Enhancing Clean Code Practices with Aspect-Oriented Programming
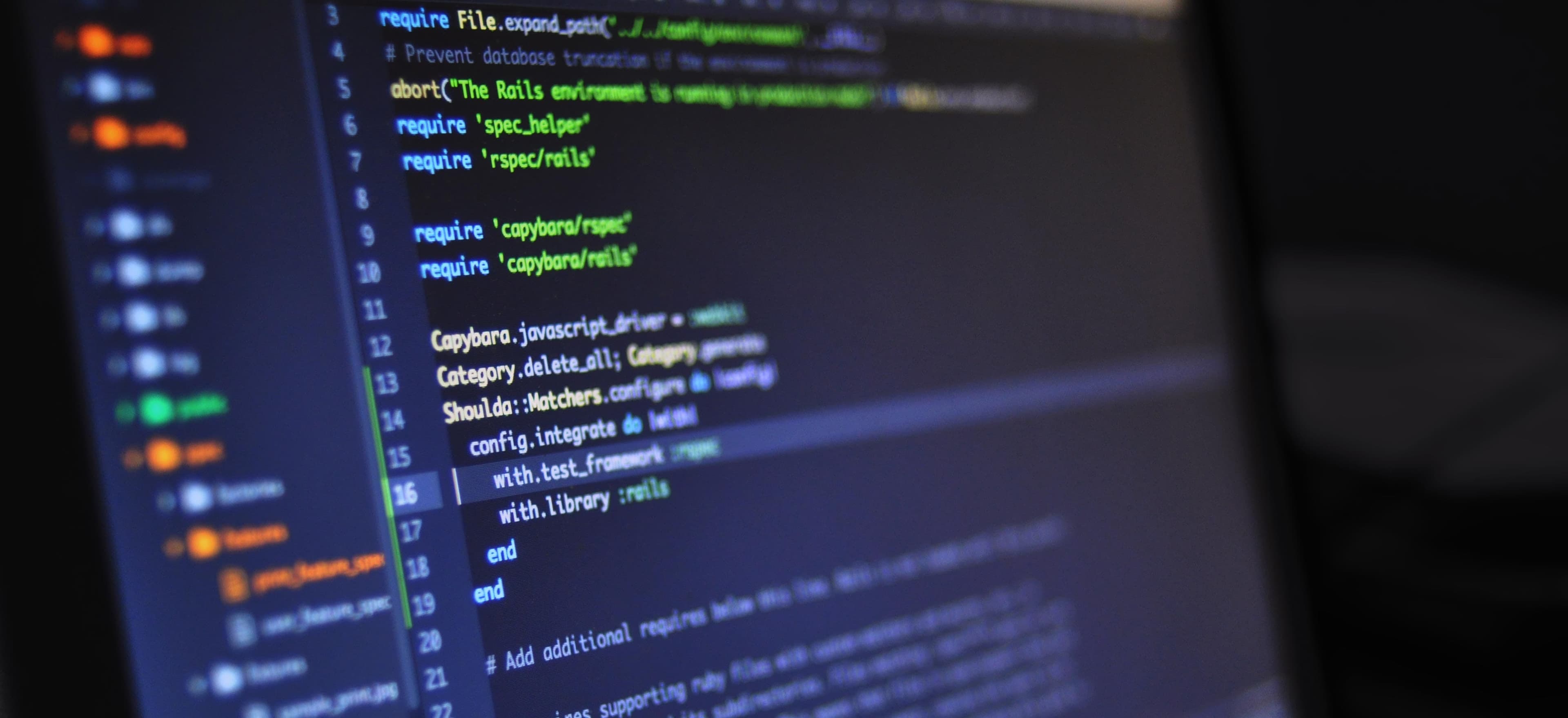
- Published on
Enhancing Clean Code Practices with Aspect-Oriented Programming
In the world of Java programming, maintaining clean, readable, and maintainable code is of paramount importance. However, as applications grow in complexity, adhering to clean code practices becomes increasingly challenging. This is where Aspect-Oriented Programming (AOP) comes into play. AOP provides a powerful mechanism to modularize crosscutting concerns, making it a valuable tool for enhancing clean code practices in Java development.
In this blog post, we will explore how Aspect-Oriented Programming can be used to improve code maintainability, reduce code duplication, and enhance the overall cleanliness of Java applications.
What is Aspect-Oriented Programming?
Aspect-Oriented Programming is a programming paradigm that aims to increase modularity by allowing the separation of crosscutting concerns. In traditional object-oriented programming, crosscutting concerns such as logging, security, and transaction management are often spread across different modules, leading to code duplication and reduced maintainability.
AOP addresses this issue by providing a way to modularize these crosscutting concerns into aspects. An aspect encapsulates behaviors that affect multiple classes and modules, allowing for a more cohesive and centralized approach to managing crosscutting concerns.
Improving Code Maintainability with AOP
One of the key benefits of using AOP is improved code maintainability. By extracting crosscutting concerns into aspects, developers can avoid scattering the same code throughout the codebase, making it easier to understand and maintain.
Let's consider the example of logging. In a traditional approach, logging statements are scattered throughout the codebase, leading to code duplication and making it challenging to update the logging behavior consistently. By using AOP, logging can be abstracted into an aspect, allowing for a centralized and consistent logging mechanism that can be applied across the entire application.
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void beforeMethodExecution(JoinPoint joinPoint) {
String methodName = joinPoint.getSignature().getName();
String className = joinPoint.getTarget().getClass().getName();
System.out.println("Executing method " + methodName + " in class " + className);
}
}
In this example, the LoggingAspect
class defines a crosscutting concern related to logging. The @Before
advice intercepts the execution of methods in the com.example.service
package and logs information about the method execution. By using AOP, the logging behavior is decoupled from the business logic, leading to cleaner and more maintainable code.
Reducing Code Duplication with AOP
Another advantage of AOP is the reduction of code duplication. Crosscutting concerns often result in duplicated code across different modules, leading to maintenance issues and increasing the risk of errors. AOP allows developers to encapsulate these crosscutting concerns into reusable aspects, eliminating the need to duplicate the same code in multiple places.
For instance, consider the scenario of adding transaction management to multiple methods across different classes. Instead of duplicating transaction management code in each method, AOP enables developers to define a single transaction aspect that can be applied to multiple methods, promoting code reuse and reducing duplication.
@Aspect
public class TransactionAspect {
@Around("execution(* com.example.service.*.*(..))")
public Object manageTransaction(ProceedingJoinPoint joinPoint) throws Throwable {
// Begin transaction
try {
Object result = joinPoint.proceed();
// Commit transaction
return result;
} catch (Exception e) {
// Rollback transaction
throw e;
}
}
}
In this example, the TransactionAspect
class defines a crosscutting concern related to transaction management. The @Around
advice intercepts the execution of methods in the com.example.service
package, allowing developers to encapsulate transaction management logic in a reusable aspect.
Enhancing Clean Code Practices with AOP Best Practices
While AOP provides powerful capabilities for enhancing clean code practices, it is essential to follow best practices to ensure effective and maintainable use of AOP in Java development.
Use AOP Where Appropriate
It's important to use AOP judiciously and apply it where it provides clear benefits. Not all crosscutting concerns are suitable for AOP, and overusing AOP can lead to overly complex and difficult-to-maintain code.
Keep Aspects Well-Defined and Focused
When defining aspects, it's crucial to keep them well-defined and focused on specific crosscutting concerns. Avoid creating overly broad and complex aspects, as they can lead to confusion and reduce the maintainability of the codebase.
Provide Clear Documentation
Aspects should be well-documented to provide clear guidance on their purpose, usage, and impact on the codebase. Clear documentation helps developers understand how aspects are used and ensures consistent application of AOP across the project.
Unit Test Aspects
Just like regular code, aspects should be unit tested to verify their behavior and ensure they function as intended. Unit testing aspects helps catch potential issues early and promotes the reliability of AOP-based solutions.
Consider AspectJ for Advanced AOP Features
While Spring AOP provides a lightweight and easy-to-use AOP framework, consider leveraging AspectJ for advanced AOP features such as pointcut expressions, weaving at compile-time, and load-time weaving when more advanced AOP capabilities are required.
A Final Look
In conclusion, Aspect-Oriented Programming provides a powerful approach to enhancing clean code practices in Java development. By modularizing crosscutting concerns into aspects, AOP promotes code maintainability, reduces code duplication, and improves the overall cleanliness of Java applications.
As developers continue to tackle increasingly complex software development challenges, AOP offers a valuable tool for managing crosscutting concerns and maintaining clean, readable, and maintainable code.
By understanding and effectively applying AOP best practices, developers can leverage the full potential of Aspect-Oriented Programming to enhance clean code practices and elevate the quality of Java applications.
Integrating AOP into Java development not only streamlines the codebase but also improves the maintainability of applications, ultimately leading to a more efficient and effective development process.
For further learning, consider exploring Spring AOP and AspectJ for more in-depth understanding of Aspect-Oriented Programming in the context of Java development.