Overcoming Dependency Injection Issues in Open Liberty
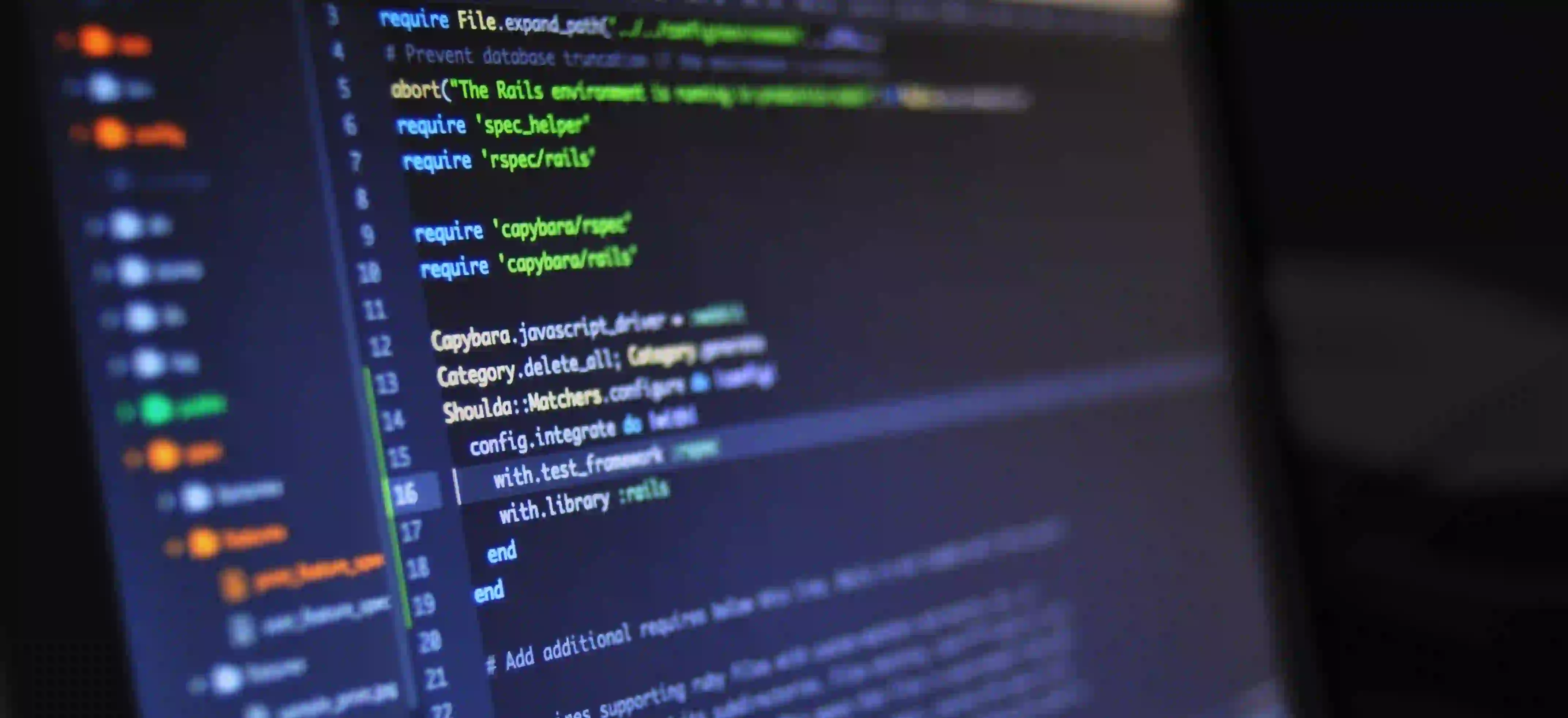
Overcoming Dependency Injection Issues in Open Liberty
In modern Java application development, careful management of dependencies is crucial for building scalable, maintainable, and testable applications. Open Liberty, a lightweight Java application server, provides a robust framework for implementing Dependency Injection (DI) through the Contexts and Dependency Injection (CDI) specification. However, like any technology, developers face challenges while integrating DI in their applications. In this blog post, we'll explore common dependency injection issues in Open Liberty and how to effectively overcome them.
What is Dependency Injection?
Dependency Injection is a design pattern that promotes loose coupling between components by injecting dependencies rather than having components create their dependencies directly. This allows for easier unit testing, maintenance, and the overall flexibility of the application. Open Liberty fully embraces the CDI specification, enabling developers to use annotations to manage dependencies seamlessly.
Why Use Open Liberty for Dependency Injection?
- Lightweight and Fast: Open Liberty is designed to be lightweight, allowing developers to build and deploy applications rapidly.
- Microservices Ready: It supports microservices architecture, allowing for more resilient systems with easier dependency management.
- Hot Deployments: Open Liberty supports hot deployments, meaning you can change code without restarting the application.
Now, let's take a closer look at some common dependency injection issues in Open Liberty and how to address them.
Common Dependency Injection Issues
1. Bean Scope Issues
Problem Statement
When working with CDI, one common issue is mismanaging the scope of beans. There are several bean scopes available, such as @ApplicationScoped
, @SessionScoped
, and @RequestScoped
. Choosing the wrong scope can lead to unexpected behavior, including memory leaks or unintended shared states among requests.
Solution
Make an informed decision about the appropriate scope for each bean. For instance:
import javax.enterprise.context.ApplicationScoped;
@ApplicationScoped
public class ApplicationService {
// Application-level services
}
Using @ApplicationScoped
ensures that the service is instantiated once and shared across all users, making it suitable for resource-heavy beans like database connections.
2. Circular Dependencies
Problem Statement
Circular dependencies occur when two or more beans depend on one another for their instantiation. This creates a situation where CDI cannot resolve the dependencies, leading to deployment failures.
Solution
Refactor your code to minimize or eliminate circular dependencies. One way to do this is by introducing an intermediary interface or utility class. For example:
import javax.enterprise.context.Dependent;
import javax.inject.Inject;
@Dependent
public class A {
private final B b;
@Inject
public A(B b) {
this.b = b;
}
}
@Dependent
public class B {
// No direct reference to A
}
In the example above, A injects B, but B does not inject A. This helps avoid a deployment issue while ensuring that both classes are still able to work together.
3. Misconfigured Beans
Problem Statement
Sometimes, beans are not properly registered, leading to NoSuchBeanException
at runtime when the container attempts to resolve a dependency.
Solution
Ensure that your beans are correctly annotated and that your project structure adheres to CDI conventions. Verify that your beans are included in the beans.xml
configuration file:
<beans xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/beans_1_1.xsd"
version="1.1" />
4. Qualifiers and Ambiguity
Problem Statement
When multiple implementations of an interface exist, CDI may struggle to determine which implementation to inject. This can lead to ambiguity issues, resulting in ResolutionException
.
Solution
Use custom qualifiers to clarify which bean should be injected. Here’s an example of using qualifiers:
import javax.inject.Qualifier;
import java.lang.annotation.Retention;
import java.lang.annotation.Target;
import static java.lang.annotation.ElementType.*;
import static java.lang.annotation.RetentionPolicy.RUNTIME;
@Qualifier
@Retention(RUNTIME)
@Target({TYPE, METHOD, FIELD, PARAMETER})
public @interface MyCustomQualifier {
}
@MyCustomQualifier
@ApplicationScoped
public class MyServiceImpl implements MyService {
// Implementation
}
@Inject
@MyCustomQualifier
private MyService myService;
Creating a custom qualifier helps specify exactly which implementation should be injected, thus preventing ambiguity complications.
5. Contextual Lifecycle Issues
Problem Statement
Misunderstanding the lifecycle of CDI beans can lead to instances where beans are not available when expected. For instance, you might attempt to inject a request-scoped bean into an application-scoped bean, which is not allowed.
Solution
Keep a clear understanding of bean lifecycles and dependencies. Refer to the CDI lifecycle documentation for better insights.
Additional Resources
To solidify your understanding and further enhance your skills in copying with DI issues, consider visiting the official Open Liberty Documentation as well as the CDI specification, providing in-depth insights into the workings of these technologies.
Lessons Learned
Effectively managing Dependency Injection in Open Liberty requires an understanding of how CDI works. By addressing common issues such as bean scope, circular dependencies, misconfigured beans, qualifiers, and lifecycle management, you can avoid pitfalls and enhance your application's architecture significantly.
Overall, Open Liberty enables developers to create efficient, scalable applications while dealing with dependency management challenges seamlessly. Applying the strategies discussed in this blog will not only help overcome existing problems but will also foster good practices for future development endeavors.
Final Thoughts
The world of Java application development is constantly evolving. Stay curious, keep learning, and ensure to leverage the best practices for Dependency Injection. Happy coding!
By focusing on the above points and best practices, you can streamline your dependency injection process in Open Liberty and build robust Java applications tailored for the modern development landscape.