Unraveling SBE: Solving Slow Data Serialization Dilemmas
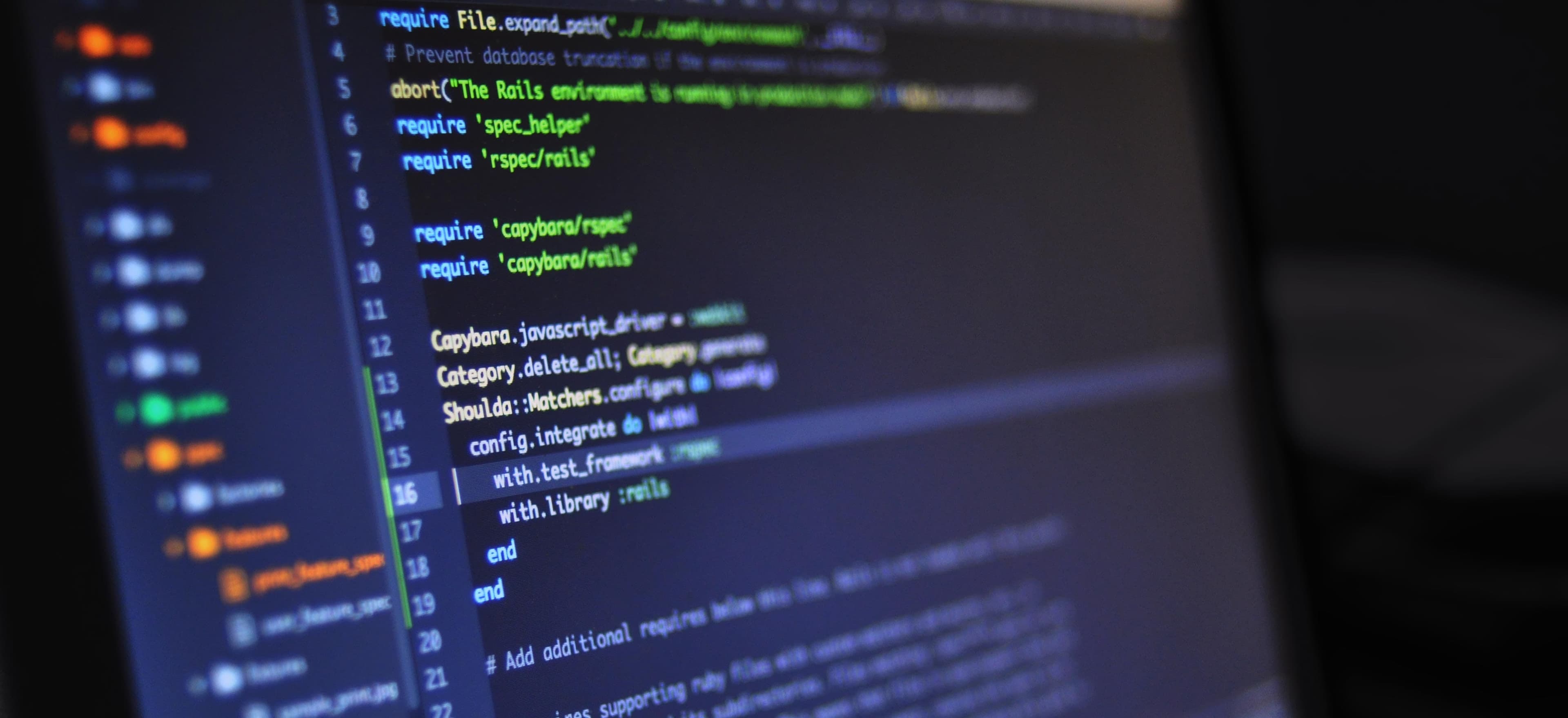
- Published on
Unraveling SBE: Solving Slow Data Serialization Dilemmas
In the world of high-frequency trading, every millisecond counts. The performance of data serialization and deserialization operations can significantly impact the overall throughput and latency of trading systems. This is where Simple Binary Encoding (SBE) comes into the picture. SBE offers a high-performance solution for encoding and decoding financial messages, providing a swift and efficient alternative to traditional XML and JSON formats.
What is SBE?
SBE is a data encoding format that optimizes the serialization and deserialization of messages, especially in financial trading systems where speed is critical. It uses a simple binary encoding scheme that facilitates ultra-fast processing of data. SBE's usage extends beyond just trading systems; it is applicable in any real-time, high-throughput data processing scenarios.
The Need for Speed
In the context of financial trading, microseconds can translate into significant monetary gains or losses. Therefore, minimizing the time spent on encoding and decoding messages is paramount. Traditional text-based formats like XML and JSON, while being human-readable, are inefficient in terms of processing speed. They introduce unnecessary overhead due to parsing and handling of textual data.
SBE addresses these inefficiencies by utilizing a binary encoding approach. By doing so, it eliminates the need for parsing and interpreting textual data, leading to remarkable performance gains.
SBE Schema Definition
At the core of SBE is its schema definition, which describes the structure of messages in a binary format. The schema enables the generation of encoding and decoding logic for various programming languages. SBE provides a schema language that allows defining message structures, data types, and encoding rules.
Let's take a look at a simple SBE schema for representing a trade message:
/* SBE schema for trade message */
message Trade {
int64 timestamp;
int32 instrumentId;
int32 quantity;
float price;
}
In this example, we define a Trade
message containing fields for timestamp, instrument ID, quantity, and price, each with its specified data type.
Generating Code from SBE Schema
Once the SBE schema is defined, we can use the SBE tooling to generate the encoding and decoding logic for our defined messages. The SBE tooling takes the schema as input and produces language-specific source code that can be integrated into our application.
For instance, using the SBE tooling, we can generate Java classes to encode and decode trade messages based on the previously defined schema.
Encoding and Decoding with SBE
Let's delve into an example of encoding and decoding a trade message using SBE in Java.
First, we need to initialize an instance of the generated SBE DirectBuffer
and set it up with the encoded trade message. Then, we can proceed with decoding the message fields from the buffer.
// Encoding a trade message
TradeEncoder tradeEncoder = new TradeEncoder();
DirectBuffer encodedBuffer = new DirectBuffer(new byte[TradeEncoder.BLOCK_LENGTH]);
tradeEncoder.wrapAndApplyHeader(encodedBuffer, 0, new MessageHeaderEncoder());
tradeEncoder.timestamp(System.currentTimeMillis());
tradeEncoder.instrumentId(12345);
tradeEncoder.quantity(1000);
tradeEncoder.price(175.50);
// Decoding a trade message
TradeDecoder tradeDecoder = new TradeDecoder();
DirectBuffer decodedBuffer = new DirectBuffer(encodedBuffer.byteArray());
tradeDecoder.wrap(decodedBuffer, 0, TradeDecoder.BLOCK_LENGTH, TradeDecoder.SCHEMA_VERSION);
long timestamp = tradeDecoder.timestamp();
int instrumentId = tradeDecoder.instrumentId();
int quantity = tradeDecoder.quantity();
float price = tradeDecoder.price().floatValue();
In this example, we use the SBE-generated TradeEncoder
to encode a trade message and then use the corresponding TradeDecoder
to decode the message fields from the encoded buffer.
Benchmarking SBE Performance
To truly appreciate the impact of SBE on serialization and deserialization performance, benchmarking is essential. By comparing the throughput and latency of SBE-encoded messages with those of traditional text-based formats, we can quantify the efficiency gains provided by SBE.
Benchmarking can be accomplished by measuring the time taken to encode and decode a large number of messages using both SBE and a comparative text-based format. This empirical data will provide concrete evidence of SBE's superiority in terms of speed and efficiency.
Leveraging SBE in Real-World Applications
SBE's benefits extend beyond the realm of high-frequency trading. Any system that demands rapid processing of structured data can reap the rewards of SBE's performance enhancements. Whether it's high-throughput messaging systems, market data distribution, or any real-time data processing, SBE can be a valuable asset in optimizing the overall system performance.
Final Thoughts
In the fast-paced world of high-frequency trading and real-time data processing, performance is paramount. SBE offers a compelling solution to the slow data serialization dilemmas that have plagued traditional text-based formats. By leveraging binary encoding and a well-defined schema, SBE catapults the processing speed of messages to a whole new level. Its impact transcends the financial domain, finding relevance in any system where speed and efficiency are non-negotiable.
With the prowess of SBE, systems can achieve unparalleled performance, empowering them to handle massive data volumes with exceptional speed and precision. As we continue to embrace the era of real-time data, SBE stands as a beacon of efficiency, resolving the challenges of slow data serialization and propelling our systems towards unprecedented levels of performance.
Checkout our other articles