Revolutionizing Design: Strategy Pattern with CDI & Lambdas
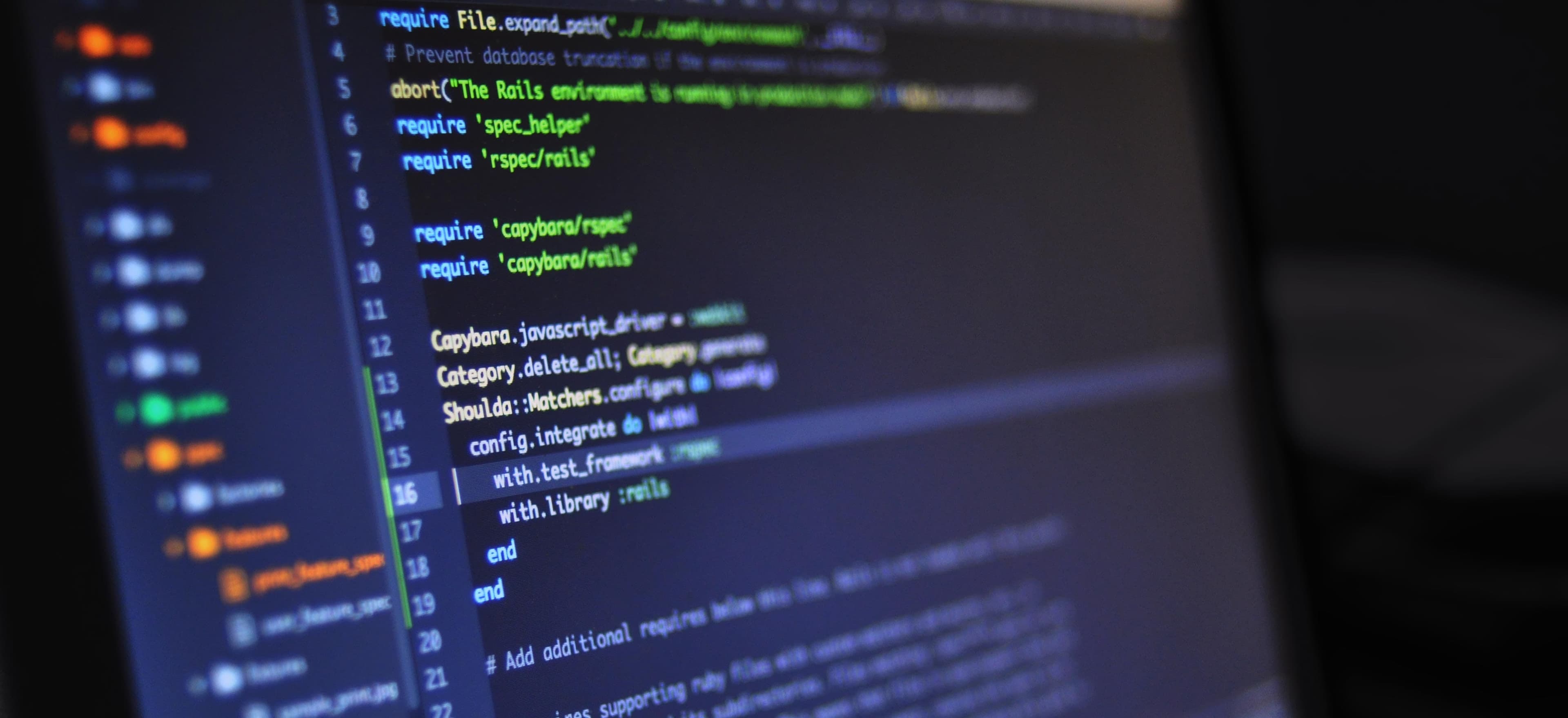
- Published on
Revolutionizing Design: Strategy Pattern with CDI & Lambdas
In modern Java development, the adoption of design patterns and functional programming paradigms has become increasingly prevalent. The Strategy Pattern is a classic design pattern that enables the encapsulation of algorithms, allowing them to be selected at runtime. With the advent of Contexts and Dependency Injection (CDI) in Java EE and lambdas in Java 8, the implementation of the Strategy Pattern has been revolutionized.
In this blog post, we will delve into how we can leverage CDI and lambdas to implement the Strategy Pattern in Java, creating a flexible and clean solution for algorithm selection. We will explore how these modern features of Java have reshaped the way we approach design patterns and functional programming. Let's dive in!
Understanding the Strategy Pattern
The Strategy Pattern is a behavioral design pattern that defines a family of algorithms, encapsulates each one of them, and makes them interchangeable. It allows the algorithm to vary independently from clients that utilize it. This pattern consists of three main components:
- Strategy Interface: Defines a common interface for a family of algorithms.
- Concrete Strategies: Encapsulate the implementation of individual algorithms.
- Context: Utilizes the strategy interface to execute the algorithm.
The flexibility and dynamic nature of the Strategy Pattern make it a valuable tool for managing algorithms in a variety of scenarios.
Traditional Implementation
Historically, the implementation of the Strategy Pattern in Java involved creating separate classes for each concrete strategy, often resulting in an excessive number of tiny classes. With the advent of lambdas and functional interfaces in Java 8, the verbosity of this traditional implementation can be significantly reduced.
public interface PaymentStrategy {
void pay(int amount);
}
public class CreditCardPaymentStrategy implements PaymentStrategy {
@Override
public void pay(int amount) {
// Payment implementation using credit card
}
}
public class PayPalPaymentStrategy implements PaymentStrategy {
@Override
public void pay(int amount) {
// Payment implementation using PayPal
}
}
public class PaymentContext {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void executePayment(int amount) {
paymentStrategy.pay(amount);
}
}
In this traditional implementation, each payment strategy is represented by a separate class. The context then utilizes the selected strategy to execute the payment. While this approach is effective, it can lead to a proliferation of small classes for each strategy.
Leveraging CDI for Strategy Selection
The introduction of Contexts and Dependency Injection (CDI) in Java EE provides a powerful mechanism for decoupling components and managing their lifecycle. By combining CDI with the Strategy Pattern, we can achieve a more elegant and flexible solution for selecting and utilizing strategies.
We can use the @Qualifier
annotation in combination with custom annotations to differentiate between different strategy implementations.
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.TYPE})
@Qualifier
public @interface PaymentMethod {
String value();
}
public class CreditCardPaymentStrategy implements PaymentStrategy {
@Override
public void pay(int amount) {
// Payment implementation using credit card
}
}
public class PayPalPaymentStrategy implements PaymentStrategy {
@Override
public void pay(int amount) {
// Payment implementation using PayPal
}
}
In this example, we created a @PaymentMethod
annotation to qualify the different payment strategies. Now, we can leverage CDI to inject the appropriate strategy based on the chosen payment method.
public class PaymentContext {
@Inject
@PaymentMethod("creditCard")
private PaymentStrategy creditCardPaymentStrategy;
@Inject
@PaymentMethod("paypal")
private PaymentStrategy payPalPaymentStrategy;
public void executePayment(int amount, String paymentMethod) {
switch (paymentMethod) {
case "creditCard":
creditCardPaymentStrategy.pay(amount);
break;
case "paypal":
payPalPaymentStrategy.pay(amount);
break;
default:
// Handle unsupported payment method
}
}
}
With CDI and custom annotations, the selection and injection of different strategies have become more streamlined. The @PaymentMethod
annotation serves as a qualifier, allowing us to easily choose the appropriate strategy at runtime.
Embracing Lambdas for Conciseness
The introduction of lambdas in Java 8 has transformed the way we write code, especially when working with functional interfaces. By utilizing lambdas, the verbosity associated with implementing functional interfaces has been significantly reduced. Let's see how lambdas can be leveraged to further streamline our Strategy Pattern implementation.
public class PaymentContext {
private Map<String, Consumer<Integer>> paymentStrategies = new HashMap<>();
public PaymentContext() {
paymentStrategies.put("creditCard", this::creditCardPayment);
paymentStrategies.put("paypal", this::payPalPayment);
}
public void executePayment(int amount, String paymentMethod) {
paymentStrategies.getOrDefault(paymentMethod, this::unsupportedPaymentMethod).accept(amount);
}
private void creditCardPayment(int amount) {
// Payment implementation using credit card
}
private void payPalPayment(int amount) {
// Payment implementation using PayPal
}
private void unsupportedPaymentMethod(int amount) {
// Handle unsupported payment method
}
}
In this refactored implementation, we leverage a Map
to associate each payment method with a corresponding lambda expression. This approach not only reduces the number of classes but also provides a more compact and concise representation of the Strategy Pattern.
Benefits of Modernizing Strategy Pattern Implementation
The integration of CDI and lambdas into the implementation of the Strategy Pattern offers several benefits:
- Reduced Boilerplate Code: CDI and custom annotations simplify the selection and injection of strategies, reducing the need for boilerplate code.
- Conciseness: Lambdas enable a more concise representation of strategy implementations, reducing the verbosity associated with traditional class-based strategies.
- Flexibility and Maintainability: The combination of modern Java features provides a flexible and maintainable solution for managing algorithms and their selection.
In Conclusion, Here is What Matters
The Strategy Pattern, coupled with the capabilities of CDI and lambdas, has evolved to meet the demands of modern Java development. By embracing these features, we can create elegant, flexible, and maintainable solutions for algorithm selection and execution. The traditional implementation of the Strategy Pattern has been reimagined, paving the way for more streamlined and expressive code.
In this blog post, we've explored how CDI simplifies strategy selection and injection, and how lambdas reduce verbosity and enhance conciseness in our implementations. By adopting these modern techniques, we can revolutionize the way we approach design patterns and functional programming in Java.
By combining the power of CDI, lambdas, and the Strategy Pattern, we can elevate the design and implementation of algorithms, paving the way for more efficient and elegant solutions in modern Java applications.
Experience the power of modern Java features by embracing the evolution of the Strategy Pattern, and unlock the potential for cleaner, more flexible, and maintainable code in your projects.
References:
- Contexts and Dependency Injection (CDI)
- Java SE 8: Lambda Quick Start
- Design Patterns: Elements of Reusable Object-Oriented Software
Are you ready to revolutionize your design patterns with CDI and lambdas? Let me know your thoughts in the comments below!