Simplifying Parallelization: A Case Study Breakdown
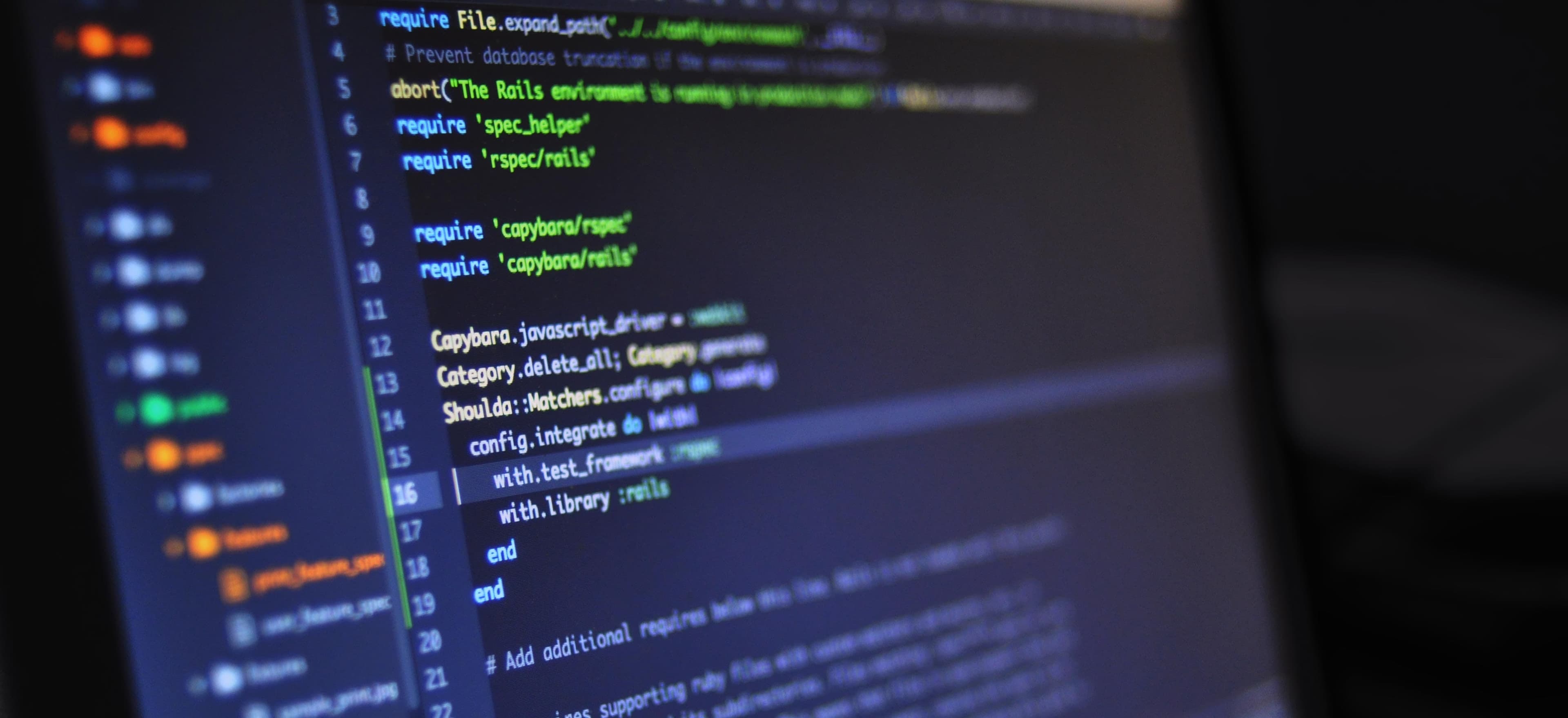
- Published on
Simplifying Parallelization: A Case Study Breakdown
In today's tech-driven world, parallelization has become essential for optimizing the performance of software applications. Java, as a popular programming language, offers robust support for parallel computing through its built-in features and libraries. In this article, we'll delve into a real-world case study to demonstrate the process of simplifying parallelization in Java for enhanced performance and scalability.
Understanding the Need for Parallelization
Before diving into our case study, let's briefly discuss the significance of parallelization in software development. Parallelization involves breaking down a task into smaller sub-tasks that can be executed simultaneously, thereby leveraging the computational power of multi-core processors. This approach is instrumental in improving the efficiency and speed of applications, particularly for tasks involving heavy computation or processing large datasets.
In the context of Java, parallelization can be achieved through techniques such as multithreading, concurrent programming, and the use of parallel streams provided by the Java Stream API. Leveraging these capabilities can lead to significant performance gains, making parallelization a compelling strategy for developers.
The Case Study: Parallelizing Data Processing
Consider a scenario where a Java application needs to process a large volume of data, such as performing complex calculations on financial transactions or analyzing massive datasets. In this case study, we'll focus on parallelizing the data processing tasks to demonstrate the tangible benefits of parallelization in a real-world context.
Traditional Sequential Approach
Let's start by examining a simplified version of the traditional sequential approach to data processing in Java:
public class DataProcessor {
public void processData(List<Data> dataList) {
for (Data data : dataList) {
// Perform data processing tasks
// ...
}
}
}
In the above code snippet, the processData
method iterates through a list of Data
objects and performs the processing tasks sequentially. While this approach is straightforward, it may not fully utilize the available computational resources, especially when dealing with a large dataset or computationally intensive operations.
Parallelizing Data Processing with Java Streams
To demonstrate the power of parallelization, let's refactor the DataProcessor
class to leverage parallel streams offered by the Java Stream API:
import java.util.concurrent.atomic.AtomicInteger;
import java.util.List;
public class DataProcessor {
public void processData(List<Data> dataList) {
AtomicInteger processedCount = new AtomicInteger(0);
dataList.parallelStream()
.forEach(data -> {
// Perform data processing tasks in parallel
// ...
processedCount.getAndIncrement();
});
System.out.println("Total data processed: " + processedCount.get());
}
}
In this refactored code snippet, we've replaced the traditional for-loop with a parallel stream that allows the data processing tasks to be executed concurrently across multiple threads. The AtomicInteger
is being used to safely track the count of processed data, taking into account the parallel execution.
Key Considerations and Best Practices
While parallelizing data processing can lead to performance improvements, it's crucial to consider certain factors and best practices to ensure the effective implementation of parallelization in Java applications:
Task Granularity
When parallelizing tasks, it's important to consider the granularity of the tasks being parallelized. Fine-grained tasks can incur significant overhead due to thread management, while coarse-grained tasks might not fully leverage parallelization. Finding the right balance is crucial for optimal performance.
Thread Safety
In a parallelized environment, ensuring thread safety is paramount to prevent data corruption and race conditions. Utilizing thread-safe data structures or synchronization mechanisms such as synchronized
blocks and java.util.concurrent
classes is essential for maintaining data integrity.
Resource Management
Efficient resource management is critical in parallel computing to prevent issues such as resource contention and excessive resource consumption. Properly configuring thread pools, managing thread lifecycles, and monitoring resource utilization are vital aspects of parallelization.
Testing and Profiling
Thorough testing and performance profiling are indispensable for identifying potential bottlenecks, race conditions, and scalability issues in parallelized code. Tools like JUnit, JMH (Java Microbenchmarking Harness), and profilers can aid in analyzing the behavior and performance of parallelized components.
The Last Word
In this case study breakdown, we've explored the process of simplifying parallelization in Java by parallelizing data processing tasks using the Java Stream API. By leveraging parallel streams, developers can harness the power of multi-core processors to enhance the performance and scalability of their applications. It's important to approach parallelization with careful consideration of task granularity, thread safety, resource management, and thorough testing to unleash its full potential in real-world scenarios.
As technology continues to advance, harnessing the capabilities of parallelization will be increasingly vital for building high-performance, concurrent Java applications that meet the demands of modern computing environments.
Incorporated Links:
Checkout our other articles