Unlocking Java 15: Mastering Sealed Classes Simplified
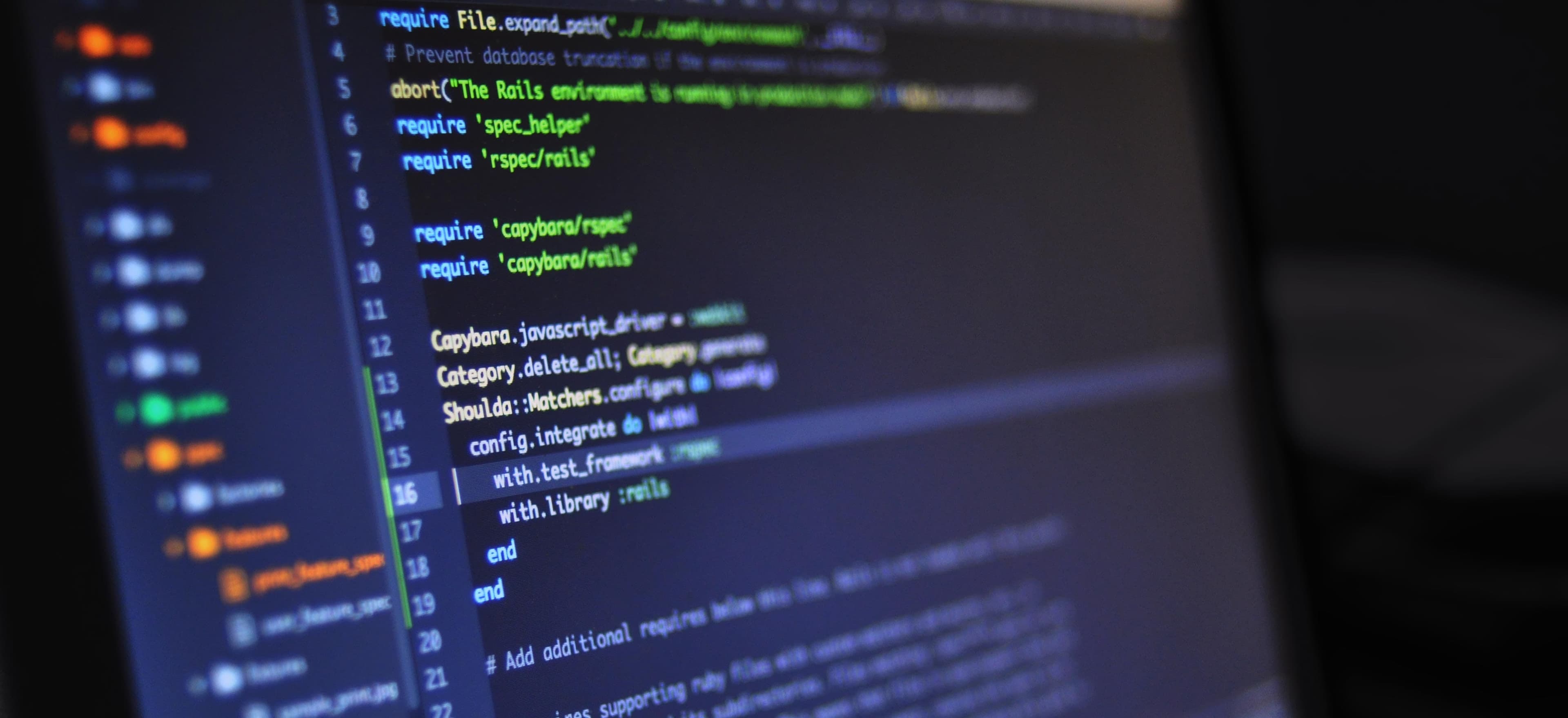
- Published on
Unlocking Java 15: Mastering Sealed Classes Simplified
Java 15 introduces a powerful new feature called sealed classes, which allows developers to restrict which classes can extend or implement a given class or interface. Sealed classes bring enhanced control over the inheritance hierarchy, providing a way to declare a limited set of classes that can extend a particular class. In this post, we will explore the concept of sealed classes, discuss their benefits, and provide practical examples to showcase their usage.
Understanding Sealed Classes
Sealed classes in Java are declared using the sealed
modifier, which must be placed before the class
or interface
keyword. Additionally, a list of permitted subtypes that can directly extend or implement the sealed class or interface is specified using the permits
clause. This effectively restricts the extensions to only those classes listed in the permits
clause.
Let's delve into a practical example to better understand the concept:
public sealed class Shape
permits Circle, Square, Triangle {
// class definition
}
In this example, the Shape
class is declared as sealed and specifies Circle
, Square
, and Triangle
as permitted subtypes.
Benefits of Sealed Classes
Sealed classes provide several benefits, including:
Enhanced Code Predictability
By restricting the inheritance hierarchy to a defined set of classes, sealed classes enhance the predictability of the codebase. This ensures that only a specific set of classes can extend the sealed class, leading to a more manageable and robust codebase.
Improved Security
Sealed classes can be particularly useful in scenarios where security and access control are critical. By explicitly defining the permitted subtypes, sealed classes offer an additional layer of control over the code, preventing unauthorized external extensions.
Facilitated API Design
In the context of API design, sealed classes enable developers to clearly communicate the intended inheritance structure. This can lead to more intuitive and understandable APIs, as the permitted subtypes are explicitly declared within the sealed class definition.
Practical Implementation
Let's explore a concrete example to demonstrate the practical implementation of sealed classes.
Suppose we have a base class Employee
that we want to seal and permit only specific types of employees to extend it: FullTimeEmployee
and PartTimeEmployee
. Here's how we can achieve this:
public sealed class Employee permits FullTimeEmployee, PartTimeEmployee {
// class definition
}
In this example, the Employee
class is declared as sealed and explicitly permits only FullTimeEmployee
and PartTimeEmployee
as its subtypes.
Now, let's consider the implementation of the permitted subtypes:
public final class FullTimeEmployee extends Employee {
// class definition
}
public final class PartTimeEmployee extends Employee {
// class definition
}
By specifying the permitted subtypes within the sealed class, we ensure that the inheritance hierarchy is restricted to the defined classes, providing a clear and controlled structure.
The Closing Argument
In conclusion, sealed classes in Java 15 offer a powerful mechanism for controlling the inheritance hierarchy, enhancing code predictability, security, and API design. By explicitly declaring the permitted subtypes, developers can enforce a clear and restricted structure within their codebase, leading to more maintainable and secure applications.
Sealed classes represent a valuable addition to the Java language, providing developers with a versatile tool for managing class hierarchies and refining API designs. With their ability to enforce strict constraints on class extensions, sealed classes empower developers to create robust, predictable, and secure codebases.
To delve deeper into Java 15 and its features, such as sealed classes, check out the official documentation on OpenJDK's official website. Learning and mastering these new features will undoubtedly enhance your proficiency as a Java developer.
Start leveraging the power of sealed classes in your Java projects and unlock a new level of control and predictability in your code!