Understanding JAXB Root Elements: Common Pitfalls to Avoid
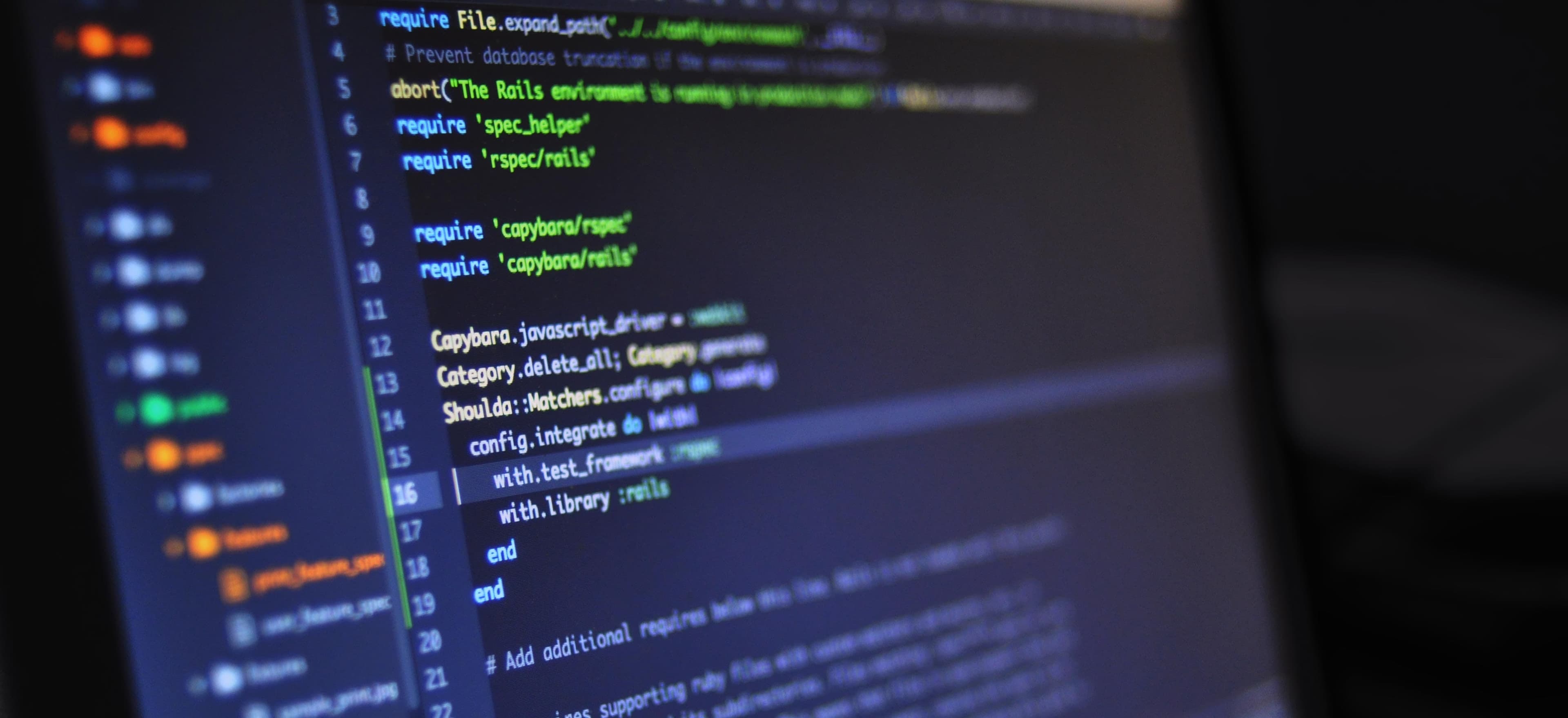
- Published on
Understanding JAXB Root Elements: Common Pitfalls to Avoid
Java Architecture for XML Binding (JAXB) is a powerful framework that allows Java developers to convert Java objects into XML representations and vice versa. Although JAXB simplifies the process of working with XML, developers often run into certain pitfalls, especially when handling root elements. This guide will explore common issues related to JAXB root elements and offer practical advice on how to avoid them.
What is JAXB?
JAXB provides a way to bind XML schemas and Java representations and enables developers to marshal (convert Java objects to XML) and unmarshal (convert XML back to Java objects) with ease. Under the hood, JAXB uses Java annotations to map Java classes to XML elements.
Key Benefits of Using JAXB
- Reduced Complexity: It minimizes boilerplate code, allowing developers to focus on business logic.
- Java Object Manipulation: It lets developers work with standard Java objects rather than dealing directly with XML.
- Schema Validation: JAXB can validate XML against specified schemas during unmarshalling.
Getting Started with JAXB Root Elements
Basic JAXB Setup
Before delving into root elements, let's set up a simple JAXB environment. Assume you have JAXB dependencies integrated into your project (for example, in Maven):
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>2.3.1</version>
</dependency>
Defining a JAXB Root Element
The root element of an XML document is the primary element that encapsulates all other XML elements. To designate a Java class as a JAXB root element, you would typically use the @XmlRootElement
annotation.
Here's a simple example:
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "person")
public class Person {
private String name;
private int age;
public Person() {
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Marshalling and Unmarshalling
Once you have your root element defined, you can marshal (convert a Java object to XML) and unmarshal (convert XML back to a Java object) using JAXB's Marshaller
and Unmarshaller
.
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import java.io.StringReader;
import java.io.StringWriter;
public class JAXBExample {
public static void main(String[] args) throws JAXBException {
// Marshalling
Person person = new Person("John Doe", 30);
JAXBContext jaxbContext = JAXBContext.newInstance(Person.class);
Marshaller marshaller = jaxbContext.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE);
StringWriter sw = new StringWriter();
marshaller.marshal(person, sw);
String xmlOutput = sw.toString();
System.out.println("Marshalled XML:\n" + xmlOutput);
// Unmarshalling
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
Person unmarshalledPerson = (Person) unmarshaller.unmarshal(new StringReader(xmlOutput));
System.out.println("Unmarshalled Person: " + unmarshalledPerson.getName() + ", Age: " + unmarshalledPerson.getAge());
}
}
Common Pitfalls with JAXB Root Elements
Despite its simplicity, developers often encounter certain pitfalls when working with JAXB root elements. Let's discuss some of these in detail.
1. Missing Default Constructor
Pitfall: Failing to provide a default (no-argument) constructor in your root element class.
Why It Matters: JAXB requires a default constructor to create instances of root elements during the unmarshalling process. Without one, an exception will be thrown.
Solution: Always implement a public no-argument constructor in your JAXB-bound classes.
2. Incorrect Namespace Handling
Pitfall: Not specifying or misconfiguring XML namespaces.
Why It Matters: JAXB leverages namespace declarations to avoid element name clashes, especially when dealing with different XML schemata. Ignoring namespaces can lead to unexpected results or failure in marshaling/unmarshaling.
Solution: Use @XmlRootElement(namespace = "your-namespace-here")
and ensure consistency across XML and Java representations.
3. Non-Serializable Fields
Pitfall: Including non-serializable fields in your JAXB class.
Why It Matters: JAXB may not know how to handle certain types of fields, such as transient fields or custom non-standard types, leading to runtime exceptions.
Solution: Use Java primitive types and standard Java objects. For complex types, consider implementing adapters using @XmlJavaTypeAdapter
.
4. Missing XML Annotations
Pitfall: Forgetting to annotate class properties with JAXB annotations.
Why It Matters: If properties are not annotated, JAXB will not include them in the marshaled XML. This can result in missing data when unmarshalling.
Solution: Always annotate fields or their getters with @XmlElement
, @XmlAttribute
, etc.
import javax.xml.bind.annotation.XmlElement;
@XmlRootElement(name = "person")
public class Person {
private String name;
private int age;
// Default constructor
public Person() {}
@XmlElement
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
5. Handling Collections
Pitfall: Mismanaging collections within JAXB classes.
Why It Matters: JAXB can handle collections, but incorrect mappings may lead to runtime errors or incomplete data after marshaling/unmarshalling.
Solution: Use Java Collections such as List
, Set
, or Map
, and annotate appropriately.
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import java.util.ArrayList;
import java.util.List;
@XmlRootElement(name = "group")
public class PeopleGroup {
private List<Person> people = new ArrayList<>();
@XmlElement(name = "person")
public List<Person> getPeople() {
return people;
}
public void setPeople(List<Person> people) {
this.people = people;
}
}
Wrapping Up
By understanding JAXB root elements and being aware of common pitfalls, Java developers can effectively utilize JAXB for XML processing. An awareness of proper class structure, annotations, and configuration can significantly reduce errors and enhance productivity.
Additional Resources
For more comprehensive coverage of JAXB, consider exploring the following:
- JAXB Documentation: Official documentation by Oracle that dives deep into JAXB capabilities.
- Introduction to JAXB: A handy guide on JAXB fundamentals, marshaling, unmarshaling, and advanced configurations.
By following these best practices and avoiding common pitfalls, you can streamline your XML interactions and focus more on developing features that matter. Happy coding!
Checkout our other articles