Starting Android Dev? Dodge These Common Beginner Pitfalls
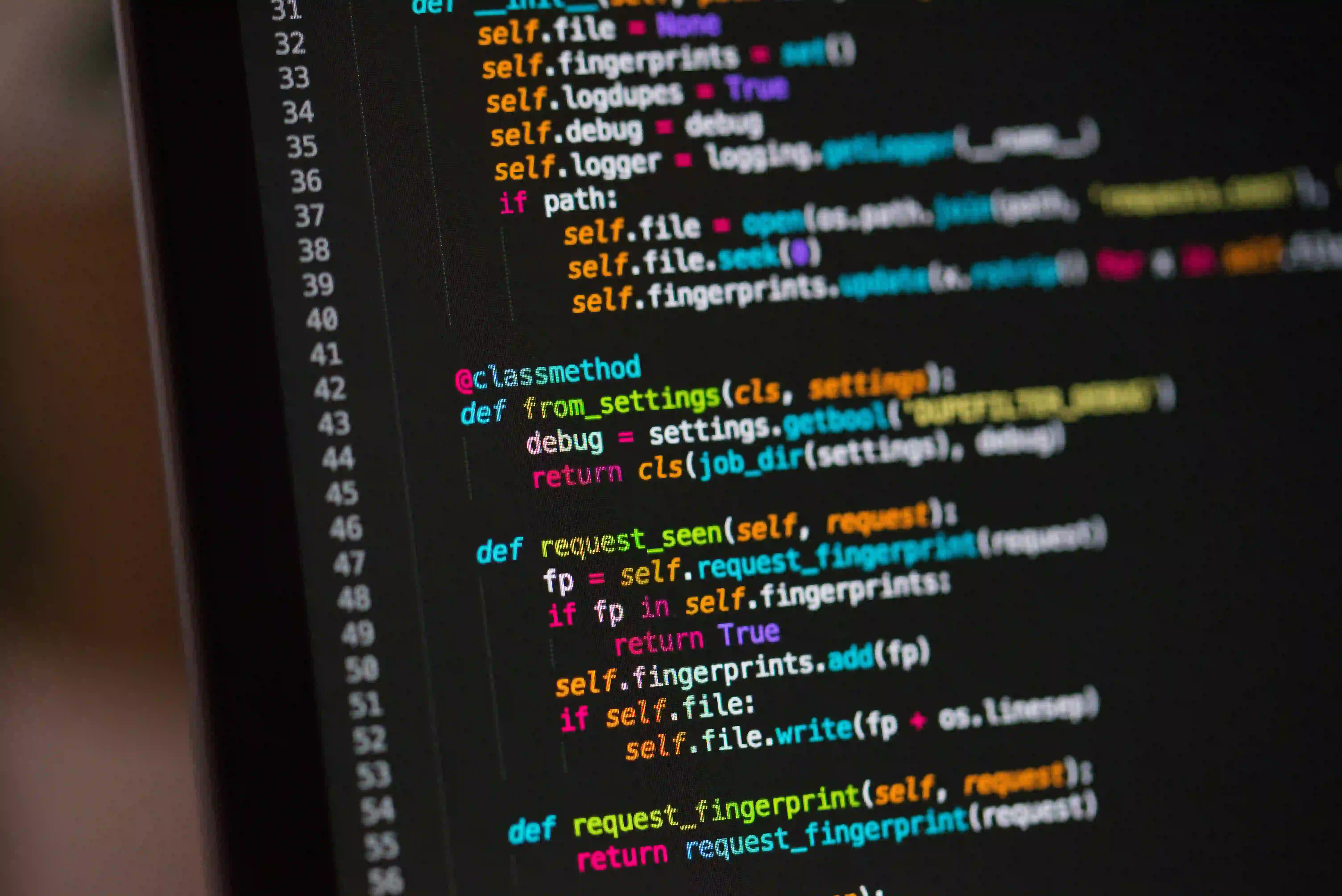
The Beginner's Guide to Avoiding Common Pitfalls in Java Programming
If you're new to Java programming, you're probably eager to dive into the world of Android development. Before you begin, it's crucial to acquaint yourself with some common pitfalls that await beginners. This guide will help you navigate through these hurdles, allowing you to code with confidence and proficiency.
Understanding Java Basics
Java is a powerful and versatile programming language, but it can be intimidating for beginners. Before diving into Android development, it's crucial to have a solid understanding of Java basics. Familiarize yourself with concepts such as variables, data types, loops, and conditional statements. These form the foundation of Java programming and are essential for Android development.
// Example of declaring a variable in Java
int number = 10;
String name = "John Doe";
Understanding the 'why' behind these basics will provide you with a strong groundwork as you progress in your coding journey.
Embracing Object-Oriented Programming (OOP) Principles
Java is an object-oriented language, and a firm grasp of OOP principles is vital for Android development. Understand the four pillars of OOP: encapsulation, inheritance, polymorphism, and abstraction. These principles enable you to create efficient, modular, and scalable code.
// Example of inheritance in Java
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Dog is barking");
}
}
Comprehending OOP principles will allow you to design robust and extensible Android applications.
Handling Exceptions Gracefully
Exception handling is a critical aspect of Java programming. Learn about checked and unchecked exceptions, and understand how to use try-catch blocks effectively. Handling exceptions gracefully will enhance the reliability and stability of your Android applications.
// Example of handling an exception in Java
try {
// Code that may throw an exception
} catch (Exception e) {
// Handle the exception
}
By understanding the 'why' behind exception handling, you'll be better equipped to write code that anticipates and handles errors effectively.
Grasping the Importance of Memory Management
In Java, memory management is handled by the JVM through automatic garbage collection. While Java simplifies memory management, it's crucial to understand memory allocation, object references, and how the garbage collector operates. This knowledge is essential for building efficient Android applications with minimal memory leaks.
Utilizing Design Patterns
Familiarize yourself with common design patterns such as Singleton, Observer, and Builder. Design patterns provide proven solutions to recurring design problems, allowing for efficient, modular, and reusable code. Understanding and implementing these patterns will elevate the quality of your Android applications.
Leveraging Libraries and Frameworks
Java offers a plethora of libraries and frameworks that streamline Android development. Familiarize yourself with popular libraries such as Retrofit for networking, Gson for JSON parsing, and Picasso for image loading. Additionally, understanding the Android framework and its components is crucial for building native Android applications efficiently.
Embracing Clean Code Principles
Writing clean, maintainable code is essential for long-term success in Java programming. Embrace principles such as SOLID, DRY (Don't Repeat Yourself), and KISS (Keep It Simple, Stupid). Writing clean code not only enhances readability but also facilitates easier troubleshooting and maintenance.
Practicing Test-Driven Development (TDD)
Consider adopting test-driven development (TDD) as part of your coding practices. Writing tests before implementing functionality can improve code quality and ensure the robustness of your Android applications. Tools like JUnit make it easy to create and run unit tests in Java.
Continuous Learning and Practice
Java, like any programming language, requires continuous learning and practice. Stay updated with the latest developments, explore advanced topics such as multithreading, concurrency, and performance optimization. Additionally, engage in hands-on projects to reinforce your understanding of Java and Android development.
To Wrap Things Up
By understanding these common pitfalls and proactively addressing them, you'll be better prepared to embark on your Android development journey with confidence. Remember, learning Java and Android development is a rewarding experience, and by navigating these initial challenges, you'll build a strong foundation for your future projects.
Let me know for more!