Solving Update Chaos with Observer Design Pattern
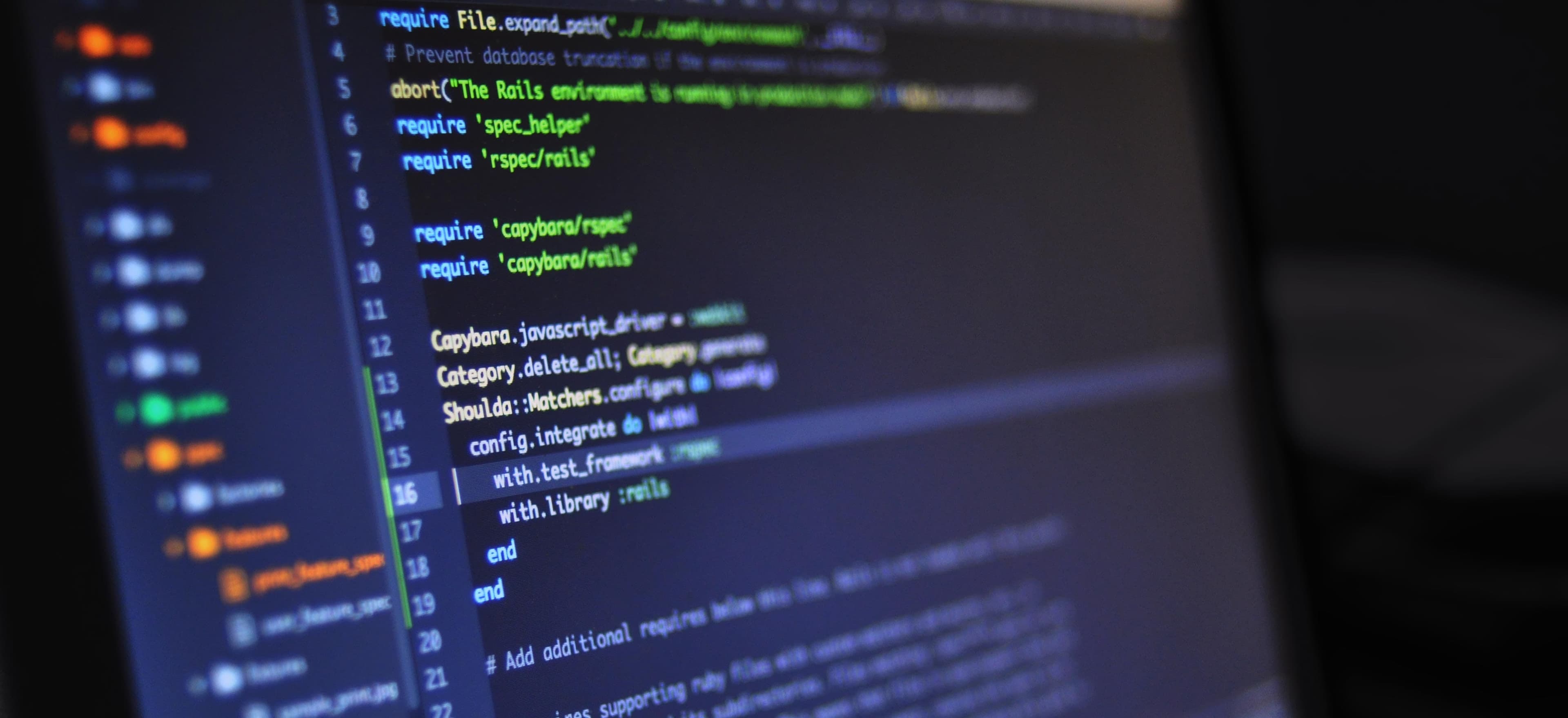
- Published on
Solving Update Chaos with Observer Design Pattern
In the world of software development, maintaining clean, readable, and efficient code is crucial. One common challenge faced by developers is managing dependencies between different components of an application. As a Java developer, you might have encountered scenarios where changes to one part of the codebase result in a cascading series of updates across multiple components, leading to what can only be described as 'update chaos'.
Enter the Observer Design Pattern, a powerful solution to this problem. In this article, we'll explore the challenges posed by update chaos, and how the Observer Design Pattern provides an elegant and efficient way to address them in Java applications.
Understanding the Update Chaos
Imagine a scenario where you are working on a complex Java application with multiple components. When a change occurs in one component, it may require updates in several other dependent components. For instance, consider an e-commerce system where the inventory is updated. This update should reflect in various parts of the system such as order processing, notifications, and reporting. Without a proper mechanism in place, managing these cross-component updates can quickly lead to chaos and spaghetti-like code.
Enter the Observer Design Pattern
The Observer Design Pattern is a behavioral design pattern that defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This not only reduces the coupling between classes but also provides a way to manage the dependencies in a structured manner.
How it works
At the core of the Observer pattern are two key entities: the Subject and the Observer. The Subject is the object that holds some important state and notifies the Observers about any changes. The Observers are the dependent objects that are interested in the state changes of the Subject.
When the Subject's state changes, it iterates through its list of registered Observers and notifies each one of them. This allows the Observers to update themselves accordingly.
Let's dive into some concrete examples in Java to understand the implementation better.
Implementing the Observer Design Pattern in Java
import java.util.ArrayList;
import java.util.List;
// Subject interface
interface Subject {
void registerObserver(Observer observer);
void removeObserver(Observer observer);
void notifyObservers();
}
// Concrete Subject
class InventorySystem implements Subject {
private List<Observer> observers = new ArrayList<>();
private int availableQuantity;
public void setAvailableQuantity(int availableQuantity) {
this.availableQuantity = availableQuantity;
notifyObservers();
}
@Override
public void registerObserver(Observer observer) {
observers.add(observer);
}
@Override
public void removeObserver(Observer observer) {
observers.remove(observer);
}
@Override
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(availableQuantity);
}
}
}
// Observer interface
interface Observer {
void update(int availableQuantity);
}
// Concrete Observer
class OrderProcessor implements Observer {
@Override
public void update(int availableQuantity) {
// Update order processing logic based on the new available quantity
}
}
In the above example, we have an InventorySystem
acting as the Subject and an OrderProcessor
acting as the Observer. The InventorySystem
maintains a list of Observers and notifies them when the availableQuantity
changes. This allows the OrderProcessor
to update its processing logic based on the new available quantity.
Why is it Effective?
The Observer Design Pattern is effective for several reasons:
-
Loose Coupling: By using the Observer pattern, the Subject and Observers are loosely coupled. The Subject doesn’t need to know the specific details of its Observers, allowing for easier maintenance and updates.
-
Scalability: Adding new Observers becomes straightforward. You can simply implement the Observer interface and register the new Observer with the Subject.
-
Modularity and Reusability: Each Observer can be developed and tested independently, promoting modularity and reusability of code.
-
Event-Driven Architecture: It provides a clean way to implement event-driven architecture, where actions in one part of the system trigger responses in other parts.
Real-World Use Cases
The Observer pattern is widely used in various Java frameworks and libraries. For instance, in Java Swing, the Observer pattern is used for event handling. Swing components such as buttons and text fields act as Subjects, while the event listeners act as Observers.
In the broader context of software development, the Observer pattern is utilized in implementing reactive programming paradigms, such as in libraries like RxJava, where changes in data streams are observed and reacted to by observers.
The Bottom Line
In the realm of Java development, the Observer Design Pattern serves as a robust solution for managing dependencies and mitigating update chaos. By fostering loose coupling, scalability, and modularity, it empowers developers to build maintainable and efficient applications.
When faced with the challenge of coordinating changes across multiple components, the Observer pattern stands as a stalwart ally, providing a clear and elegant mechanism for handling such scenarios.
Incorporating the Observer pattern in your Java applications can lead to cleaner code, streamlined updates, and a more organized codebase.
So, next time you find yourself in the midst of update chaos, remember the Observer pattern as your tool to bring order to the chaos!