Boost Performance: Overcome Slow Producers with Read-Behind Cache
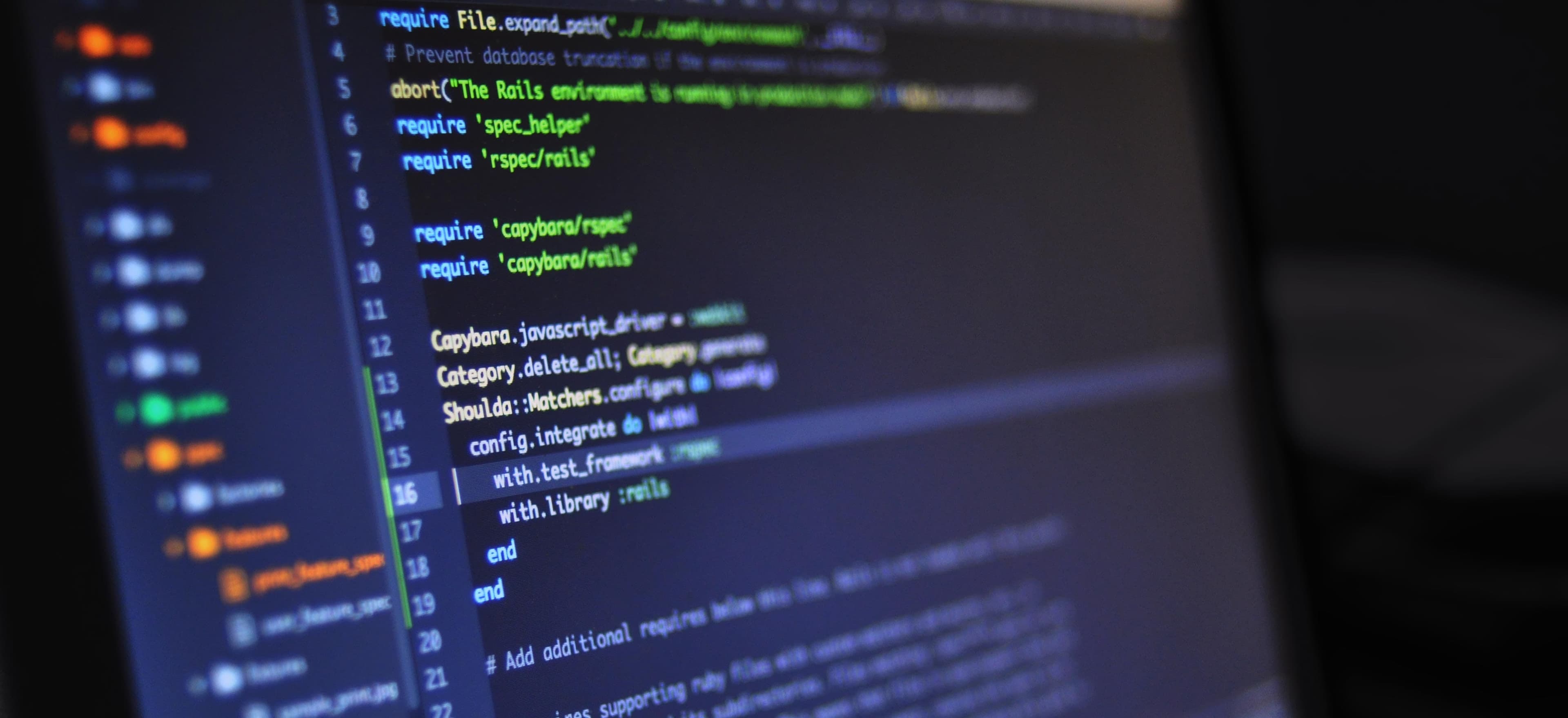
- Published on
Boost Performance: Overcome Slow Producers with Read-Behind Cache
In Java applications, it's common to encounter slow producers such as external APIs, databases, or file systems that can hinder overall performance. Slow response times from these resources can significantly impact the user experience. One way to mitigate this issue is by implementing a read-behind cache.
What is a Read-Behind Cache?
A read-behind cache, also known as a write-through cache with read-behind, is a caching technique that allows the application to continue its work while the data is being loaded into the cache in the background. When the cached data is requested, the application can retrieve it from the cache rather than waiting for the slow producer to respond. This approach can vastly improve performance and responsiveness.
Implementing a Read-Behind Cache in Java
Let's delve into the process of implementing a read-behind cache in a Java application. We'll use the Caffeine library, a high-performance caching library for Java, to demonstrate this.
Step 1: Add the Caffeine Dependency
Firstly, include the Caffeine library as a dependency in your pom.xml
file if you are using Maven:
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>3.0.0</version>
</dependency>
Or if you are using Gradle, add this to your build.gradle
:
implementation 'com.github.ben-manes.caffeine:caffeine:3.0.0'
Step 2: Create the Read-Behind Cache
Next, let's create the read-behind cache using Caffeine.
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.CompletableFuture;
public class SlowResourceCache {
private final LoadingCache<String, String> cache;
public SlowResourceCache() {
this.cache = Caffeine.newBuilder()
.maximumSize(1000)
.buildAsync(this::loadSlowResource);
}
private CompletableFuture<String> loadSlowResource(String key) {
// Code to fetch data from slow resource (e.g., external API, database, or file system)
// Return a CompletableFuture representing the asynchronous computation
}
public String get(String key) {
return cache.get(key, k -> CompletableFuture.completedFuture("default value"));
}
}
In this example, we create a SlowResourceCache
class that initializes a LoadingCache
from Caffeine. The buildAsync
method allows us to specify a function (loadSlowResource
) that will be asynchronously called to load the data into the cache when needed.
Step 3: Using the Read-Behind Cache
Now, let's see how we can use the SlowResourceCache
to access the slow resource while benefiting from the read-behind caching mechanism.
public class Main {
public static void main(String[] args) {
SlowResourceCache cache = new SlowResourceCache();
// Request the data, which will trigger read-behind caching
String key = "example_key";
String data = cache.get(key);
}
}
In this usage scenario, when cache.get(key)
is called, it will either return the cached data if it's available, or initiate the background loading of the data if it's not in the cache.
Benefits of Read-Behind Cache
The use of a read-behind cache offers several advantages:
Improved Performance
By initiating the loading of data in the background, the application does not have to wait for the slow producer to respond, leading to improved response times and overall performance.
Better User Experience
Faster response times result in a better user experience, as users are not kept waiting for data retrieval operations.
Reduced Load on Slow Producers
Slow producers are not overwhelmed with immediate simultaneous requests from the application, as the data can be loaded in the background and served from the cache when needed.
The Last Word
In conclusion, read-behind caching is a powerful technique in Java for mitigating the impact of slow data producers on application performance. By utilizing a read-behind cache, you can significantly enhance the responsiveness and efficiency of your application, resulting in a more satisfying user experience.
By adopting this approach and leveraging tools like the Caffeine library, you can effectively manage slow data producers and ensure optimal performance in your Java applications.