Beyond Java 8 Streams: Handling Complex Data Operations
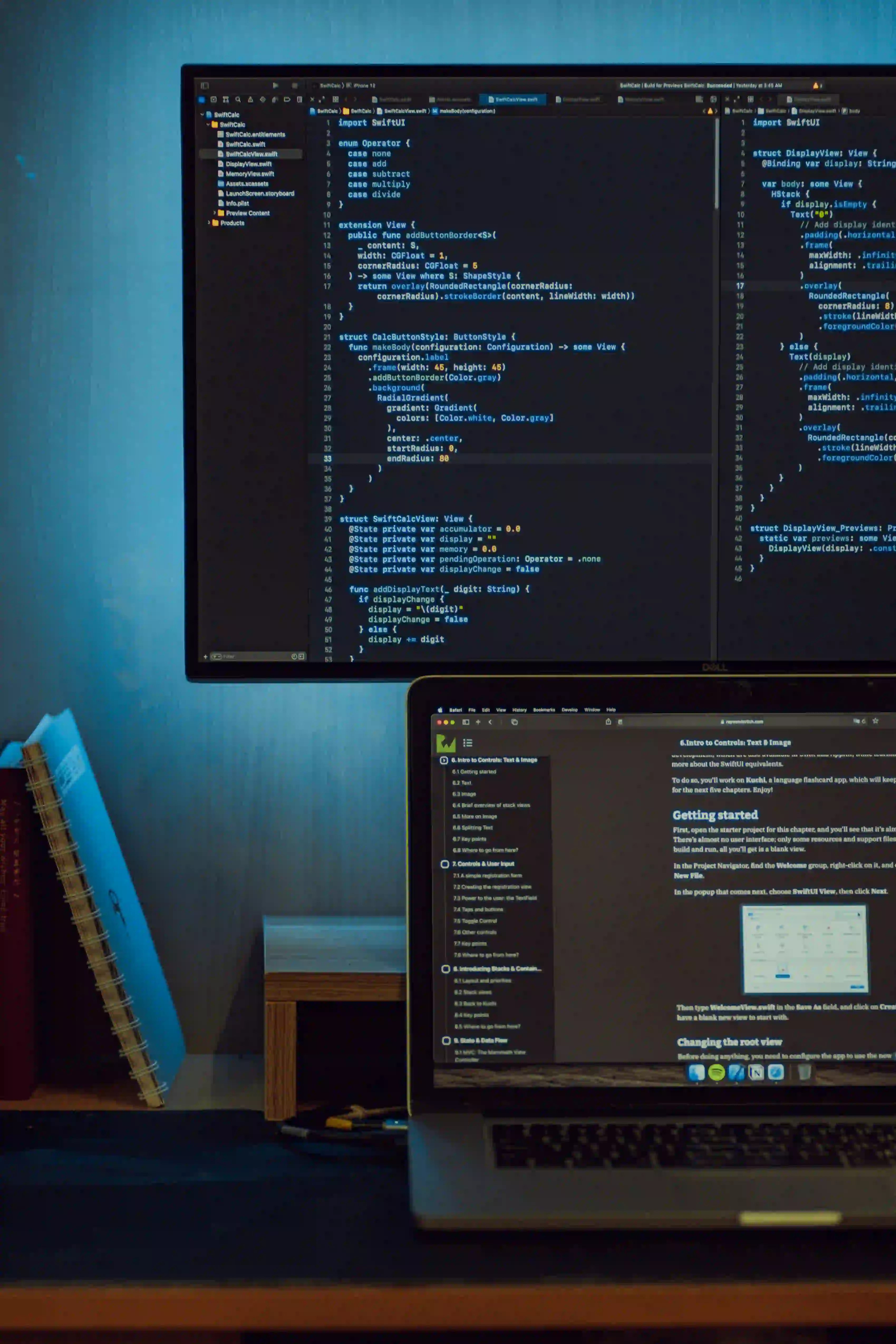
Beyond Java 8 Streams: Handling Complex Data Operations
In the world of Java development, the introduction of Streams in Java 8 revolutionized the way we handle collections and complex data operations. Streams provide a fluent and functional approach to processing data, allowing developers to perform filter, map, reduce, and other operations with ease. However, as applications grow in complexity, the need arises for more advanced techniques to handle intricate data processing scenarios. In this article, we will explore how to go beyond the basics of Java 8 Streams and address complex data operations using various advanced techniques and libraries.
Scenario: Processing Complex Data
Consider a scenario where you have a collection of objects representing financial transactions, and you need to perform advanced aggregation and filtering operations based on different criteria such as transaction type, amount, and date. While basic stream operations can handle simple tasks, complex data operations require a more sophisticated approach.
Leveraging Java 8 Comparator
Java 8 introduced the Comparator
interface with several utility methods, enabling developers to compare objects based on different attributes. When dealing with complex data operations involving sorting and comparing objects, leveraging the Comparator
interface can be immensely helpful.
Example: Sorting Transactions by Amount
Let's say we have a list of Transaction
objects and we want to sort them based on the transaction amount.
List<Transaction> transactions = getTransactions(); // Assume this method retrieves the list of transactions
transactions.sort(Comparator.comparing(Transaction::getAmount));
In this example, we use the Comparator.comparing
method to sort the transactions based on the transaction amount. This concise and readable syntax illustrates the power of utilizing the Comparator
interface in complex data operations.
Handling Complex Filtering with Predicate
In complex data processing scenarios, filtering objects based on multiple criteria becomes essential. Java 8 introduced the Predicate
functional interface, which can be leveraged to filter objects based on complex conditions.
Example: Filtering Large Transactions
Let's say we need to filter large transactions based on both transaction amount and type.
List<Transaction> largeTransactions = transactions.stream()
.filter(t -> t.getAmount() > 1000 && t.getType().equals(TransactionType.LARGE))
.collect(Collectors.toList());
In this example, we utilize the filter
method along with a complex Predicate
to filter transactions based on amount and type, showcasing the flexibility and power of Predicate
in handling complex filtering requirements.
Advanced Aggregation with Collectors
While basic stream operations allow for simple aggregation using methods like sum
and average
, complex data operations often necessitate advanced aggregation techniques. Java 8's Collectors
class provides an array of powerful methods for complex data aggregation.
Example: Calculating Total Transactions by Type
Assuming we have a list of transactions and we want to calculate the total transaction amount for each transaction type.
Map<TransactionType, Double> totalByType = transactions.stream()
.collect(Collectors.groupingBy(Transaction::getType, Collectors.summingDouble(Transaction::getAmount)));
In this example, we utilize the groupingBy
and summingDouble
collectors to calculate the total transaction amount for each transaction type, showcasing how Collectors
can be utilized for advanced aggregation in complex data operations.
Beyond Java 8: Leveraging Third-Party Libraries
While Java 8 introduced significant enhancements for handling complex data operations, there are scenarios where leveraging third-party libraries can further streamline and simplify intricate data processing tasks.
Example: Processing Time Series Data with Apache Commons Math
Consider a scenario where you need to perform complex calculations on time series data such as mean, variance, and regression analysis. Leveraging the Apache Commons Math library can provide powerful tools for handling such complex data operations.
DescriptiveStatistics stats = new DescriptiveStatistics();
for (double value : timeSeriesData) {
stats.addValue(value);
}
double mean = stats.getMean();
double variance = stats.getVariance();
In this example, we utilize the Apache Commons Math library to perform descriptive statistics on time series data, showcasing how leveraging third-party libraries can enhance the capability of handling complex data operations in Java.
Lessons Learned
Java 8 Streams have undoubtedly transformed the way we process and manipulate data in Java. However, as applications grow in complexity, it becomes imperative to leverage advanced techniques and third-party libraries to handle intricate data operations effectively. By tapping into the power of Java 8 Comparator, Predicate, Collectors, and exploring third-party libraries, developers can elevate their proficiency in handling complex data operations, ultimately enhancing the robustness and efficiency of their Java applications.
In conclusion, the capabilities of Java 8 Streams, combined with advanced techniques and third-party libraries, enable developers to tackle complex data processing scenarios with sophistication and finesse.
For further reading on advanced Java data operations, you may find the following resources helpful:
Now, armed with a deeper understanding of advanced data operations in Java, it's time to elevate your data processing capabilities and build robust, efficient applications.