Conquer Selenium Tests: Enable JavaScript Swiftly!
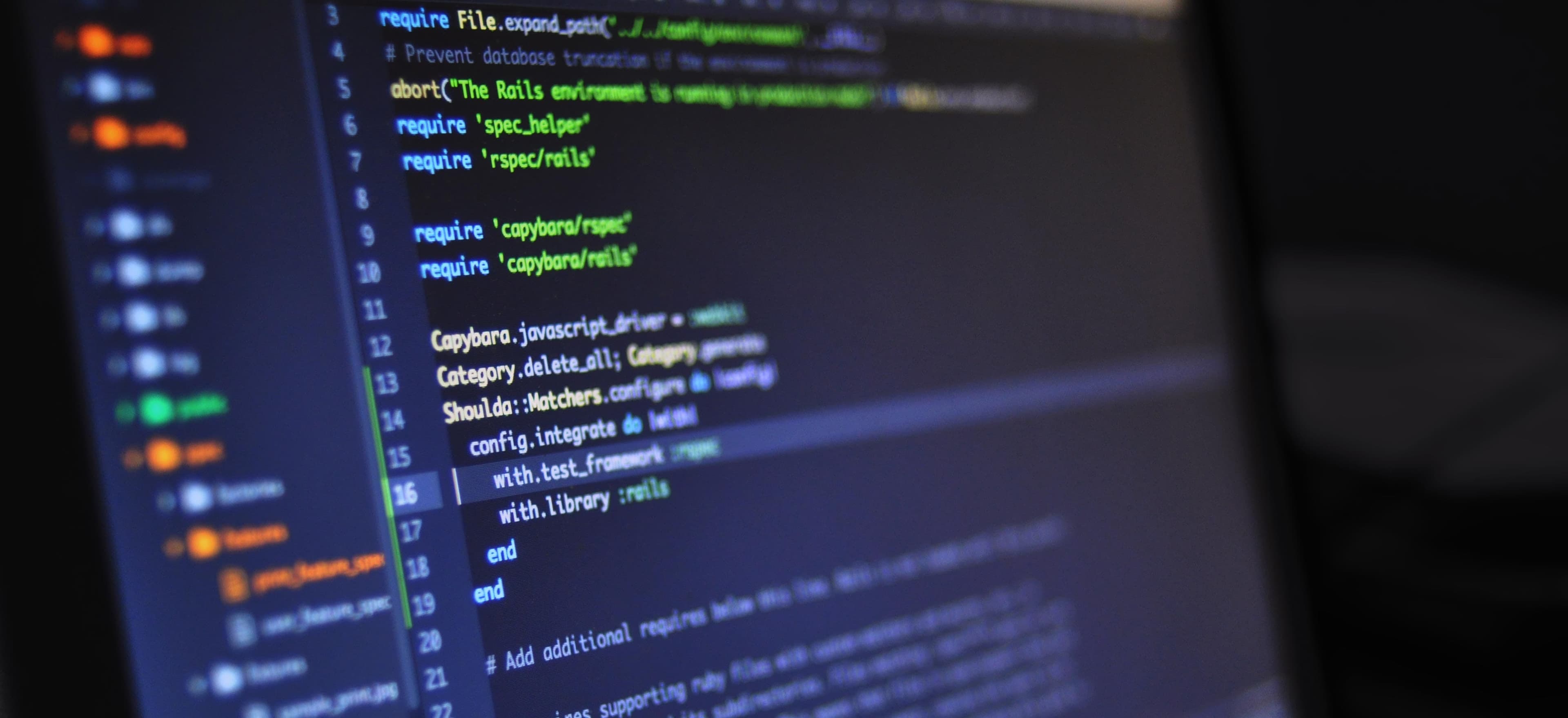
- Published on
Mastering JavaScript Execution in Selenium Testing
Selenium is a powerful tool for automating web browsers, and JavaScript plays a crucial role in modern web development. When it comes to testing web applications, it's essential to ensure that JavaScript is executed properly. In this blog post, we'll delve into the intricacies of enabling JavaScript execution in Selenium testing and explore best practices for seamlessly integrating JavaScript capabilities into your test scripts.
Why is JavaScript Execution Important in Selenium Testing?
JavaScript is the backbone of dynamic and interactive web pages, enabling functionalities such as form validation, dynamic content loading, and AJAX requests. When performing automated testing with Selenium, it is imperative to ensure that JavaScript is executed as expected to accurately replicate user interactions with the web application. Failing to execute JavaScript correctly can lead to incomplete test coverage and inaccurate results, ultimately undermining the validity of your test suite.
Default JavaScript Execution Behavior in Selenium WebDriver
By default, Selenium WebDriver enables JavaScript execution when interacting with web elements and performing actions such as click events and form submissions. However, there are scenarios where you may need to explicitly validate and manipulate JavaScript code within your test scripts, especially when dealing with complex web applications that heavily rely on JavaScript for their functionality.
Ensuring JavaScript Execution: Best Practices
1. Validate JavaScript Execution with Explicit Waits
When interacting with dynamic web elements that are manipulated or loaded via JavaScript, it's crucial to use explicit waits to ensure that the elements are fully rendered and interactive. By employing WebDriverWait in combination with expected conditions such as elementToBeClickable or elementToBeSelected, you can effectively validate that JavaScript execution has completed before proceeding with further actions.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("example")));
element.click();
Using explicit waits not only facilitates proper JavaScript execution but also enhances the stability and reliability of your Selenium tests.
2. Leveraging JavaScriptExecutor for Advanced Interactions
The JavaScriptExecutor interface in Selenium WebDriver unlocks advanced capabilities for interacting with web elements and executing custom JavaScript code within the browser environment. This can be particularly useful for scenarios where standard WebDriver methods fall short in handling complex JavaScript-based functionalities.
JavascriptExecutor jsExecutor = (JavascriptExecutor) driver;
jsExecutor.executeScript("arguments[0].setAttribute('value', 'inputText')", inputElement);
By leveraging JavaScriptExecutor, you can seamlessly integrate JavaScript snippets into your test scripts to cater to intricate interactions that cannot be achieved through conventional WebDriver methods.
3. Handling JavaScript Alerts and Popup Windows
In web applications, JavaScript alerts, confirmations, and prompt dialogs are commonly used to prompt user input or convey critical information. When automating tests with Selenium, it is essential to effectively handle these JavaScript-generated popups using the Alert interface, ensuring that your test scripts seamlessly interact with and respond to JavaScript dialogs as expected.
Alert alert = driver.switchTo().alert();
String alertText = alert.getText();
alert.accept();
By incorporating the handling of JavaScript dialogs into your test scripts, you can maintain the integrity of your test scenarios and mitigate potential disruptions caused by unhandled popups.
Overcoming Common Challenges in JavaScript Execution
1. Dealing with Asynchronous JavaScript Operations
JavaScript's asynchronous nature can pose challenges when synchronizing interactions within Selenium test scripts. When dealing with operations such as AJAX requests or dynamic content loading, it's vital to employ explicit waits and asynchronous execution handling techniques to ensure that your test scripts account for JavaScript-induced delays and dynamic page updates.
2. Mitigating Cross-Browser Inconsistencies
Different web browsers may exhibit variations in how they handle JavaScript execution, potentially leading to inconsistencies in test results across multiple browser environments. To address this, thorough cross-browser testing and meticulous validation of JavaScript execution are crucial to ascertain consistent behavior and functionality across diverse browser configurations.
Lessons Learned
Enabling seamless JavaScript execution in Selenium testing is essential for comprehensive test coverage and accurate validation of web application functionality. By adhering to best practices such as leveraging explicit waits, harnessing JavaScriptExecutor for advanced interactions, and effectively handling JavaScript-generated popups, you can elevate the robustness and reliability of your Selenium test suite while conquering the intricacies of JavaScript execution within the modern web landscape.
In the realm of Selenium testing, mastering JavaScript execution empowers you to craft resilient and effective test scripts that accurately encapsulate the dynamic nature of web applications, ultimately paving the path to comprehensive test coverage and enhanced quality assurance.
To delve deeper into the world of Selenium testing and JavaScript execution, consider exploring the official Selenium WebDriver documentation and resources from SeleniumHQ. Additionally, for insights into JavaScript best practices and capabilities, reputable sources such as MDN Web Docs offer valuable knowledge to augment your proficiency in JavaScript execution within Selenium testing.
Checkout our other articles