Overcome Hurdles in Proxy Creation with DJCProxy
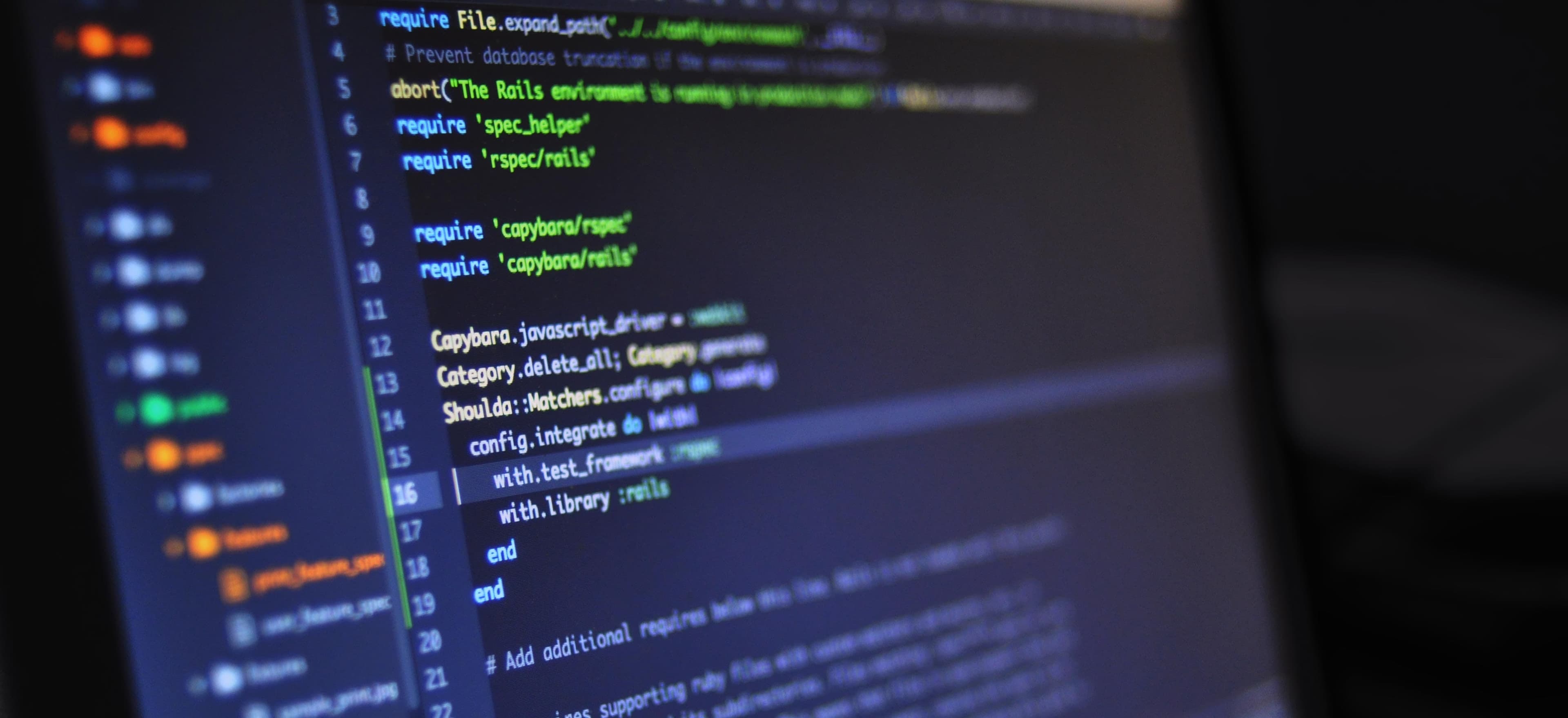
- Published on
Overcome Hurdles in Proxy Creation with DJCProxy
As a Java developer, working with proxies is an essential part of building robust and scalable applications. Proxies serve as intermediaries between clients and servers, providing control, security, and performance benefits. However, creating and managing proxies can be challenging, especially when dealing with complex networking scenarios. In this article, we'll explore how DJCProxy can help you overcome these hurdles and simplify the process of proxy creation in Java.
Understanding the Challenges of Proxy Creation
Before delving into the solution provided by DJCProxy, let's briefly discuss the challenges associated with proxy creation in Java.
1. Tedious Socket Programming
Traditionally, creating a proxy in Java involves low-level socket programming, which can be time-consuming and error-prone. Managing socket connections, handling data transmission, and dealing with network protocols adds complexity to the development process.
2. Security Concerns
Implementing secure communication channels between clients and servers is crucial in today's digital landscape. Ensuring data confidentiality, integrity, and authenticity adds another layer of complexity to proxy implementation.
3. Scalability and Performance
As your application grows, the ability to scale your proxy infrastructure becomes vital. Balancing load, optimizing throughput, and managing connection pools are essential considerations for maintaining performance and scalability.
Introducing DJCProxy
DJCProxy, short for Dynamic Java Compiler Proxy, is a powerful library that simplifies the creation and management of proxies in Java. It offers a high-level API that abstracts away the complexities of low-level networking and provides features for secure communication, scalability, and performance optimization.
Simplifying Proxy Creation
With DJCProxy, creating a proxy in Java becomes a streamlined process. Developers can focus on defining the proxy behavior and let DJCProxy handle the underlying networking details. Let's take a look at a simple example of creating a basic HTTP proxy using DJCProxy.
// Create a basic HTTP proxy server using DJCProxy
public class BasicHttpProxyExample {
public static void main(String[] args) {
DJProxyServer server = new DJProxyServer();
server.setPort(8080);
server.setRequestInterceptor((clientAddress, request) -> {
// Manipulate the incoming request if needed
return request;
});
server.setResponseInterceptor((clientAddress, response) -> {
// Manipulate the outgoing response if needed
return response;
});
server.start();
}
}
In the above example, DJCProxy's DJProxyServer
abstracts the low-level networking details, allowing developers to focus on customizing the proxy behavior using request and response interceptors.
Why DJCProxy?
DJCProxy abstracts away the complexities of low-level networking and provides a clean, high-level API for proxy creation. By leveraging DJCProxy, developers can save time and effort that would have been spent on tedious socket programming.
Security Features
Security is a top priority when it comes to proxy implementation. DJCProxy offers built-in support for SSL/TLS, allowing developers to enable secure communication channels with ease. By integrating SSL/TLS support into your proxies, you can ensure data confidentiality and integrity.
Scalability and Performance Optimization
DJCProxy provides features for managing connection pools, load balancing, and performance tuning out of the box. This allows developers to build proxies that can efficiently handle a large number of concurrent connections while maintaining optimal performance.
Getting Started with DJCProxy
Now that we've covered the benefits of using DJCProxy for proxy creation, let's explore how you can get started with integrating DJCProxy into your Java projects.
Adding DJCProxy to Your Project
To include DJCProxy in your Java project, you can add the following Maven dependency to your pom.xml
file:
<dependency>
<groupId>com.djcproxy</groupId>
<artifactId>djcproxy</artifactId>
<version>1.0.0</version>
</dependency>
Alternatively, if you're using Gradle, you can add the following dependency to your build.gradle
file:
implementation 'com.djcproxy:djcproxy:1.0.0'
Exploring the Documentation
To gain a deeper understanding of DJCProxy's features and API, it's recommended to explore the official documentation provided by the library. The documentation offers detailed examples, explanations of core concepts, and best practices for utilizing DJCProxy effectively.
Building Custom Proxies
Once you're familiar with DJCProxy, you can begin building custom proxies tailored to the specific requirements of your applications. Whether it's a simple HTTP proxy, a WebSocket proxy, or a custom protocol proxy, DJCProxy provides the tools and abstractions you need to succeed.
Key Takeaways
By leveraging DJCProxy, Java developers can overcome the hurdles associated with proxy creation and focus on building robust, secure, and scalable proxy solutions. With its intuitive API, built-in security features, and performance optimization capabilities, DJCProxy simplifies the process of proxy implementation and empowers developers to create high-quality proxy infrastructure.
Incorporating DJCProxy into your projects not only saves time and effort but also ensures that your proxies adhere to best practices and industry standards. So why struggle with low-level socket programming when DJCProxy can elevate your proxy game to the next level?
Give DJCProxy a try and experience the ease and efficiency of proxy creation in Java.
Ready to explore more about proxy creation and DJCProxy? Check out this guide on building robust proxies with Java and dive deeper into the world of proxy servers in Java.
Checkout our other articles