Escaping Callback Hell: Embracing Reactive Patterns
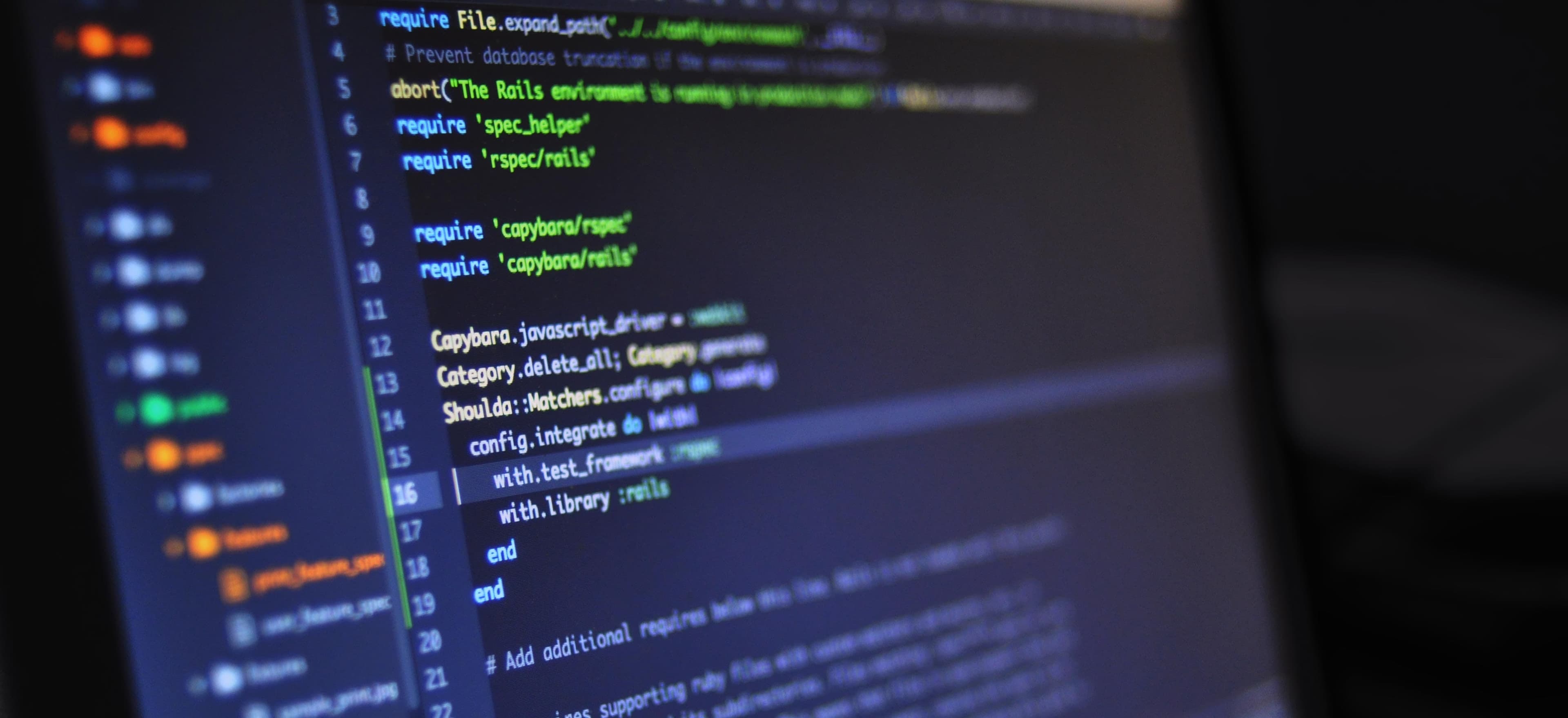
- Published on
Escaping Callback Hell: Embracing Reactive Patterns
If you've been developing applications in Java for some time, you are no stranger to the infamous "Callback Hell." Managing asynchronous operations in Java traditionally involved nesting callbacks within callbacks, leading to deeply nested and hard-to-read code. Fortunately, reactive programming offers a way out of this predicament. In this post, we'll explore how to escape the callback hell by embracing reactive patterns in Java.
Understanding Callback Hell
Callback hell, also known as the "pyramid of doom," occurs when multiple asynchronous operations are nested within one another. In Java, this is often seen in scenarios involving callbacks, such as handling HTTP requests, file I/O, or database interactions.
userService.getUser(userId, user -> {
userActivityService.getUserActivity(user.getId(), activities -> {
for (Activity activity : activities) {
// Process activity
}
});
});
Asynchronous operations are crucial for building responsive and efficient applications. However, managing the flow of asynchronous code in a readable and maintainable way can be a significant challenge.
Introducing Reactive Programming
Reactive programming is an approach to asynchronous programming that focuses on data streams and the propagation of changes. It provides a set of tools and patterns to manage and compose asynchronous event-driven code.
In the Java ecosystem, Project Reactor and its core library, Reactor, provide a rich set of features for reactive programming. Reactor implements the Reactive Streams specification, which standardizes the exchange of asynchronous stream data with non-blocking backpressure.
Escaping Callback Hell with Reactor
Let's take a look at how we can rewrite the previous example using Reactor to escape the callback hell.
userService.getUser(userId)
.flatMap(user -> userActivityService.getUserActivity(user.getId()))
.flatMapMany(Flux::fromIterable)
.subscribe(activity -> {
// Process activity
});
In this reactive code snippet, flatMap
is used to chain asynchronous operations, allowing for a more concise and readable flow. The flatMapMany
operator is used to handle the conversion from a mono (single item) to a flux (potentially multiple items).
Embracing Reactive Patterns for Asynchronous Workflows
Reactor provides a variety of operators and tools to manage asynchronous workflows seamlessly. Let's discuss some key concepts and patterns to embrace when working with reactive programming in Java.
1. Flux and Mono
In Reactor, Flux
represents a stream of 0 to N elements, while Mono
represents a stream with exactly 0 or 1 element. Understanding and leveraging these types is crucial for working effectively with reactive streams.
2. Operators
Reactor offers a wide range of operators to manipulate and control the flow of data within reactive streams. Operators such as map
, flatMap
, filter
, and reduce
allow for powerful transformations and compositions of asynchronous data.
3. Error Handling
Dealing with errors in asynchronous code is essential. Reactor provides operators like onErrorResume
, onErrorReturn
, and retry
to handle errors in a reactive and composable manner.
4. Backpressure
Reactive Streams specification addresses the issue of backpressure, where a fast data producer overwhelms a slow consumer. Reactor handles backpressure transparently, ensuring that data is consumed at a pace that the subscriber can handle.
5. Schedulers
Schedulers allow you to control the threading and execution context of reactive code. Reactor provides schedulers for common use cases, such as parallel execution, I/O-bound work, and more.
Wrapping Up
Reactive programming with Reactor offers a compelling solution to escape the callback hell and build more maintainable, composable, and efficient asynchronous code in Java. By embracing reactive patterns and leveraging the power of Reactor, developers can transform complex asynchronous workflows into clear and manageable code.
In conclusion, the shift from traditional callback-based asynchronous code to reactive programming brings a paradigm shift in how developers handle asynchronous workflows. It not only simplifies the code but also lays the foundation for building robust and responsive applications in the modern era of Java development.
Start embracing reactive programming today and bid farewell to the callback hell for good!
Remember, "Reactive programming is not about writing things that are reactive all the time; it is about having control over reactivity when needed." - Rossen Stoyanchev
So, go ahead, dive into reactive programming, and unleash the true potential of asynchronous workflows in Java!