Delta Architecture: Bridging Lambda with Storm for Hadoop
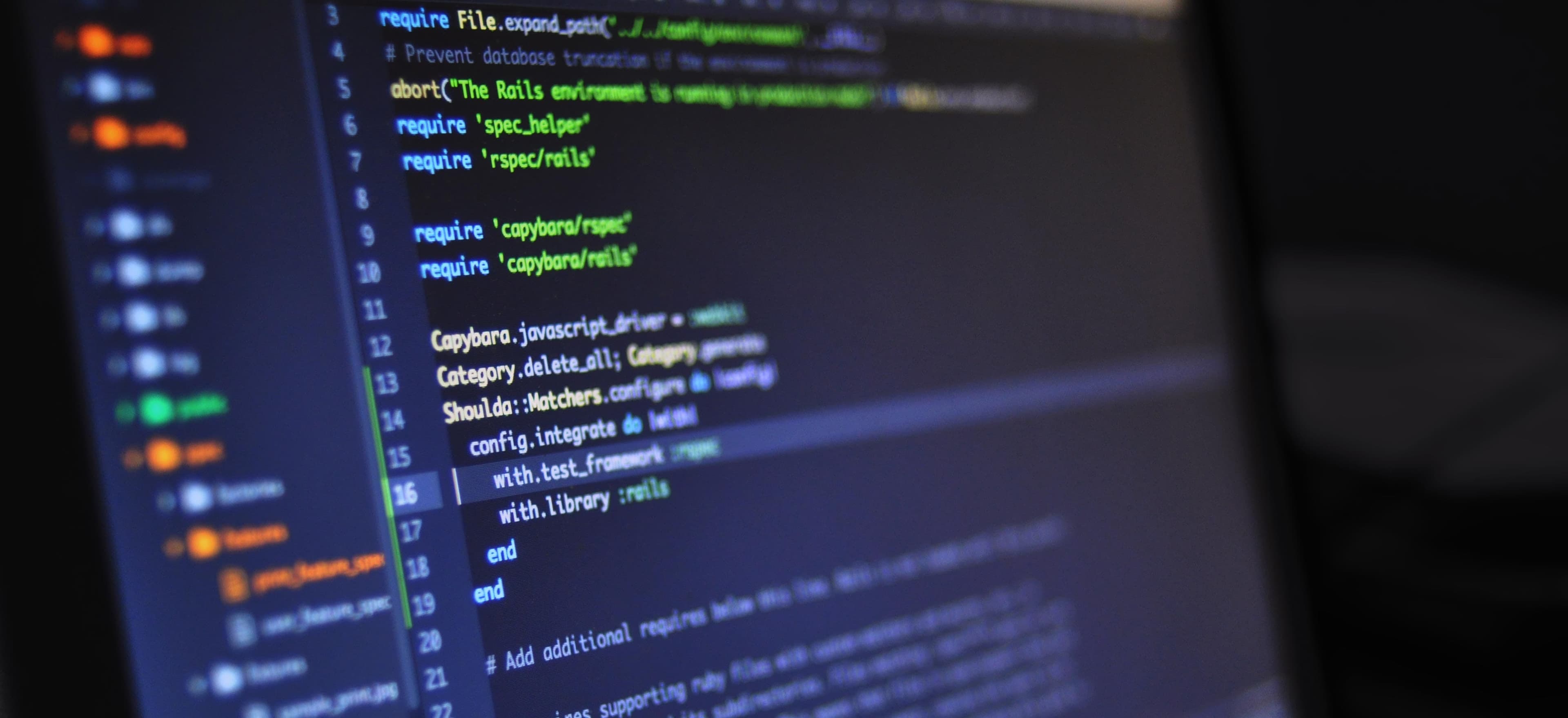
- Published on
Bridging Lambda with Storm for Hadoop: The Delta Architecture
In the world of big data processing, it is essential to have a well-structured architecture that allows for real-time and batch processing. The Delta Architecture, also known as the Lambda Architecture, is a powerful concept that combines batch processing with stream processing to handle massive amounts of data. In this blog post, we'll explore how to bridge the Delta Architecture with Apache Storm for real-time processing on Hadoop, using Java.
Understanding the Delta Architecture
The Delta Architecture is a versatile approach to big data processing, combining the strengths of both batch and real-time processing. It solves the problem of processing both historical and real-time data by using a three-layer architecture:
-
Batch Layer: This layer is responsible for processing large volumes of data at regular intervals. It computes arbitrary functions on the entire data set to generate batch views.
-
Speed Layer: This layer deals with processing real-time data streams and producing real-time views.
-
Serving Layer: This layer merges the batch views and real-time views to provide a unified view for querying.
Introducing Apache Storm
Apache Storm is a distributed real-time computation system that makes it easy to reliably process unbounded streams of data. It is designed to work with any queuing and database system and can process a massive amount of data in real-time.
Bridging the Gap with Java
Now, let's dive into how we can bridge the Delta Architecture with Apache Storm for Hadoop using Java. We'll focus on building a simple real-time data processing application that leverages the power of both Storm and Hadoop.
Setting Up the Environment
First, make sure you have Apache Storm and Hadoop installed and configured on your system. You can download Apache Storm from the official website and follow the installation instructions. For Hadoop, you can refer to the Hadoop documentation for installation and setup.
Building the Real-Time Processing Topology
In Apache Storm, a topology is the overall calculation that needs to be performed on the real-time data streams. It consists of a network of spouts and bolts that process the data. Let's create a simple topology for word count using Java.
public class WordCountTopology {
public static void main(String[] args) {
TopologyBuilder builder = new TopologyBuilder();
builder.setSpout("word-reader", new WordReaderSpout());
builder.setBolt("word-normalizer", new WordNormalizerBolt())
.shuffleGrouping("word-reader");
builder.setBolt("word-counter", new WordCounterBolt())
.fieldsGrouping("word-normalizer", new Fields("word"));
Config config = new Config();
config.setDebug(true);
LocalCluster cluster = new LocalCluster();
cluster.submitTopology("word-count", config, builder.createTopology());
Utils.sleep(10000);
cluster.killTopology("word-count");
cluster.shutdown();
}
}
In this code snippet, we define a simple topology with three components: a spout to read words, a bolt to normalize the words, and a bolt to count the words.
Creating Spouts and Bolts
In Apache Storm, spouts are responsible for reading data from external sources, while bolts are the components that process the data. Let's take a look at the implementations of the spout and bolts used in the WordCountTopology.
WordReaderSpout
public class WordReaderSpout extends BaseRichSpout {
// Implement the methods for emitting tuples and handling acks and fails
// ...
}
WordNormalizerBolt
public class WordNormalizerBolt extends BaseBasicBolt {
@Override
public void execute(Tuple input, BasicOutputCollector collector) {
// Normalize the word and emit it
// ...
}
@Override
public void declareOutputFields(OutputFieldsDeclarer declarer) {
// Declare the output fields
// ...
}
}
WordCounterBolt
public class WordCounterBolt extends BaseBasicBolt {
Map<String, Integer> counts = new HashMap<>();
@Override
public void execute(Tuple input, BasicOutputCollector collector) {
// Count the words and emit the counts
// ...
}
@Override
public void declareOutputFields(OutputFieldsDeclarer declarer) {
// Declare the output fields
// ...
}
}
Integrating with Hadoop for Batch Processing
Now that we have the real-time processing set up with Apache Storm, we can integrate it with Hadoop for batch processing. Hadoop's MapReduce framework is ideal for processing large-scale data sets in batch mode.
Writing Hadoop MapReduce Jobs in Java
Let's create a simple MapReduce program to perform word count in batch mode using Hadoop and Java.
First, define the Mapper class:
WordCountMapper
public class WordCountMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// Tokenize the input text and emit the word and count
// ...
}
}
Next, define the Reducer class:
WordCountReducer
public class WordCountReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
// Aggregate the counts for each word
// ...
}
}
Running the MapReduce Job
To run the MapReduce job, package the MapReduce program into a JAR file and submit it to the Hadoop cluster using the following command:
hadoop jar WordCount.jar input_path output_path
The Bottom Line
In this blog post, we've explored the Delta Architecture, bridging the strengths of batch processing with real-time processing using Apache Storm and Hadoop. By leveraging both technologies and integrating them with Java, we can build robust and scalable big data processing solutions. The combination of the Delta Architecture, Apache Storm, and Hadoop opens up a world of possibilities for handling large volumes of data in real-time and batch modes.
With a solid understanding of the concepts and a hands-on approach to building the necessary components, you are well-equipped to implement the Delta Architecture with Apache Storm for Hadoop in your own projects. Happy processing!
Remember, building scalable and efficient big data solutions requires deep expertise. If you have questions or need professional assistance with your big data projects, don't hesitate to reach out to our team at OurBigDataExperts.com.
Now, go ahead and unleash the power of the Delta Architecture with Apache Storm for Hadoop using Java!
Checkout our other articles