Breaking Down the Complexity: Laws of Software Design Simplified
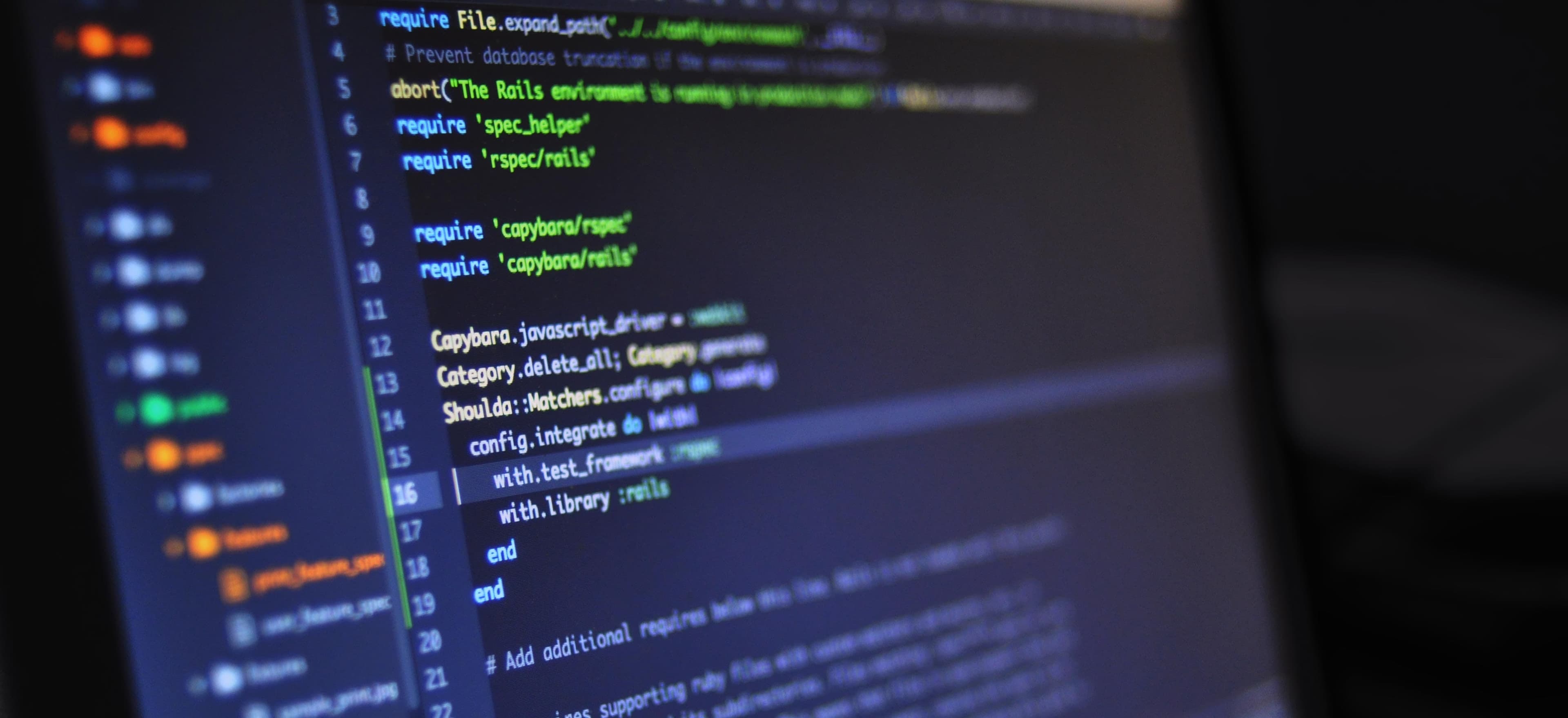
- Published on
Breaking Down the Complexity: Laws of Software Design Simplified
When it comes to creating quality software, adhering to certain principles and practices is essential. The laws of software design provide a framework for developers to follow, helping to ensure that the resulting code is efficient, maintainable, and scalable. In this article, we will explore some of the key laws of software design, breaking down complex concepts into easily digestible insights and exploring their practical application in Java.
The SOLID Principles
Single Responsibility Principle (SRP)
One of the fundamental principles of object-oriented design, the Single Responsibility Principle, states that a class should have only one reason to change. In other words, a class should have only one job.
Example:
public class Employee {
private String name;
private double salary;
public void calculateSalary() {
// Salary calculation logic
}
public void save() {
// Save employee to database
}
}
In this example, the Employee
class handles both salary calculation and persistence to the database, violating the SRP. The class can be refactored into Employee
and EmployeeRepository
to adhere to the SRP.
Open/Closed Principle (OCP)
The Open/Closed Principle suggests that software entities should be open for extension but closed for modification. This means that the behavior of a module can be extended without modifying its source code.
Example:
public interface Shape {
double area();
}
public class Circle implements Shape {
private double radius;
@Override
public double area() {
return Math.PI * radius * radius;
}
}
public class Rectangle implements Shape {
private double width;
private double height;
@Override
public double area() {
return width * height;
}
}
By adhering to the OCP, adding a new shape (e.g., Triangle
) can be achieved without modifying the existing Shape
interface or its implementations.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclass without affecting the functionality of the program.
Example:
public interface Shape {
double area();
}
public class Rectangle implements Shape {
private double width;
private double height;
@Override
public double area() {
return width * height;
}
}
public class Square extends Rectangle {
public void setWidth(double width) {
this.width = width;
this.height = width;
}
public void setHeight(double height) {
this.height = height;
this.width = height;
}
}
In this example, a Square
can replace a Rectangle
without affecting the area calculation logic.
Interface Segregation Principle (ISP)
The Interface Segregation Principle suggests that a client should not be forced to implement an interface that it doesn't use. It promotes smaller, cohesive interfaces.
Example:
// Bad design violating ISP
public interface Worker {
void work();
void eat();
void sleep();
}
// Better design following ISP
public interface Workable {
void work();
}
public interface Eatable {
void eat();
}
public interface Sleepable {
void sleep();
}
By segregating the interfaces, classes can implement only the functionality they require, thus preventing unnecessary dependencies.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules, but rather both should depend on abstractions. It promotes decoupling and easier maintenance.
Example:
public interface MessageProvider {
String getMessage();
}
public class DatabaseMessageProvider implements MessageProvider {
@Override
public String getMessage() {
// Get message from database
}
}
public class Application {
private final MessageProvider messageProvider;
public Application(MessageProvider messageProvider) {
this.messageProvider = messageProvider;
}
public void displayMessage() {
String message = messageProvider.getMessage();
// Display message
}
}
By injecting the MessageProvider
abstraction, the Application
class adheres to the DIP, allowing for easier testing and flexibility in message retrieval mechanisms.
KISS and YAGNI
Keep It Simple, Stupid (KISS)
The KISS principle advocates for simplicity in design and implementation. It suggests that systems work best if they are kept simple rather than made complex.
Example:
public double calculateTotalSalary(List<Employee> employees) {
double totalSalary = 0;
for (Employee employee : employees) {
totalSalary += employee.getSalary();
}
return totalSalary;
}
The calculateTotalSalary
method follows the KISS principle by straightforwardly summing employee salaries without unnecessary complexities.
You Aren't Gonna Need It (YAGNI)
YAGNI advises developers not to add functionality until it is necessary. This helps to avoid over-engineering and the accumulation of unused code.
Example:
public class Product {
private String name;
private double price;
// YAGNI in action - no need for a constructor with additional parameters
public Product(String name, double price) {
this.name = name;
this.price = price;
}
}
By sticking to minimal, required functionality, the codebase remains lean and focused.
DRY and BOD
Don't Repeat Yourself (DRY)
The DRY principle aims to reduce redundancy in code by promoting the reuse of existing code where possible. It enhances maintainability and reduces the chance of inconsistencies.
Example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
By creating reusable methods like add
and subtract
, the DRY principle is upheld, preventing the need to rewrite similar logic in multiple places.
Boy Scout Rule (BOD)
The Boy Scout Rule encourages developers to leave the codebase cleaner than they found it. It advocates for making small improvements whenever possible to maintain a healthy, sustainable codebase.
Example:
// Applying the Boy Scout Rule by improving method naming
public double calculateAreaOfCircle(double radius) {
return Math.PI * radius * radius;
}
By adhering to the BOD, the codebase evolves incrementally, resulting in an overall improvement over time.
The Closing Argument
In the world of software design, these laws and principles serve as guiding lights, steering developers toward writing clean, maintainable, and scalable code. By following these principles, we can craft software that is not only efficient and bug-free but also easier to extend and maintain. From the SOLID principles to KISS, YAGNI, DRY, and BOD, these guidelines offer a roadmap to success in the realm of software design. Embracing and applying these principles in Java development will undoubtedly lead to code that is robust, adaptable, and a joy to work with.
In the next blog post, we will delve into design patterns and their practical implementation in Java, further enhancing our understanding of software design best practices.
By understanding and applying these laws of software design, we can pave the way for a more efficient, maintainable, and scalable codebase. Remember, software development is not just about writing code; it's about crafting elegant and effective solutions. Happy coding!
Remember, in the world of software design, keeping it simple and intuitive is often the best path to follow. By sticking to well-established principles, you can streamline your development process and build more effective solutions.