Mastering Java 8: Tackling The Stream API Learning Curve
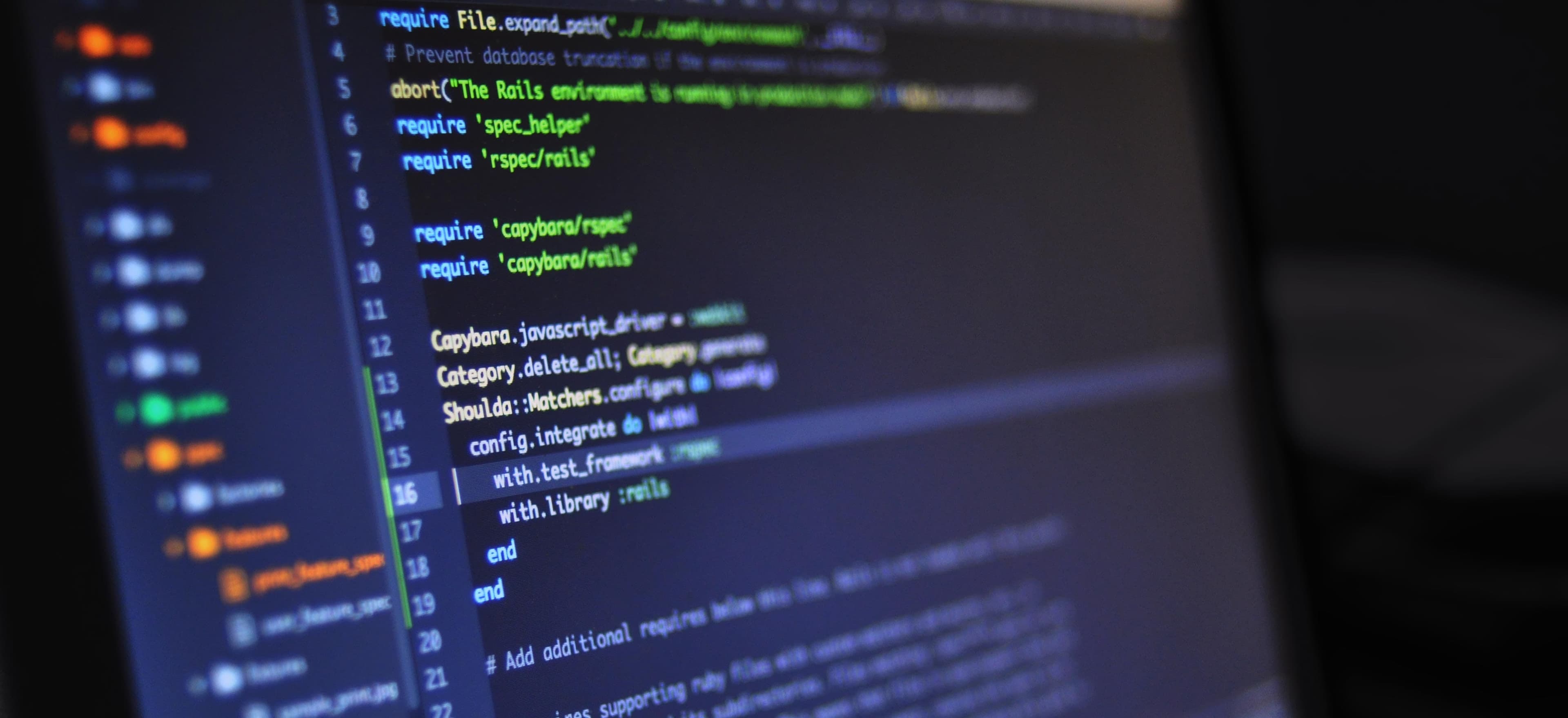
- Published on
Mastering Java 8: Tackling The Stream API Learning Curve
When Java 8 was released in 2014, it brought a multitude of new features to the table, and the Stream API is undoubtedly one of the most powerful and game-changing additions. Streams provide a modern, concise, and functional approach to processing data in Java, and unlocking their potential can significantly elevate your code. In this blog post, we'll delve into the intricacies of the Stream API, exploring its features, best practices, and how it can revolutionize your Java programming.
Understanding Streams in Java 8
In Java, a stream is not a data structure, but rather a new construct that allows you to process data in a functional manner. Leveraging the power of lambda expressions, streams enable developers to write concise and readable code for data manipulation. Streams can be operated in a pipeline fashion, where a sequence of operations can be applied to the data.
Let's dive into a foundational example to illustrate the power of streams:
List<String> languages = Arrays.asList("Java", "Python", "JavaScript", "C#");
// Using Streams to filter and print languages starting with "J"
languages.stream()
.filter(lang -> lang.startsWith("J"))
.forEach(System.out::println);
In this code snippet, the filter
method processes the stream, retaining only elements that fulfill the specified condition. The forEach
method then performs an action, in this case, printing the filtered results.
The Beauty of Stream Chaining
One of the highlights of the Stream API is the ability to chain multiple operations together. This lets you write compact and expressive code, reducing the need for traditional loops and conditional statements.
Let's take an example of mapping and reducing using streams:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using Streams to map, filter, and reduce
int sum = numbers.stream()
.map(num -> num * 2)
.filter(num -> num > 5)
.reduce(0, Integer::sum);
System.out.println("Sum: " + sum);
The map
operation modifies each element by doubling its value, the filter
operation then retains only numbers greater than 5, and finally, the reduce
operation sums up all the filtered numbers. This sequence of actions is not only concise but also comprehensible, showcasing the elegance of stream chaining.
Advantages of Using Streams
Enhanced Readability and Maintainability
Streams promote a more declarative style of programming, where code focuses on expressing what is to be done rather than how it should be done. This leads to cleaner, more readable code that is easier to maintain and understand.
Parallel Execution Support
Another significant advantage of streams is their inherent support for parallel execution. Leveraging the parallelStream
method, programmers can efficiently utilize multi-core architectures for processing large data sets. Java takes care of the complexities of parallel execution, allowing developers to focus on the logic of their operations.
Best Practices for Stream Usage
Be Mindful of Intermediate and Terminal Operations
In the realm of streams, it's crucial to understand the distinction between intermediate and terminal operations. Intermediate operations (such as filter
, map
, and sorted
) return a new stream and are typically lazy, meaning they do not process elements until a terminal operation is invoked. Terminal operations (such as forEach
, reduce
, and collect
) trigger the processing of the stream and produce a non-stream result. When constructing stream pipelines, it's vital to ensure the correct order and usage of these operations to prevent unexpected behavior.
Embrace Method References and Lambda Expressions
Streams heavily leverage lambda expressions and method references, which are key features introduced in Java 8. Embracing these modern constructs can vastly enhance the clarity and conciseness of your stream operations. Leveraging method references can declutter the code, making it more focused on the actual data processing logic.
Key Takeaways
The Stream API in Java 8 represents a paradigm shift in the way developers approach data processing. With its functional, expressive, and parallel execution capabilities, streams offer a streamlined approach to handling data. By mastering the Stream API and integrating it into your Java projects, you can elevate your code to new heights of elegance and efficiency. Harness the power of streams to unlock the full potential of modern Java programming.
In conclusion, Java 8's Stream API is a potent tool that embodies the evolution of the language towards a more functional and expressive paradigm. By embracing the stream's capabilities and adopting best practices, developers can significantly enhance the readability, performance, and maintainability of their code. With the increasing ubiquity of Java 8 and beyond, proficiency in utilizing streams is essential for any Java developer aiming to stay competitive in the ever-evolving landscape of software development.