sequences. In such cases, developers often have to find alternative solutions to emulate sequence-like behavior in these databases.
In this blog post, we'll explore how to emulate sequences in databases that do not natively support them using JDBC and discuss why this approach can be beneficial.
Emulating Sequences: The Challenge
Before we dive into the solution, let's understand the challenge at hand. When working with databases that do not have native support for sequences, we need to find a way to create a similar mechanism using the available features provided by the database.
In databases like MySQL, we typically use auto-increment columns to achieve similar functionality to sequences. However, in more complex scenarios, such as when we need to generate sequence values outside the scope of regular INSERT operations, emulating sequences becomes a more intricate task.
This is where a deep understanding of JDBC and database-specific behaviors comes into play. By leveraging the power of JDBC, we can devise a solution to emulate sequences seamlessly, regardless of the underlying database system.
Leveraging JDBC for Sequence Emulation
JDBC provides a uniform interface for accessing different types of databases, allowing us to write database-agnostic code. When it comes to emulating sequences in databases that lack native support, JDBC empowers us to develop custom solutions that align with the database's capabilities.
To illustrate this, let's consider a scenario where we need to generate sequence-like values for a table in a database that does not support sequences, such as MySQL.
We can achieve this by creating a separate table to maintain the sequence values and using JDBC to interact with this table to obtain and update the sequence values as needed.
Implementing Sequence Emulation with JDBC
Below is a simplified example of how we can emulate sequences in a MySQL database using JDBC. This example demonstrates the essential components of sequence emulation, including the creation of a sequence table and the logic to retrieve and update sequence values.
Creating the Sequence Table
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) {
String createSequenceTableQuery = "CREATE TABLE IF NOT EXISTS sequences ("
+ " sequence_name VARCHAR(50) NOT NULL PRIMARY KEY,"
+ " sequence_value BIGINT NOT NULL"
+ ")";
try (Statement statement = connection.createStatement()) {
statement.execute(createSequenceTableQuery);
}
} catch (SQLException e) {
// Handle any SQL exceptions
e.printStackTrace();
}
In this snippet, we use JDBC to create a table called sequences
if it does not already exist. This table will store the sequence names and their corresponding values.
Retrieving Sequence Values
public long getNextSequenceValue(String sequenceName) throws SQLException {
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) {
String selectSequenceValueQuery = "SELECT sequence_value FROM sequences WHERE sequence_name = ?";
try (PreparedStatement preparedStatement = connection.prepareStatement(selectSequenceValueQuery)) {
preparedStatement.setString(1, sequenceName);
try (ResultSet resultSet = preparedStatement.executeQuery()) {
if (resultSet.next()) {
long sequenceValue = resultSet.getLong("sequence_value");
return sequenceValue + 1;
} else {
// Handle sequence not found
return 1;
}
}
}
}
}
In this snippet, we define a method to retrieve the next sequence value for a given sequence name. We use a prepared statement to execute a SELECT query and obtain the current sequence value from the sequences
table.
Updating Sequence Values
public void updateSequenceValue(String sequenceName, long newValue) throws SQLException {
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password")) {
String updateSequenceValueQuery = "REPLACE INTO sequences (sequence_name, sequence_value) VALUES (?, ?)";
try (PreparedStatement preparedStatement = connection.prepareStatement(updateSequenceValueQuery)) {
preparedStatement.setString(1, sequenceName);
preparedStatement.setLong(2, newValue);
preparedStatement.executeUpdate();
}
}
}
In this snippet, we define a method to update the sequence value for a given sequence name. We use a REPLACE INTO
statement to update the sequence value in the sequences
table. The REPLACE
statement ensures that if a sequence with the given name does not exist, it will be inserted, and if it does exist, its value will be updated.
In Conclusion, Here is What Matters
Emulating sequences in databases that do not natively support them can be achieved effectively using JDBC. By leveraging JDBC's capabilities to interact with the database and implementing custom logic, developers can seamlessly emulate sequence behavior regardless of the underlying database system.
Understanding the nuances of JDBC and the specific features of the target database is crucial for creating robust and efficient sequence emulation solutions. With the right approach and a solid understanding of JDBC, developers can overcome the limitations of unsupported databases and implement reliable sequence emulation mechanisms for their applications.
In conclusion, while databases may vary in their feature support, JDBC provides a level of consistency and flexibility that enables developers to implement creative solutions, such as sequence emulation, to meet the requirements of diverse database environments.
By combining JDBC's power with a deep understanding of database behaviors, developers can navigate the intricacies of working with different databases and deliver robust, database-agnostic solutions. Emulating sequences in unsupported databases is just one example of how JDBC empowers developers to bridge the gaps between varying database capabilities and deliver consistent functionality across different database systems.
So, the next time you encounter a database that lacks native sequence support, remember that JDBC offers the tools and flexibility to overcome such limitations, allowing you to implement elegant, database-agnostic solutions with ease.