Boost App Engagement: Streamline Emails with Mailgun API
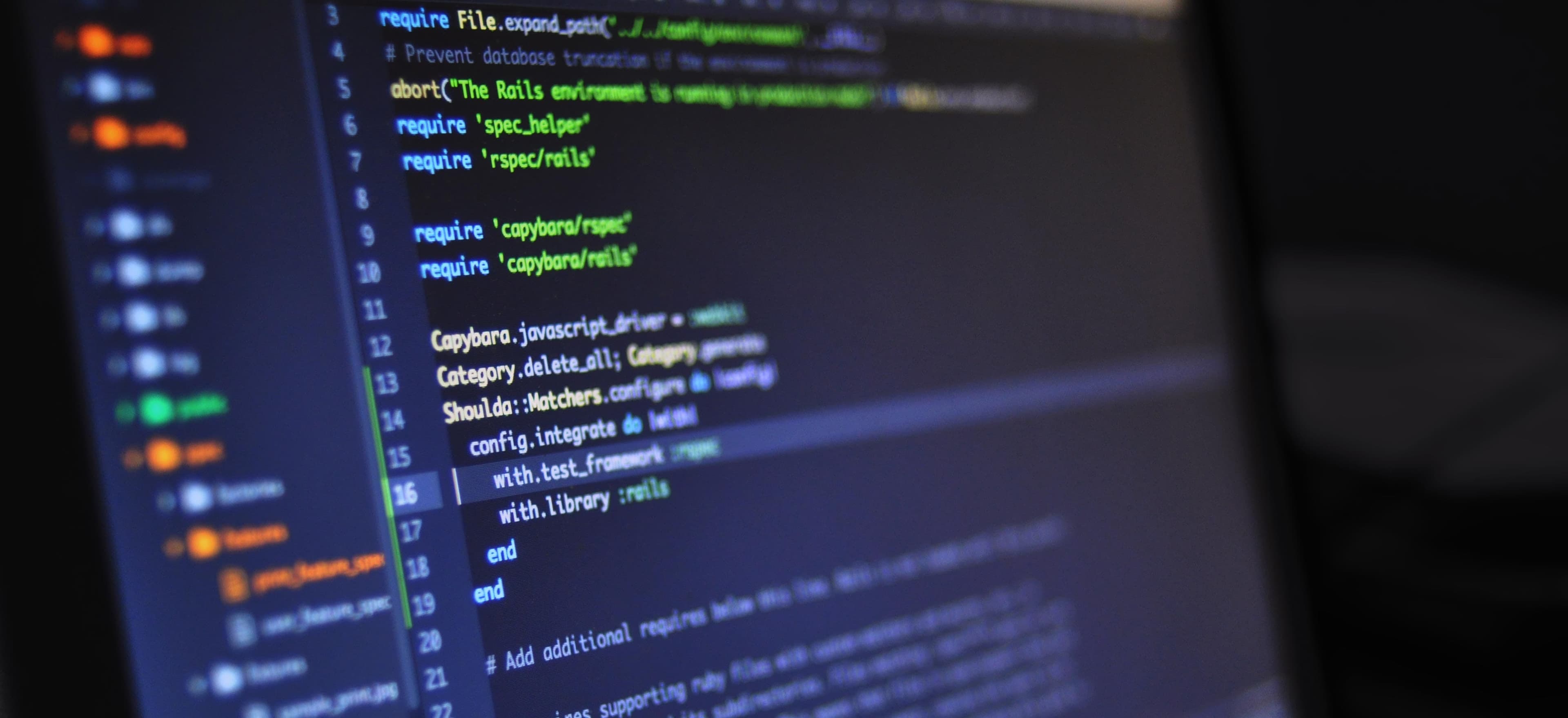
- Published on
Streamlining Email Handling in Java with Mailgun API
Email communication is an essential aspect of any application, be it for user onboarding, notifications, or marketing campaigns. However, as your application grows, managing and optimizing email delivery can become complex. Java, being a widely used language for building robust applications, offers several powerful tools and APIs to streamline email handling. In this article, we will explore how to integrate the Mailgun API with a Java application to simplify and enhance email delivery.
Why Mailgun API?
Mailgun is a popular email service provider that offers a robust set of APIs for sending, receiving, and tracking emails. By leveraging the Mailgun API, developers can ensure reliable email delivery, handle bounce notifications, track email opens and clicks, and more. This makes Mailgun a compelling choice for streamlining email communication within your Java application.
Setting Up Mailgun Account
Before diving into the code, you need to sign up for a Mailgun account if you haven't already. Upon signing up, you will obtain your API credentials, including the API key and the domain associated with your Mailgun account. These credentials will be used to authenticate and authorize your API requests.
Integrating Mailgun API in Java
Step 1: Adding the Mailgun Dependency
To begin with, you need to add the Mailgun Java client library to your project. Using a build automation tool such as Maven, you can simply include the Mailgun dependency in your pom.xml
file:
<dependency>
<groupId>net.sargue</groupId>
<artifactId>mailgun</artifactId>
<version>4.0.0</version>
</dependency>
After adding the dependency, make sure to update your project to fetch the new dependency and refresh the project environment.
Step 2: Sending an Email
Let's dive into the practical integration of the Mailgun API by demonstrating how to send an email through the Mailgun service.
First, you need to initialize the Mailgun client with your API key and domain:
import net.sargue.mailgun.*;
public class MailgunExample {
public static void main(String[] args) {
MailConfiguration config = new MailConfiguration()
.domain("your-mailgun-domain.com")
.apiKey("your-mailgun-api-key");
MailBuilder.using(config)
.to("recipient@example.com")
.from("sender@example.com")
.subject("Hello from Mailgun")
.text("This is a test email sent using Mailgun API.")
.build()
.send();
}
}
In the above code, we initialize the Mailgun client with our domain and API key, then construct an email message specifying the recipient, sender, subject, and content. Finally, we send the email using the send()
method.
Step 3: Handling Email Events
Mailgun provides powerful event tracking features, allowing you to monitor and handle various email events such as opens, clicks, bounces, and more. Let's see how to retrieve and process these events using the Mailgun Java client library.
public class MailgunEventHandling {
public static void main(String[] args) {
MailgunClient client = new MailgunClient("your-mailgun-domain.com", "your-mailgun-api-key");
EventResponse response = client.fetchEvents()
.limit(10) // Limit the number of events
.get();
for (MailEvent event : response.getItems()) {
System.out.println("Event type: " + event.getEvent());
System.out.println("Recipient: " + event.getMessage().getRecipients());
// Process other event attributes as per your requirement
}
}
}
In the above code, we initialize the Mailgun client and fetch the latest email events, such as opens, clicks, and bounces. You can then process these events based on your application's needs.
Bringing It All Together
Integrating the Mailgun API with your Java application provides a seamless and feature-rich solution for managing your email communication. By following the steps outlined in this article, you can simplify email handling, ensure reliable delivery, and leverage powerful event tracking capabilities. As you continue to enhance your application, leveraging reliable and scalable email services like Mailgun becomes increasingly crucial for maintaining user engagement and delivering a seamless user experience.
Remember, efficient email handling is vital for enhancing app engagement. With Mailgun API and Java integration, you can streamline your application's email communication and drive user engagement to new heights.
Start integrating Mailgun API into your Java application today to unlock the full potential of streamlined and efficient email communication. Happy coding!
By seamlessly integrating the Mailgun API with your Java application, you can optimize email processes and drive user engagement. Experience the power of simplified, reliable email communication.
Checkout our other articles