Java Lambdas vs Auto-Closeable: Where to Draw the Line?
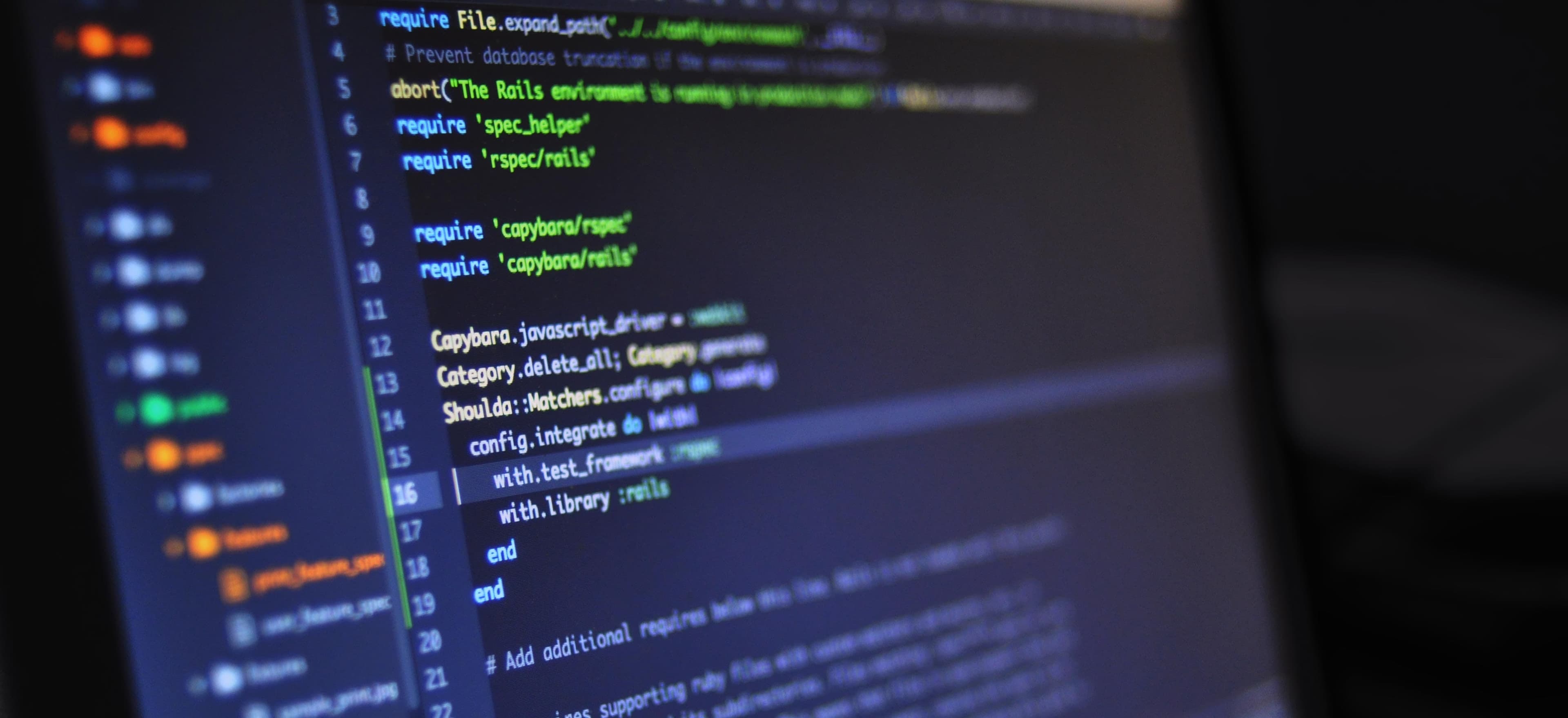
- Published on
Java Lambdas vs Auto-Closeable: Where to Draw the Line?
When it comes to modern Java development, the use of Lambdas and Auto-Closeable resources has become increasingly prevalent. Both features have significantly improved the way Java developers write concise and efficient code. However, there are occasions where the use of these features may conflict, prompting the need to draw a clear line between them.
In this article, we will explore the nuances of Java Lambdas and Auto-Closeable resources, their respective use cases, and how to effectively manage their coexistence.
Understanding Lambdas and Auto-Closeable Resources
Java Lambdas: Brief Overview
Lambdas, introduced in Java 8, provide a concise way to express a piece of functionality. They are essentially a mechanism for representing an anonymous function. One of the primary uses of Lambdas is in functional interfaces, where they can be used to pass behavior around your application.
Consider the following example, where a Lambda is used to define a simple addition operation:
// Lambda definition for addition
MathOperation addition = (int a, int b) -> a + b;
Auto-Closeable Resources: Introduction
Auto-Closeable is an interface introduced in Java 7 that facilitates automatic resource management. It is commonly used with try-with-resources statements to ensure that resources are closed after being used, without the need for explicit close() method calls.
Here's a basic example of using Auto-Closeable with a file resource:
try (FileReader reader = new FileReader("file.txt")) {
// Read from the file
} catch (IOException e) {
// Handle exception
}
The Clash: Lambdas and Auto-Closeable Resources
The Issue at Hand
The conflict arises when lambdas are used in the context of Auto-Closeable resources. Since lambdas are essentially anonymous functions, they do not throw checked exceptions. On the other hand, Auto-Closeable resources may throw IOException or other checked exceptions. This mismatch can lead to a potential problem if not handled properly.
Consider the following code snippet that attempts to use a Lambda within a try-with-resources block:
try (ResourceType resource = acquireResource()) {
// Use the resource with a lambda
resource.doSomething(() -> {
// Lambda logic
});
} catch (IOException e) {
// Handle exception
}
In this example, the lambda expression used inside the doSomething method does not declare any checked exceptions, potentially leading to a compilation error.
Deeper Analysis: Why the Conflict?
The conflict arises due to the fact that Lambdas are not allowed to throw checked exceptions that are not caught or declared in the functional interface's abstract method. This is a design decision to keep Lambda expressions concise and to avoid the clutter of handling checked exceptions within the Lambda body.
Auto-Closeable resources, on the other hand, often involve I/O operations, which can throw checked exceptions. When using lambdas within the scope of Auto-Closeable resources, the potential for checked exceptions becomes a crucial consideration.
Finding the Balance: Best Practices
Handling Checked Exceptions
To address the conflict between Lambdas and Auto-Closeable resources, it is crucial to handle checked exceptions properly. One approach is to catch and handle the exceptions within the Lambda expression to avoid propagating the checked exceptions out of the Lambda.
Consider the following modified code snippet where the IOException is handled within the Lambda:
try (ResourceType resource = acquireResource()) {
resource.doSomething(() -> {
try {
// Lambda logic which potentially throws IOException
} catch (IOException e) {
// Handle the exception
}
});
} catch (IOException e) {
// Handle exception
}
In this modified version, the IOException is caught and handled within the Lambda, preventing it from being propagated outside.
Utilizing Functional Interfaces
Another approach to circumvent the issue is to use functional interfaces that declare the checked exceptions thrown by the Lambda expressions. By defining custom functional interfaces that declare the checked exceptions, the issue of mismatch between Lambdas and Auto-Closeable resources can be effectively mitigated.
@FunctionalInterface
public interface CheckedConsumer<T, E extends Exception> {
void accept(T t) throws E;
}
By using custom functional interfaces that declare the checked exceptions, it becomes possible to handle exceptions within the lambda expressions effectively without resorting to suppressing or wrapping them.
Striking a Balance
While handling checked exceptions and using custom functional interfaces can alleviate the conflict, it's essential to strike a balance and avoid excessive handling or suppression of exceptions within Lambdas. Overly complex exception handling within Lambdas can diminish their readability and conciseness, which is one of their primary benefits.
Bringing It All Together
In conclusion, while Java Lambdas and Auto-Closeable resources have greatly improved code expressiveness and resource management, there are challenges that arise when using them in conjunction. By understanding the clash between Lambdas and Auto-Closeable resources, and adopting best practices for handling checked exceptions and utilizing custom functional interfaces, developers can effectively manage their coexistence.
Drawing the line between Lambdas and Auto-Closeable resources involves finding a delicate balance that ensures concise, readable, and exception-safe code. By addressing these challenges, developers can harness the full power of both features without sacrificing code quality or maintainability.
In summary, when it comes to handling Lambdas and Auto-Closeable resources, it's not about choosing one over the other, but rather about finding the right approach to harmoniously integrate them in your Java applications.
Checkout our other articles