Avoiding Common Pitfalls with Java 8's Filter Method
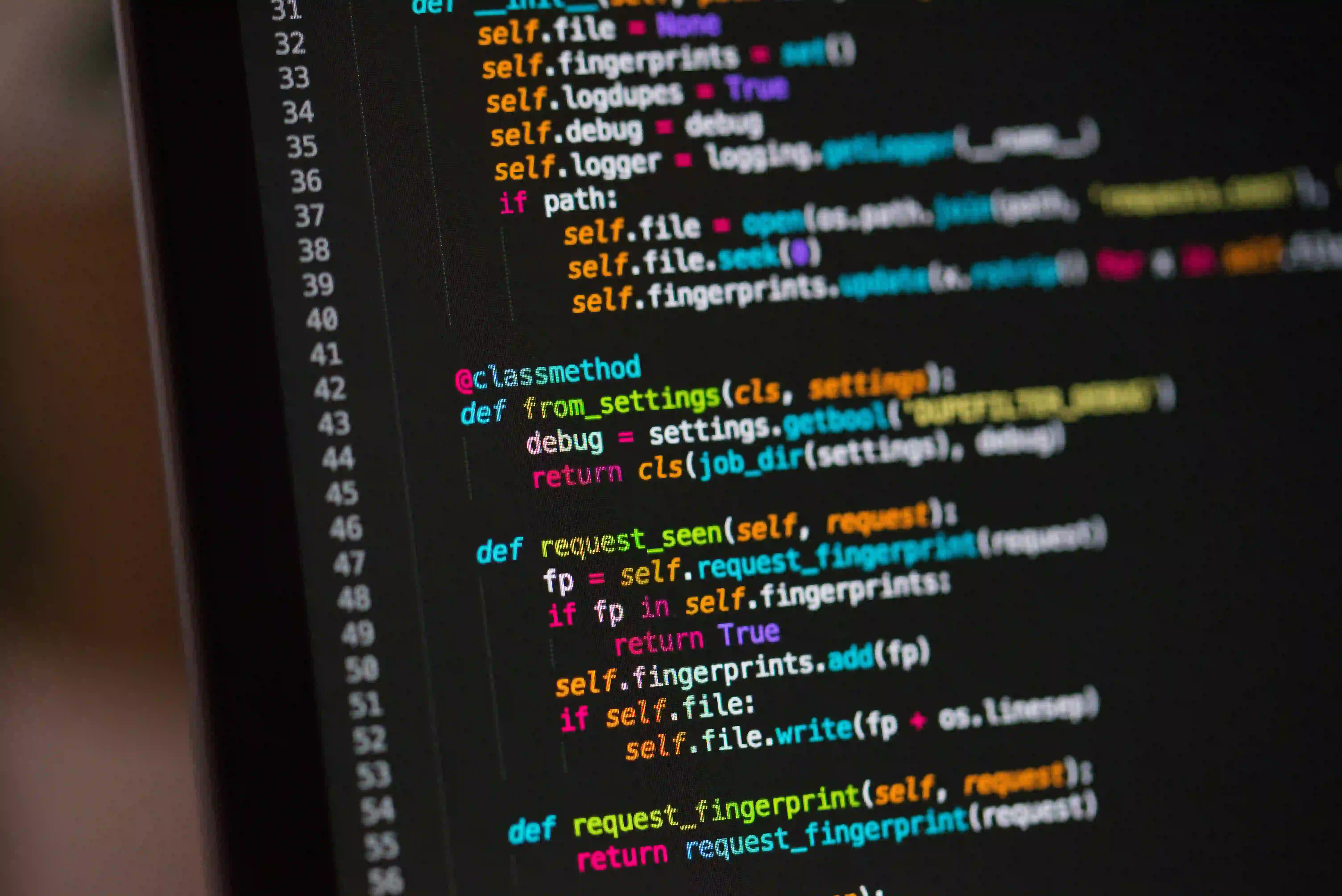
Avoiding Common Pitfalls with Java 8's Filter Method
In Java 8, the introduction of functional programming features brought about significant changes to the way developers handle collections. One of the most commonly used methods is filter()
, which allows developers to extract elements from a collection based on a specified condition. Although it seems straightforward, there are several common pitfalls that developers may encounter when using the filter()
method. In this article, we will delve into these pitfalls and discuss how to avoid them.
Understanding the Filter Method
The filter()
method is a part of the Stream API introduced in Java 8. It is used to filter elements based on a specified condition and return a new stream containing the filtered elements.
Here is a basic syntax of the filter()
method:
Stream<T> filter(Predicate<? super T> predicate)
The filter()
method takes a Predicate
as an argument, which is a functional interface used to define a condition for filtering elements. When the condition defined by the Predicate
is true
for an element, it is included in the resulting stream; otherwise, it is excluded.
Common Pitfalls with Filter Method
1. Not Handling the Returned Stream
One of the common mistakes when using the filter()
method is not handling the returned stream properly. Since filter()
returns a new stream, developers must ensure that the resulting stream is consumed or processed further. Failing to do so can lead to a scenario where the filter operation is never executed due to the laziness of streams.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Incorrect usage of filter - filter is not executed
numbers.stream().filter(n -> n > 3);
// Correct usage - collecting the filtered elements
List<Integer> filteredNumbers = numbers.stream()
.filter(n -> n > 3)
.collect(Collectors.toList());
2. Mutating the State
When using the filter()
method, it is crucial to remember that it does not modify the original collection. Instead, it creates a new stream with the filtered elements. If a developer unintentionally assumes that the original collection is mutated, it can lead to unexpected behavior and bugs in the code.
3. Overusing Complex Conditions
Developers often fall into the trap of using overly complex conditions within the filter()
method. While it is powerful to use lambdas and method references within the filter()
method, writing complex conditions can make the code less readable and harder to maintain.
4. Performance Considerations
In some cases, developers may overlook the performance implications of using the filter()
method. It is essential to understand that the filter()
method iterates through all elements in the stream and applies the specified condition. If the underlying collection is large or the condition is computationally expensive, it can impact the performance of the application.
Best Practices to Avoid Pitfalls
1. Ensure Stream Termination
To avoid the pitfall of not handling the returned stream, always terminate the stream by using terminal operations like collect()
, forEach()
, or reduce()
after applying the filter()
method.
2. Immutable Data Structures
To prevent unintentional mutation of the original collection, consider using immutable data structures from libraries like Google's Guava or Apache Commons Collections. These libraries provide immutable collection types that guarantee the original collection remains unchanged.
3. Encapsulate Complex Conditions
When dealing with complex filtering conditions, consider encapsulating the logic into separate methods or predicates. This approach improves code readability and makes the conditions reusable in different contexts.
4. Use Short-Circuiting Operations
Java 8 streams offer short-circuiting operations like findFirst()
and findAny()
, which can be combined with the filter()
method to improve performance, especially when dealing with large streams. These operations stop processing as soon as the desired element is found, potentially avoiding unnecessary iterations.
Closing Remarks
The filter()
method in Java 8 is a powerful tool for working with collections, but it comes with its own set of potential pitfalls. By understanding these common pitfalls and following best practices, developers can leverage the filter()
method effectively while writing clean, maintainable, and performant code.
Avoiding common pitfalls with Java 8's filter()
method is crucial for writing efficient and bug-free code. By understanding the common mistakes and best practices, developers can harness the power of functional programming features in Java 8 while minimizing the risk of errors and performance issues. Happy coding!
Remember to always test your code and use the aforementioned best practices to ensure that your implementations are error-free and optimized performance-wise.
References: