Overcoming Maven Dependencies Hell in Java Web Projects
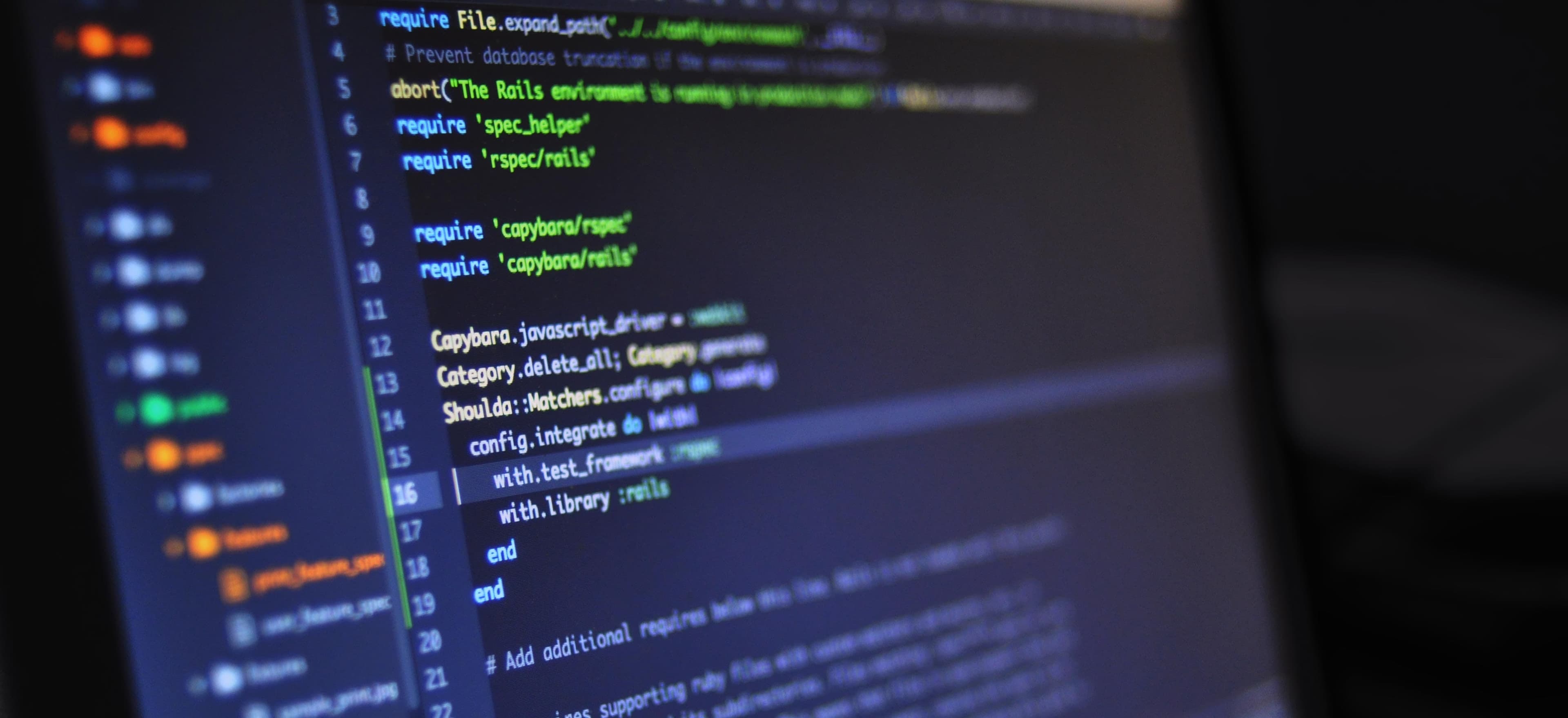
- Published on
Overcoming Maven Dependencies Hell in Java Web Projects
Maven has become an integral part of Java web development, providing a robust way to manage project dependencies and build processes. However, as projects grow in size and complexity, managing dependencies can sometimes turn into a headache commonly referred to as "dependencies hell." In this article, we'll explore common issues faced in managing dependencies in Java web projects and the strategies to overcome them.
The Maven Dependencies Hell
When working on Java web projects, it's common to rely on numerous third-party libraries and frameworks. Maven simplifies the management of these dependencies by automatically resolving transitive dependencies and ensuring the required libraries are available during the build process. However, various challenges can arise, creating what developers refer to as "Maven dependencies hell."
These challenges may include:
- Conflicting Versions: Different dependencies requiring conflicting versions of the same library.
- Transitive Dependencies: Unintended dependencies being pulled in transitively, leading to version conflicts or runtime issues.
- Excessive Dependency Hierarchies: Projects with deep dependency hierarchies making it hard to unravel and isolate issues.
- Unnecessary Dependencies: Including unnecessary dependencies, leading to bloated project sizes and potential conflicts.
Strategies to Overcome Maven Dependencies Hell
1. Use Dependency Management Plugins
Maven offers powerful dependency management plugins such as the maven-enforcer-plugin
and maven-dependency-plugin
. These plugins allow you to enforce rules on dependencies and analyze the project's dependency tree, providing insights into any conflicts or issues.
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-enforcer-plugin</artifactId>
<version>3.0.0-M3</version>
<executions>
<execution>
<id>enforce</id>
<goals>
<goal>enforce</goal>
</goals>
<configuration>
<!-- Enforce specific dependency versions or rules -->
</configuration>
</execution>
</executions>
</plugin>
<!-- Add maven-dependency-plugin configuration as needed -->
</plugins>
2. Use Dependency Exclusion
When experiencing conflicts due to transitive dependencies, Maven allows you to exclude specific transitive dependencies, providing more control over the dependency graph.
<dependency>
<groupId>org.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>org.unwanted</groupId>
<artifactId>unwanted-artifact</artifactId>
</exclusion>
</exclusions>
</dependency>
3. Dependency Management Section
Utilize the <dependencyManagement>
section to centralize and manage the versions of dependencies. This allows for consistent versioning across modules and projects, reducing the likelihood of conflicts.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
</dependency>
<!-- Other dependencies -->
</dependencies>
</dependencyManagement>
4. Use Bill of Materials (BOM)
Create a Bill of Materials (BOM) to centralize and manage dependency versions across multiple projects. By importing a BOM, you can ensure that all projects use the same version for a given set of dependencies.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>example-bom</artifactId>
<version>1.0.0</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
5. Continuous Integration and Dependency Analysis
Integrate continuous integration tools such as Jenkins, TeamCity, or GitLab CI/CD to perform dependency analysis. Tools like JFrog Xray can also be used to identify and remediate any security or licensing issues within dependencies.
6. Regular Dependency Updates
Regularly update dependencies to their latest stable versions, ensuring that your project benefits from bug fixes, performance improvements, and security patches.
Key Takeaways
Managing dependencies in Java web projects can be complex, but by leveraging the right tools and strategies, you can overcome the "Maven dependencies hell" and ensure a robust and stable project. Utilizing dependency management plugins, exclusion, centralized version management, BOM, continuous integration, and regular updates can greatly alleviate the challenges associated with dependencies, leading to a more efficient and reliable development process.
By implementing these strategies, you can navigate the complexities of dependency management with Maven and maintain a well-structured, scalable, and maintainable Java web project.
Remember, while dependencies are essential for building modern Java web applications, effective management is key to preventing the dreaded "dependencies hell." Happy coding!
In this article, we explored the common challenges faced in managing dependencies in Java web projects and provided practical strategies to overcome them. With the tips and best practices shared, you can now effectively navigate Maven dependencies and ensure a smoother development process for your Java web projects. If you found this article helpful, you may also be interested in exploring more about Java development and Maven.