Streamline Automation: Integrating Playwright with JUnit 5
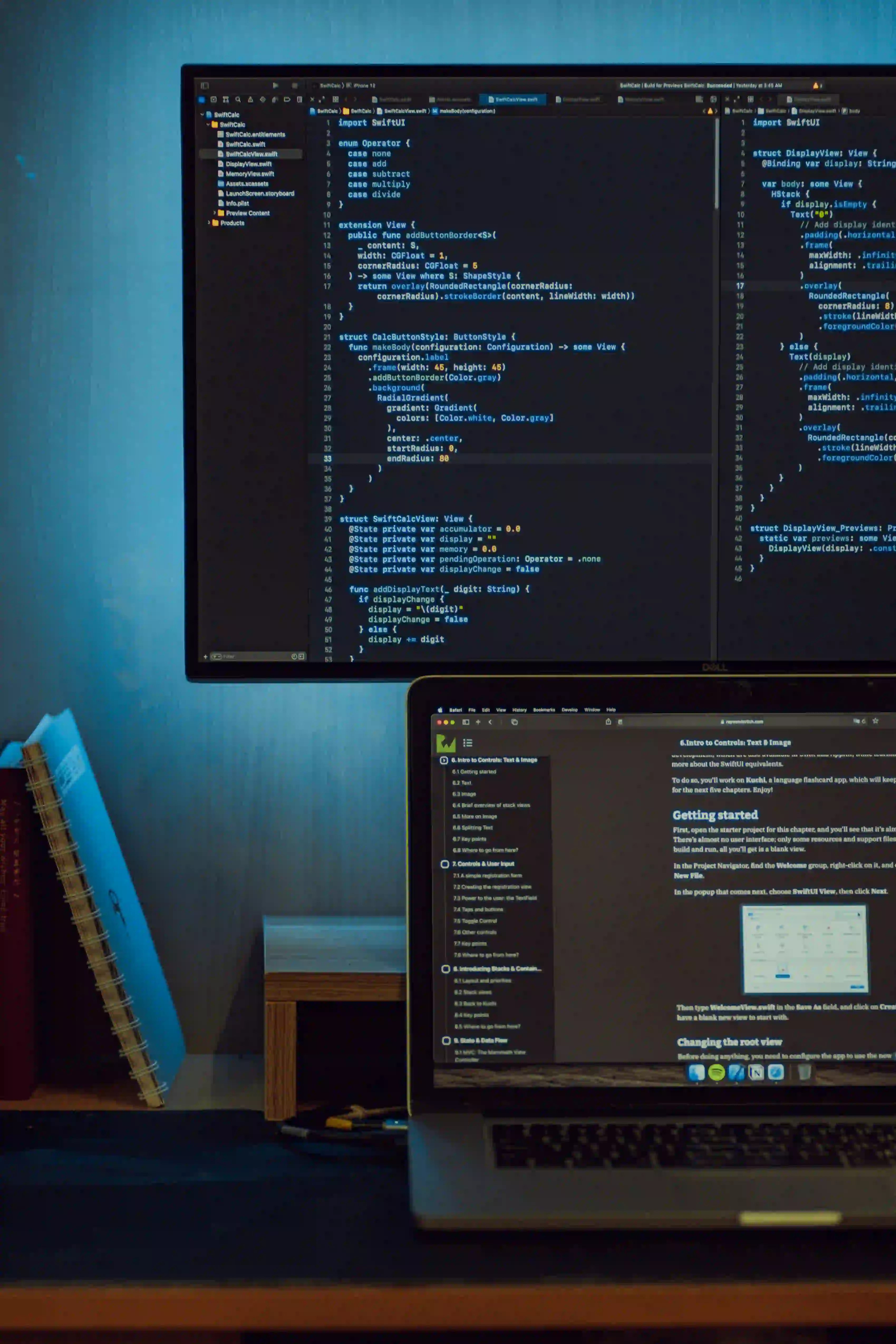
Streamline Automation: Integrating Playwright with JUnit 5
Automated testing is an integral part of modern software development, and Java has always been a preferred choice for building robust test automation frameworks. With the emergence of new tools and technologies, it's essential to adapt and streamline the automation process to achieve better efficiency and maintainability.
In this article, we will explore the integration of Playwright, a powerful automation library for modern web browsers, with JUnit 5, the latest version of the popular Java testing framework. This integration will enable us to leverage the capabilities of Playwright to write reliable end-to-end tests and seamlessly integrate them into our existing JUnit test suite.
Why Choose Playwright for Automation?
Playwright, developed by Microsoft, is a modern and developer-friendly automation library that provides a unified API to automate browsers such as Chromium, WebKit, and Firefox. It offers several advantages over traditional automation tools, including:
-
Cross-Browser Compatibility: Playwright allows you to write consistent tests that can be executed across different browser engines, ensuring the broadest possible test coverage.
-
Native Support for Modern Web Technologies: Playwright natively supports modern web technologies such as Single Page Applications (SPAs) and Web Components, making it suitable for testing contemporary web applications.
-
Asynchronous Execution: Playwright's API is built around modern async/await syntax, enabling efficient and non-blocking automation code.
Setting Up the Project
Before we dive into the integration, let's set up a new Maven project and add the necessary dependencies for Playwright and JUnit 5.
Step 1: Create a Maven Project
Use the following command to create a new Maven project or configure an existing one:
mvn archetype:generate -DgroupId=com.example -DartifactId=playwright-junit5-integration -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Step 2: Add Dependencies
Add the dependencies for Playwright and JUnit 5 in the pom.xml
file:
<dependencies>
<!-- Playwright -->
<dependency>
<groupId>com.microsoft.playwright</groupId>
<artifactId>playwright</artifactId>
<version>1.9.2</version>
<scope>test</scope>
</dependency>
<!-- JUnit 5 -->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
Step 3: Verify Dependencies
Verify that the dependencies are successfully resolved by running the following Maven command:
mvn clean install
Once the project is set up and the dependencies are in place, we are ready to integrate Playwright with JUnit 5 for writing automated tests.
Integrating Playwright with JUnit 5
To integrate Playwright with JUnit 5, we will leverage the @BeforeAll
and @AfterAll
annotations provided by JUnit 5 to set up and tear down the browser context before and after running the tests.
Step 1: Create a Test Class
Create a new test class, for example, PlaywrightJUnitIntegrationTest
, and annotate it with @TestInstance(TestInstance.Lifecycle.PER_CLASS)
to ensure that the test methods are executed against the same instance of the test class.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import org.junit.jupiter.api.*;
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class PlaywrightJUnitIntegrationTest {
private Browser browser;
private BrowserContext context;
@BeforeAll
public void setUp() {
browser = Playwright.create().chromium().launch();
context = browser.newContext();
}
@AfterAll
public void tearDown() {
context.close();
browser.close();
}
// Add test methods here
}
In this setup, the setUp
method initializes the browser and creates a new browser context using Playwright before the test class execution, while the tearDown
method closes the browser context and the browser after all the test methods have been executed.
Step 2: Write Test Methods
Now, let's add some test methods to interact with a sample web application using Playwright's API. For example, let's navigate to a website, assert the page title, and validate the presence of a specific element.
@Test
public void testPageNavigation() {
var page = context.newPage();
page.navigate("https://example.com");
Assertions.assertEquals("Example Domain", page.title());
}
@Test
public void testElementVisibility() {
var page = context.newPage();
page.navigate("https://example.com");
var element = page.locator("h1");
Assertions.assertTrue(element.isVisible());
}
In these test methods, we utilize Playwright's API to create a new page, navigate to a URL, retrieve the page title, and validate the visibility of an element.
Step 3: Run the Tests
Run the test class, PlaywrightJUnitIntegrationTest
, using the JUnit 5 test runner, and observe the browser automation in action.
Benefits of Playwright-JUnit Integration
The integration of Playwright with JUnit 5 brings several benefits to the table:
-
Robust and Efficient Tests: Playwright's modern automation capabilities combined with JUnit 5's testing framework enable the creation of robust and efficient end-to-end tests for web applications.
-
Unified Testing Infrastructure: By integrating Playwright with JUnit 5, teams can leverage a unified infrastructure for both unit tests and end-to-end tests, leading to a cohesive testing strategy.
-
Cross-Browser Testing: Playwright's cross-browser compatibility, when integrated with JUnit 5, allows for seamless execution of tests across different browser environments.
Lessons Learned
In this article, we have explored the process of integrating Playwright, a modern browser automation library, with JUnit 5, a powerful testing framework for Java. By combining the strengths of both tools, we can achieve a streamlined and efficient automation process, enabling the creation of reliable end-to-end tests for web applications.
The integration of Playwright with JUnit 5 not only enhances the testing capabilities but also fosters a cohesive testing ecosystem within the Java development landscape. As technology continues to evolve, adapting and integrating modern automation tools becomes imperative for delivering high-quality software.
By embracing the integration of Playwright with JUnit 5, teams can elevate their automated testing efforts and ensure the robustness and quality of their web applications across different browser environments. Start integrating Playwright with JUnit 5 today to unleash the full potential of browser automation in your Java projects.