Master Filterer Pattern in 10 Steps: Revolutionize Searches
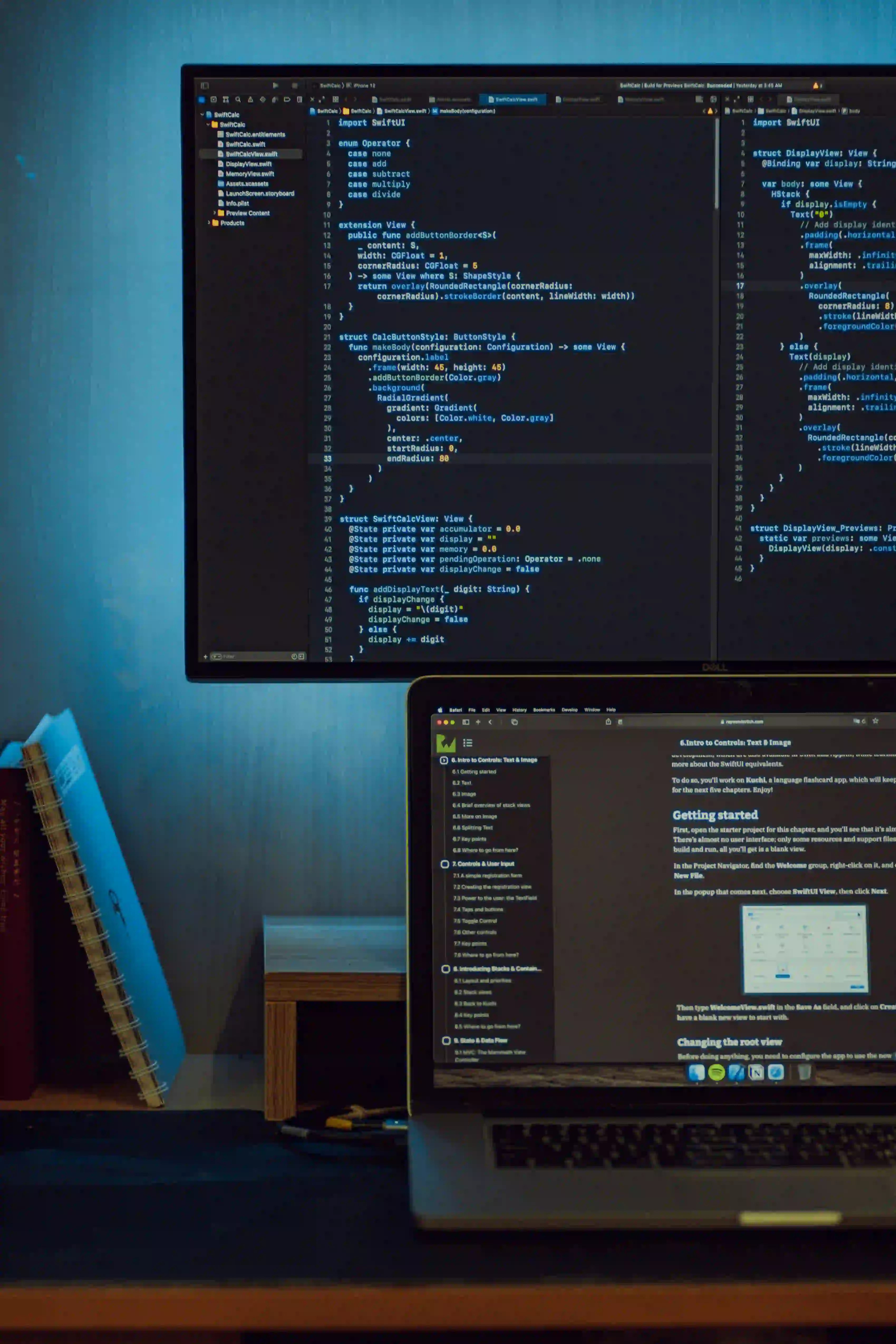
Master Filterer Pattern in 10 Steps: Revolutionize Searches
The filterer pattern is a powerful design pattern that simplifies and enhances the process of filtering data in Java applications. By following this pattern, developers can significantly improve the efficiency and maintainability of their code when dealing with search and filter operations.
In this tutorial, we'll explore the master filterer pattern in 10 actionable steps, and uncover how it can revolutionize the way searches are performed in Java. By the end of this guide, you'll be equipped with the knowledge and skills to implement the filterer pattern effectively in your own projects.
Step 1: Understand the Need
To begin with, it's essential to comprehend the need for a robust filtering mechanism in any application. Whether it's filtering a list of products based on multiple criteria in an e-commerce platform or refining search results in a database application, the ability to apply filters seamlessly is critical for a seamless user experience.
Step 2: Explore the Filterer Pattern
The filterer pattern is a structural design pattern that allows developers to define a set of criteria and apply them to a collection of objects. This pattern encapsulates the filtering logic, making it reusable and easy to maintain. By separating the filtering logic from the core functionality, the filterer pattern promotes code reusability and maintainability.
Step 3: Define Filter Criteria
Identify the specific criteria based on which the data needs to be filtered. This could include attributes such as name, price, category, or any other relevant properties based on the context of your application. Understanding the filter criteria is crucial for designing an effective filtering strategy.
public interface FilterCriteria<T> {
boolean meetsCriteria(T item);
}
In the above code snippet, we define a FilterCriteria
interface, which will serve as the contract for defining filter criteria.
Step 4: Implement Filterer
Create a Filterer
class responsible for applying the filter criteria to a collection of objects. This class encapsulates the filtering logic and provides methods to apply the criteria and retrieve the filtered results.
public class Filterer<T> {
public List<T> filter(List<T> items, FilterCriteria<T> criteria) {
// Apply the criteria and return the filtered results
}
}
By encapsulating the filtering logic within the Filterer
class, we ensure a clean separation of concerns and facilitate easy modifications or extensions to the filtering process.
Step 5: Create Concrete Filter Criteria
Implement concrete classes that represent specific filter criteria based on the defined filter criteria interface. For example, a PriceFilterCriteria
, NameFilterCriteria
, or CategoryFilterCriteria
can be created to encapsulate the individual filtering conditions.
Step 6: Apply Filterer Pattern
Integrate the Filterer
class and the concrete filter criteria into your application where filtering is required. Instantiate the Filterer
, create instances of the concrete filter criteria, and apply them to the collection of objects to obtain the filtered results.
Filterer<Product> productFilterer = new Filterer<>();
List<Product> filteredProducts = productFilterer.filter(allProducts, new PriceFilterCriteria(100, 200));
By following the filterer pattern, we can easily apply various filtering criteria without cluttering the core business logic with complex filter conditions.
Step 7: Handle Dynamic Filters
A powerful aspect of the filterer pattern is its ability to handle dynamic filtering requirements. By allowing the creation and application of filter criteria at runtime, the filterer pattern accommodates dynamic and evolving filtering needs without necessitating extensive code modifications.
Step 8: Reusability and Maintenance
One of the primary advantages of the filterer pattern is the reusability of filter criteria and the filterer class across different parts of the application. Additionally, maintaining and updating filtering logic becomes simpler and less error-prone due to the encapsulation of filtering concerns.
Step 9: Testing and Validation
Thoroughly test the filterer pattern implementation by applying various filter criteria and verifying the correctness of the filtered results. Additionally, ensure that the filterer handles edge cases and unexpected input gracefully.
Step 10: Refactor and Optimize
Continuously evaluate the filterer pattern implementation and identify opportunities for optimization. Refactor the code to enhance performance, readability, and maintainability, ensuring that the filterer pattern aligns with the evolving requirements of the application.
Lessons Learned
The master filterer pattern presents a powerful and elegant solution for handling data filtering in Java applications. By following the 10 steps outlined in this tutorial, you can revolutionize the way searches and filters are implemented, leading to cleaner, more maintainable, and flexible code.
By embracing the filterer pattern, developers can streamline the filtering process, promote code reusability, and adapt to changing filtering requirements with ease. The master filterer pattern truly empowers Java developers to revolutionize searches and filtering operations in their applications.
Start integrating the filterer pattern into your Java projects to experience its transformative impact on search and filter operations.
Remember, mastering the filterer pattern is a journey, and continual exploration and refinement will pave the way for its effective implementation in diverse real-world scenarios.
Give it a try, and witness the revolution in search and filter operations with the master filterer pattern!