Choosing Between 2 Deployment Tactics for Subsystems
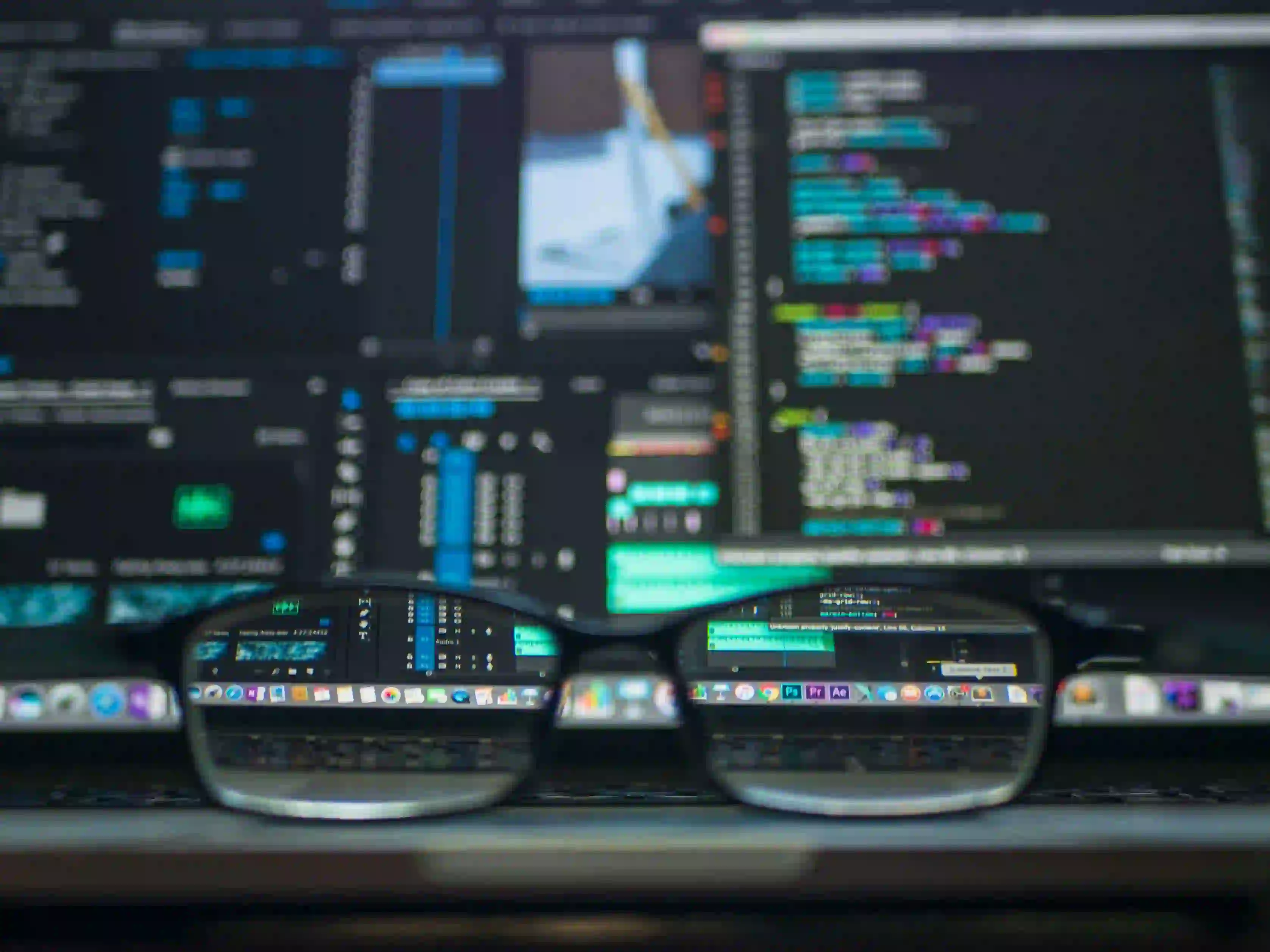
Choosing Between 2 Deployment Tactics for Subsystems
In the realm of software development, the decision-making process often involves choosing between different deployment tactics for subsystems. As a Java developer, it's essential to understand the options available and make informed decisions based on the specific requirements of the project. In this blog post, we will explore two common deployment tactics - microservices and monoliths - and discuss the considerations that can help you make the right choice for your subsystems.
Understanding Microservices
Microservices architecture involves developing a single application as a suite of small services, each running its own process and communicating with lightweight mechanisms. These services are built around business capabilities and independently deployable.
Advantages of Microservices
-
Scalability: Microservices allow individual components to be independently scalable based on demand, offering better resource utilization.
-
Flexibility: Each microservice can be developed, deployed, and maintained independently, providing flexibility in technology stack and updates.
-
Resilience: Failure in one microservice does not necessarily affect the entire system, as other services can continue to function.
Considerations for Microservices Deployment
When considering microservices deployment, it's important to evaluate the level of complexity and operational overhead associated with managing multiple services. Microservices may introduce distributed system complexities such as network latency, eventual consistency, and data management challenges.
Embracing the Monolith
The monolithic architecture, on the other hand, involves building the entire application as a single cohesive unit. All the components of the application are interconnected and interdependent.
Advantages of Monoliths
-
Simplicity: Monolithic architecture simplifies the development and deployment process as the entire application is contained within a single codebase.
-
Easier Debugging: Debugging and testing the application can be more straightforward as all components are tightly coupled.
-
Singular Deployment Unit: Deploying a monolithic application involves deploying the entire system, which can simplify versioning and deployment strategies.
Considerations for Monoliths Deployment
While monolithic architecture offers simplicity, it may pose challenges in terms of scalability and flexibility. As the application grows, it can become harder to maintain and extend the codebase, leading to longer development cycles and potential performance bottlenecks.
Choosing the Right Deployment Tactic
When to Choose Microservices
-
Complex, Diverse Architectures: If your subsystem requires a high level of autonomy, diverse technology stacks, and scalability, microservices might be the right choice.
-
Rapid Iteration & Deployment: If you anticipate frequent updates and changes to individual components without affecting the entire system, microservices offer flexibility in deployment.
-
Fault Isolation: If fault isolation and resilience are critical requirements for your subsystem, microservices can mitigate the impact of failures.
Example of Microservices Deployment in Java
// Sample code for a simple microservice in Java using Spring Boot
@RestController
public class UserController {
@GetMapping("/users/{id}")
public User getUserById(@PathVariable String id) {
// Implementation to retrieve user by ID
}
@PostMapping("/users")
public User createUser(@RequestBody User user) {
// Implementation to create a new user
}
}
In this example, the UserController is a microservice that handles user-related functionalities independently, making it easier to scale and maintain.
When to Choose Monoliths
-
Simplicity and Cohesion: If your subsystem has a relatively simple architecture and does not require complex scaling or diverse technology stacks, a monolithic approach can streamline development.
-
Resource Constraints: For smaller projects or teams with limited resources, a monolithic architecture may be more practical in terms of development and maintenance.
-
Data Integrity: In cases where the subsystem requires strong consistency and data integrity, a monolithic architecture can offer a more straightforward approach.
Example of Monolithic Deployment in Java
// Sample code for a monolithic Java application
public class MonolithicApplication {
public static void main(String[] args) {
// Main entry point for the monolithic application
// Initialization and execution of the entire subsystem
}
}
In this simplified example, the entire subsystem is initialized and executed within a single application, showcasing the cohesive nature of monolithic deployment.
Closing Remarks
In conclusion, the decision between microservices and monolithic deployment tactics for subsystems should be based on an in-depth understanding of the specific requirements, constraints, and future growth prospects of the subsystem. While microservices offer scalability and flexibility, they come with added complexity and operational overhead. On the other hand, monolithic architecture provides simplicity but may become unwieldy as the subsystem evolves.
Understanding the trade-offs and making an informed decision based on the unique needs of the subsystem will ensure a robust and sustainable deployment strategy. Whether you opt for microservices or monoliths, leveraging the strengths of each approach can contribute to the success of your Java projects.
By carefully weighing the considerations and analyzing the potential impact of both deployment tactics, you can steer your subsystem towards a deployment strategy that aligns with its current and future goals.
References: