Mastering JAX-RS: Navigating Exceptions & Status Codes
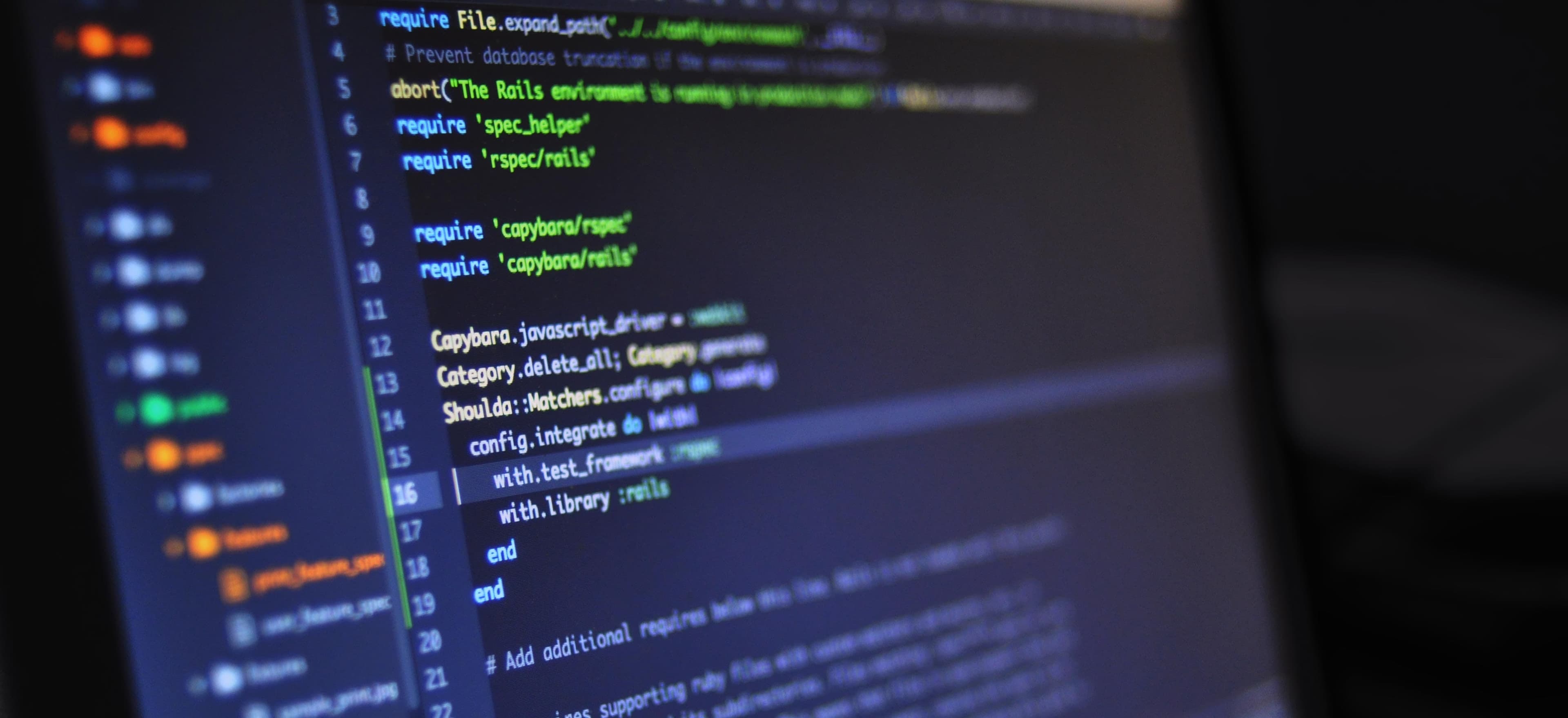
- Published on
Mastering JAX-RS: Navigating Exceptions & Status Codes
JAX-RS, or Java API for RESTful Web Services, is a powerful tool for building robust and scalable web applications using the REST architecture. Exception handling and status codes are critical components of any web API as they help communicate the outcome of API requests and enable clients to react accordingly. In this post, we will delve into the world of JAX-RS exceptions and status codes, understanding their importance, and how to effectively utilize them in your applications.
Understanding Status Codes
HTTP status codes are an integral part of the HTTP protocol, providing valuable information about the outcome of a client's request. Status codes are three-digit integers that are part of the response from the server to the client. They are grouped into different categories, each indicating a specific type of response. Some common status code categories include:
- 2xx: Success
- 4xx: Client Error
- 5xx: Server Error
When building a RESTful API using JAX-RS, it's crucial to use the appropriate status codes to convey the result of an operation. For example, when a resource is successfully created, the server should respond with a status code of 201 (Created). On the other hand, if a resource is not found, the server should respond with a 404 (Not Found) status code.
Handling Exceptions in JAX-RS
Exception handling in JAX-RS plays a pivotal role in providing meaningful error responses to clients. When an exception occurs during the processing of a request, it needs to be handled appropriately and translated into a relevant HTTP status code.
In JAX-RS, exceptions can be mapped to specific HTTP status codes using the @Provider
annotation along with implementing the ExceptionMapper
interface. By doing this, developers can customize the mapping of exceptions to status codes and provide informative error messages to the client.
@Provider
public class CustomExceptionMapper implements ExceptionMapper<CustomException> {
@Override
public Response toResponse(CustomException ex) {
return Response.status(Response.Status.NOT_FOUND)
.entity("Resource Not Found")
.build();
}
}
In the above example, the CustomExceptionMapper
handles instances of CustomException
and maps them to a 404 (Not Found) status code with a corresponding error message. This allows for consistent and clear communication of errors to the API clients.
Using Status Codes and Exceptions in Practice
Let’s explore a practical example of utilizing status codes and exceptions in a JAX-RS application. Suppose we have a resource endpoint for retrieving user details by their ID. If the user is not found, we want to respond with a 404 status code.
@Path("/users")
public class UserResource {
@GET
@Path("/{id}")
public Response getUserById(@PathParam("id") String id) {
User user = userService.getUserById(id);
if (user == null) {
throw new UserNotFoundException("User not found");
}
return Response.ok(user).build();
}
}
In the above code, if the user is not found, a UserNotFoundException
is thrown, which triggers the UserNotFoundExceptionMapper
to map it to a 404 status code, resulting in a clear and consistent error response for the client.
Best Practices for Status Codes and Exceptions
When working with status codes and exceptions in JAX-RS, there are several best practices to keep in mind:
1. Use Standard Status Codes
Prefer using standard HTTP status codes to ensure interoperability and understanding across different systems. For example, use 400 (Bad Request) for client input validation errors and 500 (Internal Server Error) for unexpected server errors.
2. Provide Descriptive Error Messages
Along with status codes, include informative error messages in the response entity to assist the client in understanding the cause of the error. This can greatly aid in troubleshooting and debugging for developers using your API.
3. Leverage Exception Hierarchy
Utilize the exception hierarchy effectively to create specific exception classes for different error scenarios. This allows for granular exception handling and precise mapping to appropriate status codes.
4. Centralize Exception Handling
Centralize exception handling and mapping to status codes by using the @Provider
annotation and implementing custom ExceptionMapper
classes. This promotes consistency and ease of maintenance across the application.
The Closing Argument
In the world of JAX-RS, mastering exceptions and status codes is essential for building reliable and developer-friendly web APIs. By understanding the significance of status codes, effectively handling exceptions, and adhering to best practices, developers can enhance the overall usability and robustness of their RESTful services. Remember to use standard status codes, provide clear error messages, and leverage exception mapping to create a cohesive and informative API experience for clients.
Incorporating these techniques into your JAX-RS applications will not only improve error handling but also contribute to establishing a more resilient and predictable interaction between your APIs and their consumers.
Now that we've uncovered the vital role of status codes and exception handling in JAX-RS, it's time to put this knowledge into practice and elevate the quality of your RESTful services.