Master Hibernate: Keeping Your Table Histories Intact
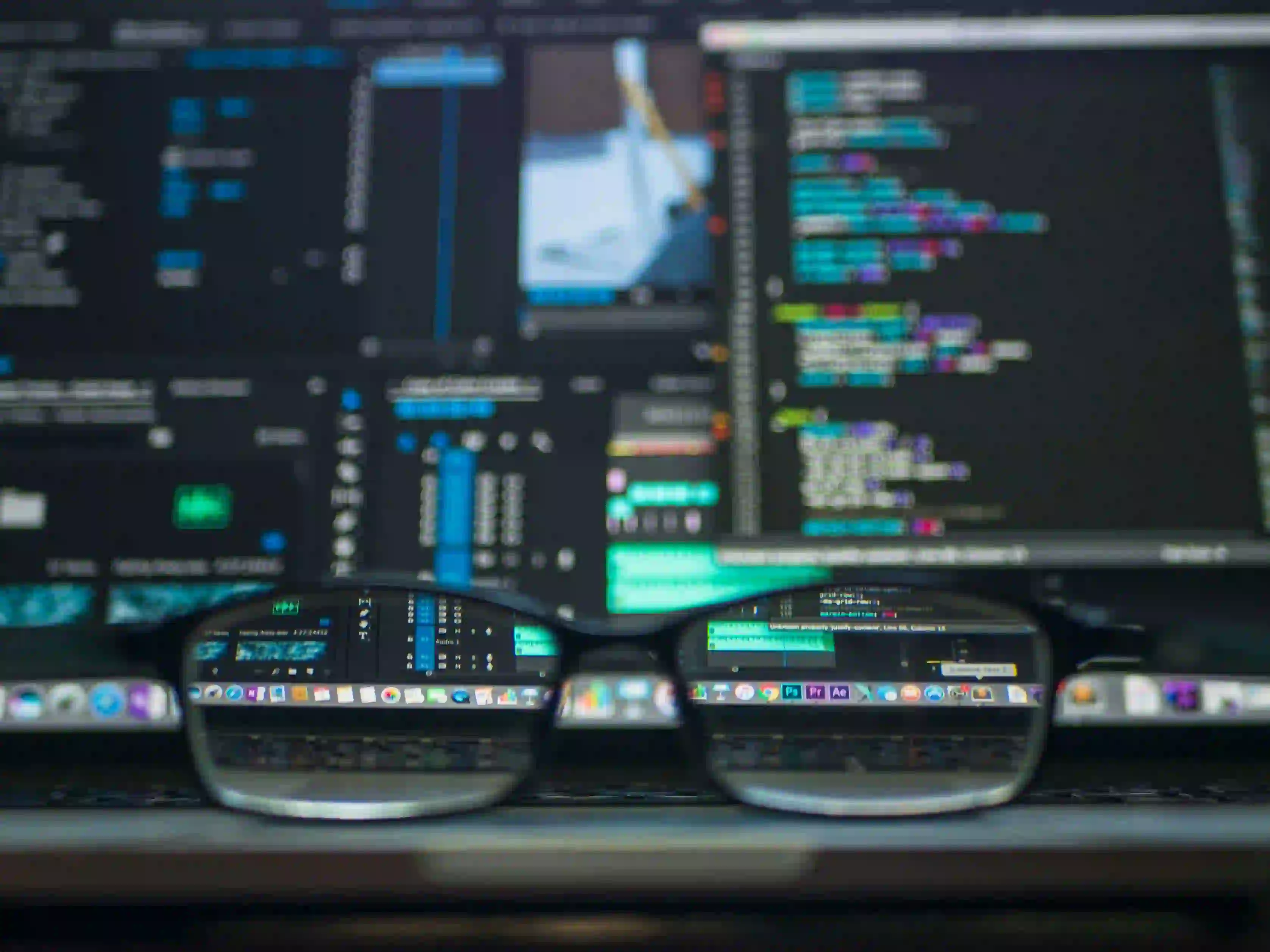
Master Hibernate: Keeping Your Table Histories Intact
In the world of relational databases, it's often crucial to maintain historical records of data changes. This ensures data integrity, aids in auditing, and provides a trail of how data has evolved over time. To achieve this in a Java application, Hibernate offers powerful tools and techniques for managing historical data effectively.
Why Manage Table Histories?
Imagine you have an application dealing with customer information. A customer changes their address, their order status gets updated, or their account details are modified. Without a historical record, you would lose the ability to track these changes over time. This is where managing table histories becomes indispensable.
Enter Hibernate Envers
Hibernate Envers is an extension to the popular Hibernate ORM framework, designed specifically to address the need for auditing and historical data management. It enables easy versioning of entities, tracking changes, and providing retrieval of historical data. Envers seamlessly integrates with Hibernate, making it a natural choice for historical data management in Java applications.
Getting Started with Hibernate Envers
To begin using Hibernate Envers, the first step is to include the necessary dependencies in your project. For a Maven project, simply add the following dependency to your pom.xml
:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-envers</artifactId>
<version>${hibernate.version}</version>
</dependency>
Replace ${hibernate.version}
with the appropriate version of Hibernate used in your project.
Annotating Entities for Auditing
Once the dependencies are in place, annotating your entities to enable auditing is straightforward. Let's consider a simple Customer
entity:
@Entity
@Audited
public class Customer {
@Id
@GeneratedValue
private Long id;
@Audited
private String name;
@Audited
private String address;
// Getters and setters
}
By adding the @Audited
annotation to the entity class and the audited fields, Hibernate Envers will automatically track the changes to these fields and maintain a history of all modifications.
Retrieving Historical Data
Retrieving historical data with Hibernate Envers is as simple as querying regular entities. The only difference is that you will be querying the historical versioned entities.
Customer customer = entityManager.find(Customer.class, customerId, revisionNumber);
Here, revisionNumber
refers to the specific revision at which you want to retrieve the entity. This allows you to access the data as it existed at a particular point in time, providing a complete view of historical changes.
Handling Auditing Annotations on Relations
When dealing with relationships between entities, it's important to note how auditing annotations are handled by Hibernate Envers.
Consider a scenario where a Customer
entity has a one-to-many relationship with an Order
entity.
@Entity
@Audited
public class Customer {
// ...
@OneToMany(mappedBy = "customer")
@Audited
private List<Order> orders;
// ...
}
By annotating the collection of orders with @Audited
, Hibernate Envers will track changes to the orders associated with a customer. This ensures that the historical data of related entities is also captured seamlessly.
Customizing Audit Tables
Hibernate Envers creates special audit tables to store historical information. These tables contain additional columns such as revision numbers, revision dates, and revision types to track changes effectively.
In some cases, you may need to customize the structure of these audit tables to align with specific requirements or naming conventions. This can be achieved by providing a custom revision entity and modifying the audit table schema to suit your needs.
Integrating Lifecycle Events
In addition to tracking attribute-level changes, Hibernate Envers supports integration with JPA lifecycle events to capture more granular audit information. This includes events such as entity creation, modification, and removal, providing a comprehensive audit trail of all data modifications.
Wrapping Up
Managing table histories is a critical aspect of application development, especially when dealing with sensitive or frequently changing data. Hibernate Envers simplifies the process by seamlessly integrating historical versioning into your existing Hibernate entities, allowing you to focus on building robust, auditable Java applications.
By harnessing the power of Hibernate Envers, you can maintain data integrity, meet compliance requirements, and gain valuable insights into the evolution of your data over time. Incorporating historical data management into your Java applications with Hibernate Envers is a significant step towards building reliable and accountable systems.
Mastering Hibernate Envers empowers Java developers to handle table histories with precision, ensuring that no data modifications are ever lost or unaccounted for.
To delve deeper into Hibernate Envers, consider exploring the official documentation at Hibernate Envers User Guide.
Start incorporating Hibernate Envers into your projects today, and elevate your data management capabilities to new heights!