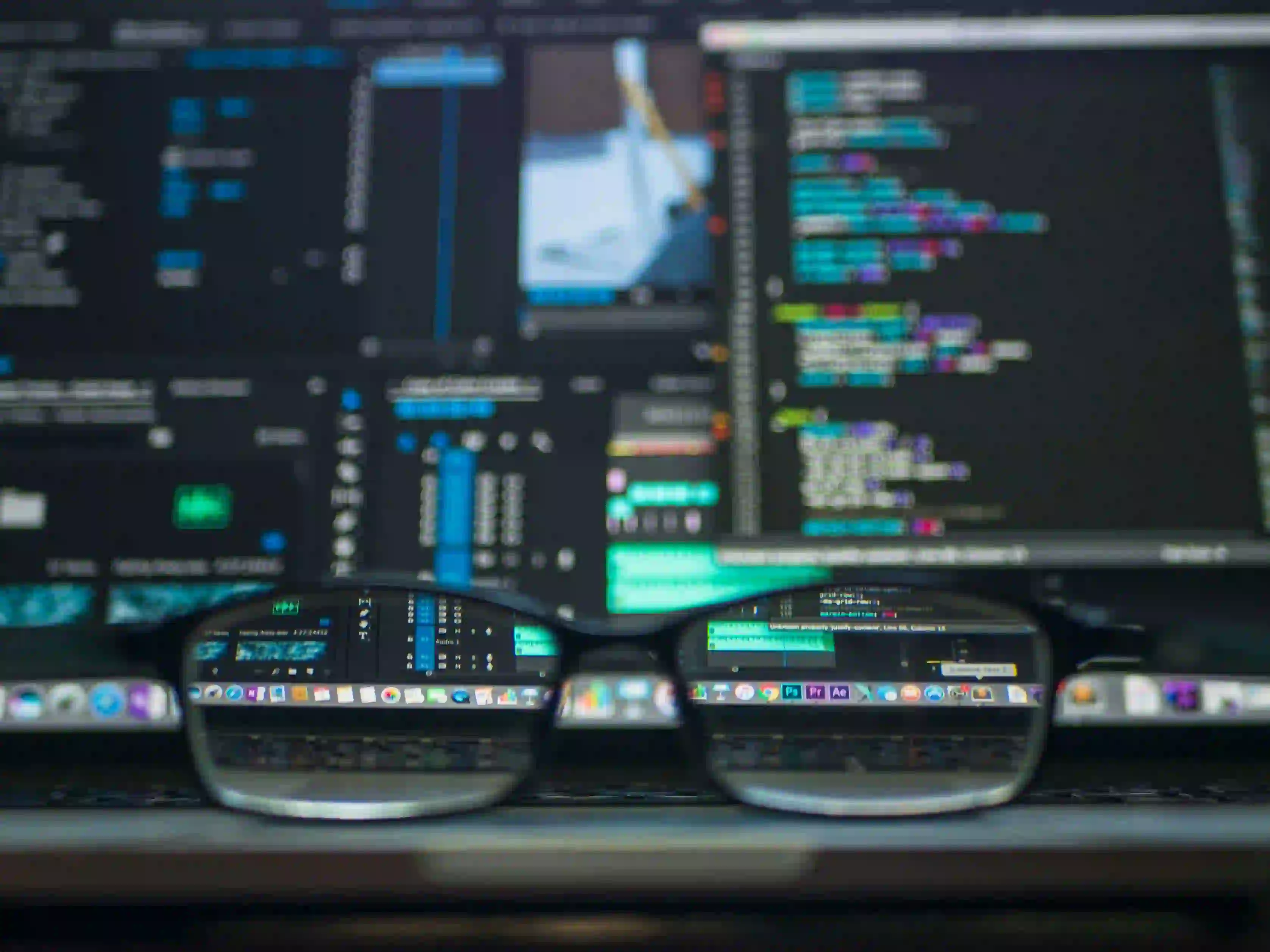
Mastering the Art of Integration Testing with Spring & Hibernate
In the world of Java development, the combination of Spring and Hibernate has become a powerful and popular choice for building robust and scalable applications. However, ensuring that the integration between these two frameworks works seamlessly can be a challenging task. This is where integration testing comes into play. In this article, we will delve into the realm of integration testing with Spring and Hibernate, learning how to master this art for creating reliable and high-quality applications.
What is Integration Testing?
Integration testing is a crucial phase in the software development lifecycle, where individual units of code are combined and tested as a group. It focuses on verifying the interactions between various modules of an application to ensure that they work together as expected. In the context of Spring and Hibernate, integration testing involves testing the collaboration between the Spring framework and Hibernate ORM for database interactions.
Why is Integration Testing Important?
Integration testing is essential for uncovering defects that arise from the interaction between different components of an application. It ensures that the entire system, including the database layer, works harmoniously. By simulating real-world scenarios, integration testing provides confidence in the overall behavior and performance of the application.
Setting the Stage: Spring & Hibernate Configuration
Before diving into integration testing, let's set up a basic configuration for Spring and Hibernate integration. In this example, we'll use Spring Boot to simplify the setup process.
Setting up the Maven Dependencies
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Configuring the Application Properties
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
spring.jpa.show-sql=true
In this configuration, we are using the H2 in-memory database for simplicity. In a real-world scenario, you would replace these settings with your actual database configuration.
Integration Testing with Spring & Hibernate
Now that we have the groundwork set, let's move on to integration testing. We will create a simple integration test that verifies the interaction between Spring Data JPA and Hibernate for data persistence.
Integrating Spring Data JPA and Hibernate
First, let's define an entity class using JPA annotations for mapping to a database table.
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String name;
@Column(nullable = false)
private BigDecimal price;
// Getters and setters
}
In this example, we have a Product
entity with attributes mapped to columns in a database table. The @Entity
and @Table
annotations define the mapping to the database table.
Creating a Repository Interface
Next, let's create a Spring Data JPA repository interface for the Product
entity.
public interface ProductRepository extends JpaRepository<Product, Long> {
Optional<Product> findByName(String name);
}
The ProductRepository
interface extends the JpaRepository
interface, providing out-of-the-box CRUD operations for the Product
entity. We also define a custom query method to retrieve a product by its name.
Writing the Integration Test
Now, let's write an integration test to ensure that the interaction between Spring Data JPA and Hibernate works correctly.
@RunWith(SpringRunner.class)
@SpringBootTest
@AutoConfigureTestDatabase
public class ProductRepositoryIntegrationTest {
@Autowired
private ProductRepository productRepository;
@Test
public void whenFindByName_thenReturnProduct() {
Product product = new Product("Laptop", new BigDecimal("1000"));
productRepository.save(product);
Optional<Product> found = productRepository.findByName("Laptop");
assertThat(found.isPresent()).isTrue();
assertThat(found.get().getName()).isEqualTo(product.getName());
assertThat(found.get().getPrice()).isEqualTo(product.getPrice());
}
}
In this integration test, we use the @RunWith(SpringRunner.class)
annotation to enable the Spring test runner. The @SpringBootTest
annotation indicates that the test should load the complete application context. The @AutoConfigureTestDatabase
annotation configures a test database for the test.
Within the test method, we save a Product
entity using the ProductRepository
, and then verify that we can retrieve the saved product by its name.
Wrapping Up
Mastering the art of integration testing with Spring and Hibernate is crucial for building reliable and maintainable applications. By testing the interaction between these frameworks, you can ensure that your application's database layer functions seamlessly with the rest of the system. Integration testing not only boosts confidence in the codebase but also contributes to the overall stability and performance of the application.
In conclusion, integration testing with Spring and Hibernate is an indispensable practice for Java developers aiming to deliver high-quality software. By mastering this art, you can elevate the reliability and robustness of your applications, consequently enhancing the overall user experience.
In your Java development journey, understanding and implementing integration testing with Spring and Hibernate will undoubtedly set you on the path to becoming a proficient and sought-after developer.
To delve deeper into the intricacies of integration testing with Spring and Hibernate, consider exploring the official documentation for Spring Framework and Hibernate. These resources provide comprehensive insights and best practices for honing your integration testing skills.
Happy coding!