Cracking Enums: Modifying Final Static Fields Exposed!
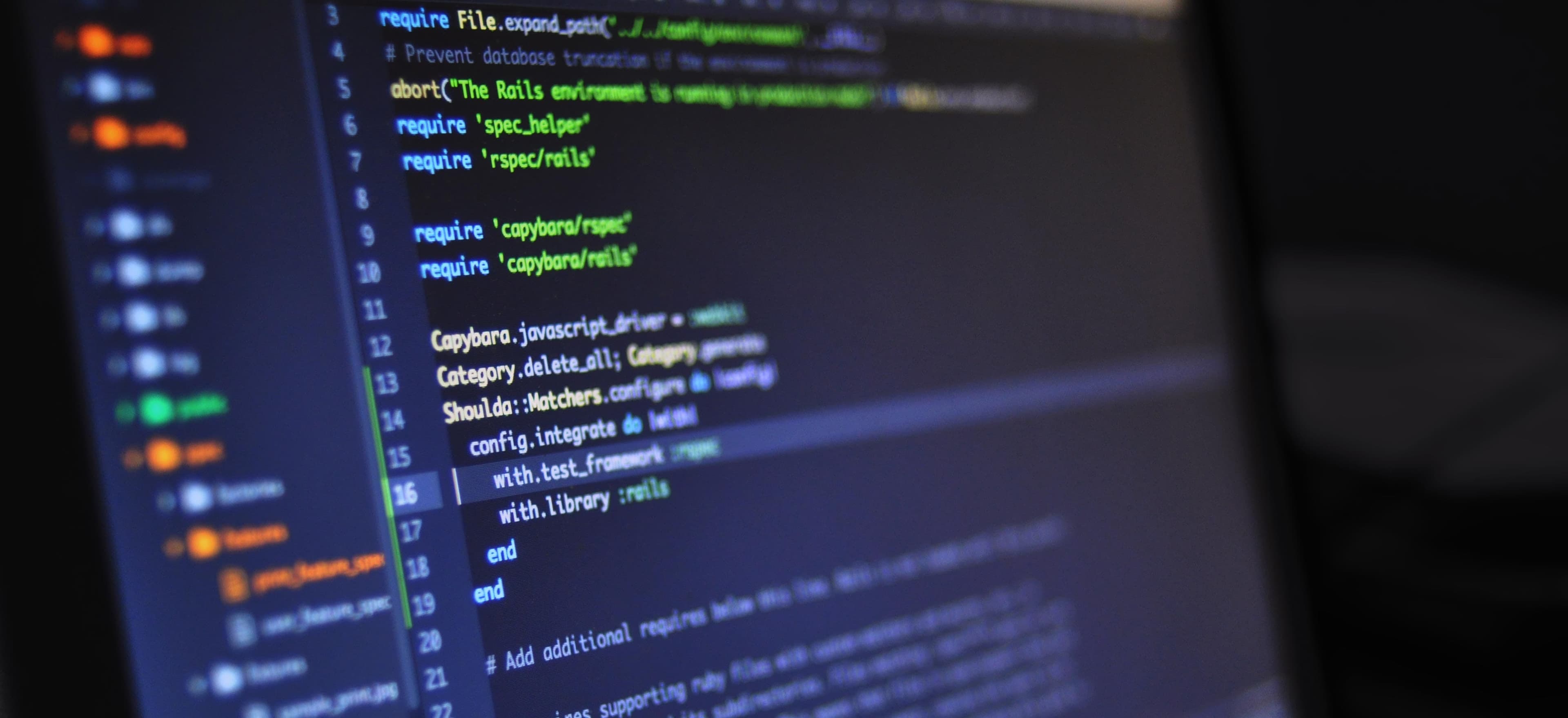
- Published on
Cracking Enums: Modifying Final Static Fields Exposed!
Enums have been a fundamental part of Java programming since Java 5. They provide a way to represent a fixed set of constants, making the code more readable and maintainable. Enums are often used in various design patterns and are a powerful tool in the hands of a Java developer. However, there's a common misconception that enum constants are immutable. In this blog post, we'll explore the potential risk of modifying final static fields in enums and understand how to mitigate this risk.
Understanding Enums in Java
In Java, enums are a special type of class that represents a group of constants (unchangeable variables, like final variables). Enum constants are implicitly static and final. It's the combination of static and final that makes enum constants appear immutable. Let's start by defining a simple enum to understand its basic structure:
public enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY;
}
In this example, Day
is an enum representing the days of the week. Each constant (SUNDAY, MONDAY, etc.) is implicitly declared as public, static, and final. This ensures that once the enum constants are created, their values cannot be changed.
The Myth of Immutable Enums
While the enum constants themselves are indeed immutable, the fields within the enum class can still be modified, provided proper precautions are not taken. Let's consider an example where an enum is used to represent configuration properties for different environments.
public enum Environment {
DEV("http://dev.example.com"),
QA("http://qa.example.com"),
PROD("http://prod.example.com");
private static String currentUrl;
public static void setCurrentUrl(String url) {
currentUrl = url;
}
public static String getCurrentUrl() {
return currentUrl;
}
private final String url;
Environment(String url) {
this.url = url;
}
public String getUrl() {
return url;
}
}
In this example, the Environment
enum has a currentUrl
field, which can be modified using the setCurrentUrl
method. This means that the supposedly immutable enum is no longer consistent across all its usages, which can lead to unexpected behavior in the application.
Mitigating the Risk
To prevent modification of enum fields, we can employ several strategies. One of the most effective approaches is to make defensive copies of mutable components. Let's update the Environment
enum to ensure that the currentUrl
field is properly encapsulated and remains immutable.
public enum Environment {
// previous enum constants remain unchanged
private final String url;
Environment(String url) {
this.url = url;
}
public String getUrl() {
return url;
}
// New method to set the URL by returning a new instance
public Environment withUrl(String url) {
return new Environment(url);
}
}
By introducing the withUrl
method, we ensure that any modification returns a new instance of the Environment
enum, leaving the original instance unchanged. This effectively prevents the modification of the enum's fields.
Bringing It All Together
While enums in Java provide a powerful way to define a fixed set of constants, it's crucial to understand that the immutability of enum constants does not extend to the entire enum class. By being aware of the potential risks and employing defensive coding practices, we can ensure that our enums remain consistent and predictable throughout our applications.
In this blog post, we've explored the myth of immutable enums, demonstrated the potential risk of modifying final static fields within enums, and discussed strategies to mitigate this risk. It's essential for Java developers to grasp the nuances of enums and adopt best practices to maintain the integrity of their code.
Understanding the nuances of enums and immutability is crucial in writing robust and maintainable Java code. By grasping the potential risks and employing defensive coding practices, we can ensure that our enums remain consistent and predictable throughout our applications.
As Java continues to evolve, it's important to stay updated on best practices and potential pitfalls. To delve deeper into Java enums and immutability, check out the official Java documentation.
Checkout our other articles