Overcoming Scalability Hurdles with Play Framework
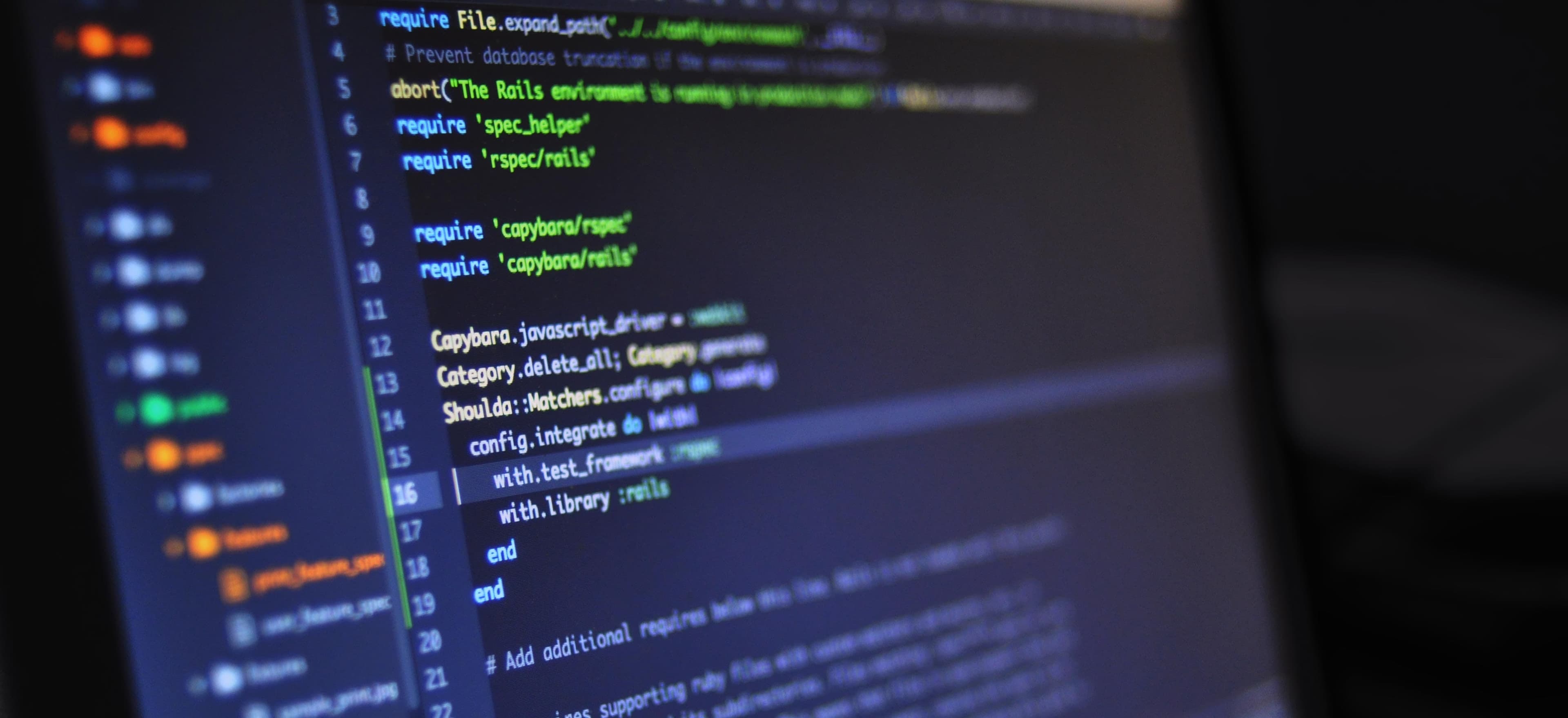
- Published on
Overcoming Scalability Hurdles with Play Framework
In today's fast-paced and ever-evolving digital landscape, the ability to scale a software solution is an essential factor for its success. When it comes to building highly scalable and responsive web applications, Play Framework emerges as a robust choice for Java developers. Play Framework, with its non-blocking I/O and state-of-the-art Akka toolkit, provides a powerful foundation for building scalable web applications.
In this article, we will delve into the concept of scalability and explore how Play Framework can be leveraged to overcome scalability hurdles in Java web development.
Understanding Scalability in Web Applications
Scalability, in the context of web applications, refers to the capability of a system to handle growing amounts of work in a graceful and efficient manner. This growth can be in the form of increased user traffic, higher data volumes, or more complex computations.
There are two primary types of scalability: horizontal scalability and vertical scalability. Horizontal scalability involves adding more machines to distribute the load, while vertical scalability focuses on adding more resources (CPU, memory, etc.) to a single machine.
Challenges with Traditional Approaches
Traditional web application frameworks often face scalability challenges due to their blocking I/O model. When a request is made to the server, the thread handling the request is blocked until the operation is completed, leading to resource underutilization and diminished responsiveness under high load.
Enter Play Framework
Play Framework, with its underlying architectural principles, addresses the scalability challenges faced by traditional web application frameworks. It is built on an asynchronous, non-blocking model, allowing it to efficiently handle a large number of concurrent connections without exhausting system resources.
Actor-Based Concurrency with Akka
At the heart of Play Framework is Akka, a powerful toolkit for building concurrent, distributed, and resilient applications. Akka's actor-based model allows for efficient management of application state and seamless scalability across multiple cores and even multiple machines.
Let's take a look at a simple example of how actor-based concurrency in Akka can enhance scalability:
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
public class SimpleActorExample {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("actorSystem");
// Define the actor
class SimpleActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, message -> {
System.out.println("Received message: " + message);
})
.build();
}
}
// Create the actor
ActorRef simpleActor = system.actorOf(Props.create(SimpleActor.class), "simpleActor");
// Send a message to the actor
simpleActor.tell("Hello, Akka!", ActorRef.noSender());
}
}
In this example, we create a simple actor that can asynchronously handle messages. This actor-based concurrency model enables efficient utilization of resources and seamless scaling of concurrent operations.
Non-Blocking I/O
Play Framework's use of non-blocking I/O further enhances its scalability. By leveraging asynchronous operations, Play Framework can handle a large number of concurrent requests without being limited by the number of threads available in the system.
public Result asyncOperation() {
CompletableFuture<Result> futureResult = CompletableFuture.supplyAsync(() -> {
// Perform asynchronous operation
return ok("Operation complete");
}, httpExecutionContext.current()); // Use the built-in execution context
return async(() -> futureResult);
}
In this snippet, the asyncOperation
method returns a Result
asynchronously. This allows the server to handle other requests while the asynchronous operation is in progress, thus improving overall responsiveness and scalability.
Leveraging Reactive Programming
Another key aspect of Play Framework's approach to scalability is its support for reactive programming. Reactive programming enables the development of resilient, responsive, and elastic applications that can easily scale to meet growing demands.
Play Framework's integration with libraries such as ReactiveMongoDB and ReactiveStreams allows developers to build highly responsive and scalable applications that can efficiently handle complex data processing and streaming operations.
Achieving Horizontal Scalability
With Play Framework's support for stateless, non-blocking, and asynchronous operations, achieving horizontal scalability becomes a feasible goal. By adding more instances of the application and distributing the load across them, developers can seamlessly scale their applications to meet increasing demands.
In Conclusion, Here is What Matters
Play Framework's unique blend of non-blocking I/O, actor-based concurrency with Akka, and support for reactive programming makes it a compelling choice for overcoming scalability hurdles in Java web development. By embracing the principles of scalability from the ground up, Play Framework empowers developers to build web applications that can effortlessly scale to meet the demands of modern digital environments.
In conclusion, by incorporating Play Framework into your Java web development arsenal, you can effectively tackle the challenges of scalability and position your applications for success in an increasingly dynamic and competitive landscape.
To delve deeper into the world of Play Framework and Scala, check out Play Framework's official documentation and Akka Toolkit documentation. These resources provide comprehensive insights into leveraging the full potential of Play Framework and Akka for building scalable web applications.