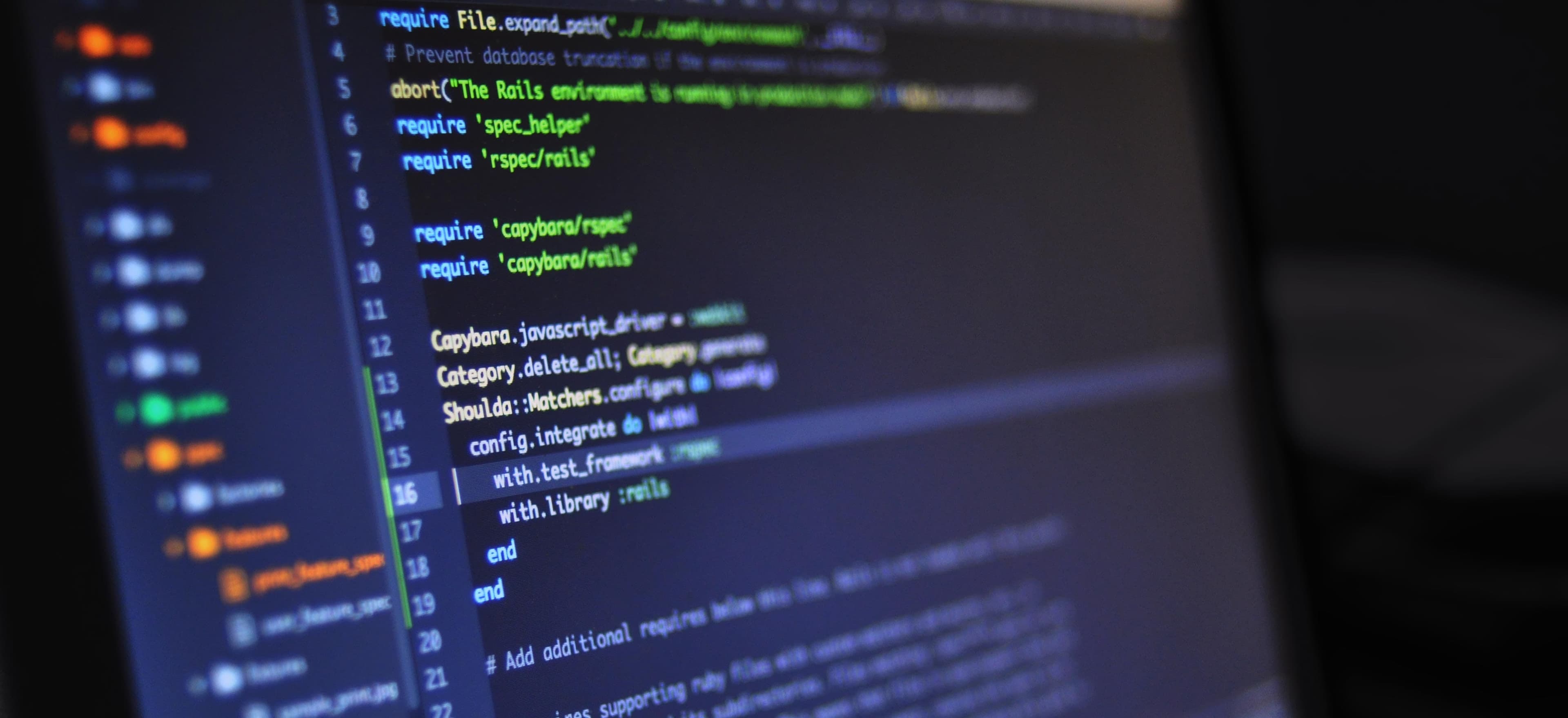
- Published on
Mastering Scala: Tips to Navigate Tuples and Lists
Scala is a powerful programming language that is known for its concise syntax and functional programming capabilities. One of the key features of Scala is its robust support for tuples and lists, which are fundamental data structures widely used in functional programming. In this blog post, we will delve into some advanced tips and techniques to effectively navigate and manipulate tuples and lists in Scala.
Understanding Tuples in Scala
Tuples in Scala are immutable, can hold objects with different data types, and are of fixed sizes. They are a great way to group related data together. Let's explore some tips for working with tuples in Scala.
Tip 1: Destructuring Tuples
Destructuring is an elegant feature in Scala that allows you to extract individual elements from a tuple. This can be achieved using pattern matching as shown below:
val t = (1, "scala")
val (num, str) = t
println(num) // Output: 1
println(str) // Output: scala
Using pattern matching simplifies the process of accessing individual elements of a tuple, making the code more readable and concise.
Tip 2: Tuple Concatenation
Scala provides a simple way to concatenate tuples using the ++
operator. This can be handy when you need to combine multiple tuples into a single tuple.
val t1 = (1, "scala")
val t2 = (2, "tuples")
val concatenatedTuple = t1 ++ t2
println(concatenatedTuple) // Output: (1, scala, 2, tuples)
The above code demonstrates how to concatenate two tuples using the ++
operator, resulting in a new tuple containing elements from both input tuples.
Manipulating Lists in Scala
Lists are another essential data structure in Scala, offering a range of operations for functional programming. Let's explore some advanced tips for working with lists in Scala.
Tip 1: Flattening Lists
In Scala, flattening a list means to concatenate all the sub-lists within the main list to create a single list. This can be achieved using the flatten
method. Here's an example:
val listOfLists = List(List(1, 2), List(3, 4))
val flattenedList = listOfLists.flatten
println(flattenedList) // Output: List(1, 2, 3, 4)
The flatten
method simplifies the process of flattening nested lists, providing a clean and concise approach to dealing with hierarchical data.
Tip 2: Zipping Lists
Zipping in Scala refers to combining corresponding elements from multiple lists into pairs. This can be accomplished using the zip
method. Consider the following example:
val list1 = List(1, 2, 3)
val list2 = List("a", "b", "c")
val zippedList = list1.zip(list2)
println(zippedList) // Output: List((1,a), (2,b), (3,c))
The zip
method allows for easy pairwise combination of elements from two lists, offering a convenient way to process related data in parallel.
Bringing It All Together
Mastering tuples and lists is essential for leveraging the full power of functional programming in Scala. By understanding the advanced tips and techniques discussed in this post, you can enhance your productivity and efficiency when working with tuples and lists. Stay tuned for more Scala insights and best practices!
For further readings on Scala and functional programming, check out the following resources:
Happy coding!