Unraveling the Magic: Demystifying @Configurable in Spring
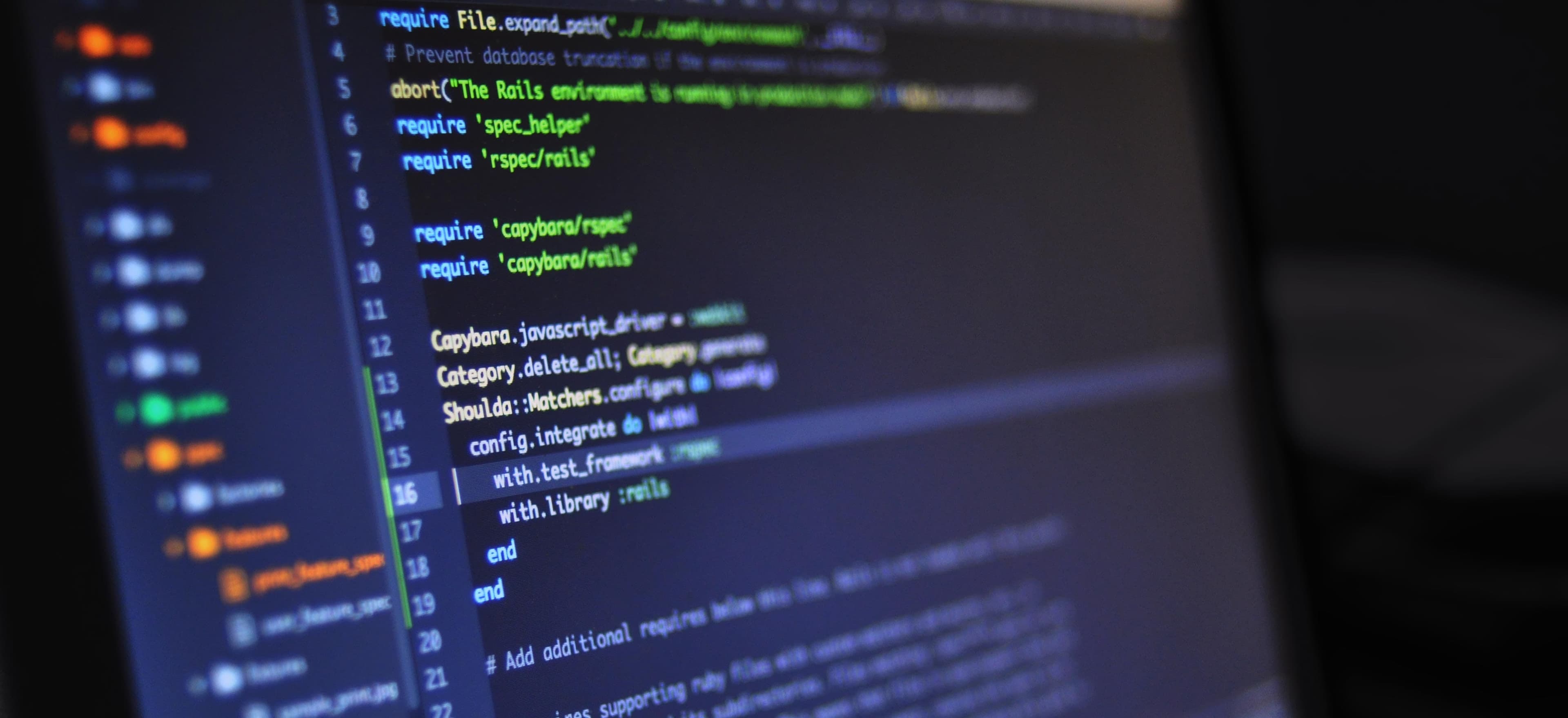
- Published on
Demystifying '@Configurable' in Spring
When diving into the realm of Spring framework, developers are often intrigued by the plethora of annotations it offers. One such annotation is '@Configurable'. This annotation may seem mysterious at first, but understanding its nuances and potential applications can greatly enhance our grasp of the Spring framework. In this blog post, we'll unravel the magic behind '@Configurable' and shed light on how it can be utilized effectively in our Java applications.
Understanding '@Configurable'
At its core, '@Configurable' is an annotation provided by the Spring framework that allows for dependency injection into domain objects. This means that it enables the dependency injection mechanism to be applied to objects that are not managed by the Spring container. This seemingly simple concept carries significant implications for the design and architecture of our applications.
The Magic of AspectJ
The magic behind '@Configurable' lies in its integration with AspectJ, a powerful and versatile aspect-oriented programming (AOP) framework. AspectJ enables the weaving of aspects into our code at compile-time or runtime. When we apply '@Configurable' to a class, AspectJ goes to work behind the scenes, enhancing the class and enabling Spring to perform dependency injection on it, irrespective of whether it is managed by the Spring container.
An Example Scenario
Let's consider a practical scenario to better understand the use case for '@Configurable'. Suppose we have a domain object User
that is not managed by the Spring container. We want to inject a service userService
into this domain object. By annotating the User
class with '@Configurable' and using AspectJ weaving, we can seamlessly inject the userService
dependency without having to manually manage the User
object within the Spring container.
Code Walkthrough
@Configurable
public class User {
@Autowired
private UserService userService;
// Constructors, methods, and other members
}
In the above code snippet, the '@Configurable' annotation marks the User
class as eligible for weaving by AspectJ. The @Autowired
annotation on the userService
field indicates that the dependency will be injected by the Spring framework.
Enabling '@Configurable' Support
To enable '@Configurable' support in our Spring application, we need to make a few configurations. First, we must ensure that AspectJ is correctly configured and weaving is enabled. Next, we need to enable load-time weaving in our Spring configuration. This can be achieved by referencing the 'aspectj' agent in the JVM arguments when starting the application.
Code Walkthrough
@EnableLoadTimeWeaving(aspectjWeaving = AspectJWeaving.ENABLED)
public class AppConfig {
// Configuration details
}
In the above configuration class, we enable load-time weaving along with AspectJ weaving using the @EnableLoadTimeWeaving
annotation.
Leveraging the Power of AspectJ
It's worth noting that the integration of '@Configurable' with AspectJ provides a powerful way to apply cross-cutting concerns and aspects to our domain objects. AspectJ allows us to define aspects that can be woven into classes, effectively decoupling concerns such as logging, security, and transactions from the core business logic. This not only simplifies our codebase but also enhances maintainability and testability.
When to Use '@Configurable'
While '@Configurable' offers powerful capabilities, it's essential to use it judiciously. Its primary use case arises when we need to perform dependency injection into domain objects that are not managed by the Spring container. This typically occurs when dealing with objects instantiated outside the purview of the Spring context, such as entities in a JPA (Java Persistence API) context or objects created using the 'new' operator.
Best Practices and Considerations
When employing '@Configurable', we should adhere to best practices to ensure its effective usage. It's crucial to maintain clarity in the design and understand the implications of applying aspects through AspectJ. Additionally, thorough testing, especially integration testing, becomes vital to validate the behavior of '@Configurable' annotated classes.
In Conclusion, Here is What Matters
In conclusion, '@Configurable' in Spring, while initially shrouded in mystery, unveils itself as a powerful tool for enabling dependency injection into non-managed domain objects. By integrating with AspectJ, it opens avenues for weaving aspects and applying cross-cutting concerns seamlessly. Understanding the nuances of '@Configurable' equips us with the capability to architect robust and flexible applications, leveraging the full potential of the Spring framework.
By leveraging the power of '@Configurable', we can untangle the complexities of dependency injection and aspect-oriented programming, enriching our Java applications with greater flexibility and elegance.
So, let's embrace the magic of '@Configurable' and unlock a new dimension of possibilities in our Spring-powered Java applications!
Checkout our other articles