Enhancing Your JAX-RS Services for HTTP/2: A Vital Shift
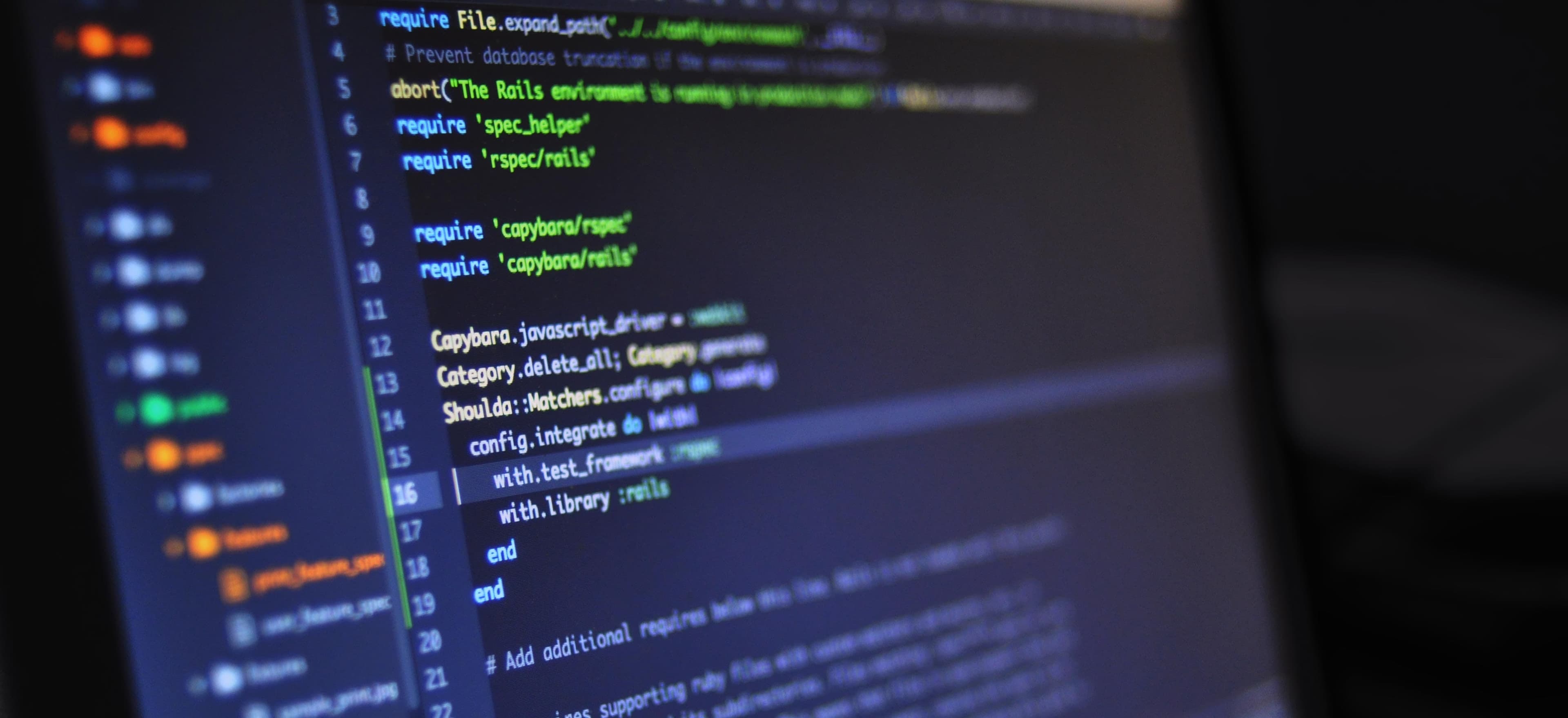
- Published on
Enhancing Your JAX-RS Services for HTTP/2: A Vital Shift
In the ever-evolving world of web development, the introduction of HTTP/2 has brought about significant improvements in website performance and user experience. With its multiplexing capabilities, server push, and header compression, HTTP/2 offers an array of benefits over its predecessor, HTTP/1.1. While its adoption has been widespread, its integration with JAX-RS services in Java applications is a crucial step towards optimizing web service performance.
In this post, we'll delve into the key aspects of enhancing your JAX-RS services for HTTP/2, the benefits it brings, and the steps to implement it effectively.
Understanding the Significance of HTTP/2 for JAX-RS Services
HTTP/2 introduces several features that improve web performance, such as:
- Multiplexing: This feature enables multiple requests and responses to be sent in parallel over a single TCP connection, eliminating the need for multiple connections.
- Server Push: HTTP/2 allows the server to push responses to the client's cache without waiting for a new request, thereby reducing latency.
- Header Compression: Headers are compressed, reducing overhead and resulting in faster data exchange.
By leveraging these capabilities, JAX-RS services can provide enhanced performance, quicker loading times, and a more efficient utilization of network resources.
Implementing HTTP/2 in Your JAX-RS Services
To leverage the benefits of HTTP/2 in your JAX-RS services, you need to ensure that your server, clients, and application infrastructure support this protocol.
Server-Side Implementation
1. Update Your Servlet Container
You'll need to ensure that your chosen servlet container, such as Tomcat or Jetty, supports HTTP/2. Configuration settings and dependencies may vary, so it's essential to refer to the specific documentation for your selected container.
2. Enable HTTP/2 Support
Once your servlet container is updated, enable HTTP/2 support in the configuration. For instance, in Tomcat, you may need to update the <Connector>
element in the server.xml
file to include the upgradeProtocol
attribute with the value "h2c"
.
Client-Side Implementation
Ensure that the clients consuming your JAX-RS services also support HTTP/2. Most modern web browsers already provide HTTP/2 support, but it's crucial to verify if your specific client applications, libraries, or frameworks are compatible.
Application-Level Implementation
1. Use HTTPS
HTTP/2 mandates the use of HTTPS, so ensure that your JAX-RS services are accessible over HTTPS. This may require obtaining and configuring an SSL certificate for your server.
2. Update Dependencies
Ensure that your JAX-RS implementation and associated libraries are compatible with HTTP/2. Upgrading to the latest versions of frameworks such as Jersey or RESTEasy may be necessary to harness HTTP/2 benefits seamlessly.
By following these implementation steps, you can effectively gear your JAX-RS services to take advantage of the performance enhancements offered by HTTP/2.
Leveraging HTTP/2 Features in JAX-RS Services
Once HTTP/2 is integrated into your JAX-RS services, you can leverage its features to optimize the performance and responsiveness of your web applications.
Multiplexing Requests
With HTTP/2, multiple requests can be sent concurrently over a single connection, eliminating the bottleneck caused by multiple TCP connections in HTTP/1.1. As a result, JAX-RS services can efficiently handle a higher volume of requests, enhancing throughput and reducing latency.
Example:
@GET
@Path("/multiplexingExample")
public Response multiplexingExample() {
// Method implementation
}
Server Push
HTTP/2 allows servers to push related resources to clients before they are requested. This can be particularly advantageous for JAX-RS services that deliver web pages with associated resources such as CSS, JavaScript, and images.
Example:
@GET
@Path("/serverPushExample")
public void serverPushExample(@Suspended AsyncResponse asyncResponse) {
// Implementation to push related resources
}
Header Compression
HTTP/2's header compression reduces overhead, leading to faster data exchange between clients and JAX-RS services. This is especially beneficial when handling RESTful API calls with extensive header information.
Example:
@GET
@Path("/headerCompressionExample")
public Response headerCompressionExample() {
// Method implementation
}
By incorporating these features, JAX-RS services can deliver optimized responses, reduced latencies, and an overall improved user experience.
In Conclusion, Here is What Matters
The shift towards HTTP/2 presents a vital opportunity for enhancing the performance of JAX-RS services in Java applications. By embracing HTTP/2's multiplexing, server push, and header compression, developers can significantly improve the efficiency and responsiveness of their web services.
As web technology continues to advance, staying abreast of such pivotal shifts is crucial for delivering modern, high-performance web applications. Embracing HTTP/2 in your JAX-RS services is a decisive step towards achieving this goal.
Incorporating HTTP/2 support across your JAX-RS services empowers you to harness the full potential of this protocol, ensuring that your web applications remain competitive and responsive in an ever-evolving digital landscape.
By following the outlined steps and leveraging HTTP/2 features, you can propel your JAX-RS services to new heights of performance and user satisfaction, solidifying your position at the forefront of the digital realm.
Make the pivotal leap to HTTP/2 for your JAX-RS services today, and revolutionize the way your Java applications interact with the web.
With HTTP/2, the future of JAX-RS services is now within your grasp.
This blog post was brought to you by TechWorld - your go-to source for the latest tech insights and innovations.
Explore the benefits of HTTP/2 and revolutionize your JAX-RS services with Jetty HTTP/2.